Set Font Size for Label Text in Tkinter Python
In Tkinter, the Label
widget is used to display text or images. To set the font size of a Label, the font
option is used. The font can be specified in the following formats:
- Tuple format:
(font_family, size, style)
(e.g.,("Arial", 14, "bold")
) - String format:
"font_family size style"
(e.g.,"Helvetica 16 italic"
)
In this tutorial, we will go through multiple examples to set font size in a Tkinter Label.
Examples
1. Setting Font Size Using Tuple Format in Tkinter
In this example, we will set the font size using a tuple (font_family, size, style)
.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Font Size Example")
root.geometry("400x200")
# Create a Label with font size 20
label = tk.Label(root, text="Hello, Tkinter!", font=("Arial", 20))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with text “Hello, Tkinter!” appears with font size 20.
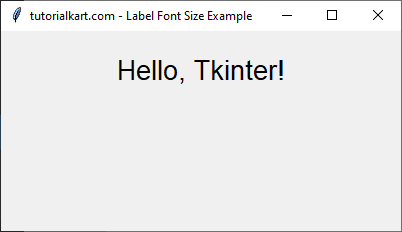
2. Setting Font Size Using String Format in Tkinter
In this example, we will set the font size using a string "font_family size style"
.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Label Font Size Example")
root.geometry("400x200")
# Create a Label with font size 18 using string format
label = tk.Label(root, text="Welcome to Tkinter!", font="Helvetica 18 bold")
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with text “Welcome to Tkinter!” appears with font size 18 in bold style.
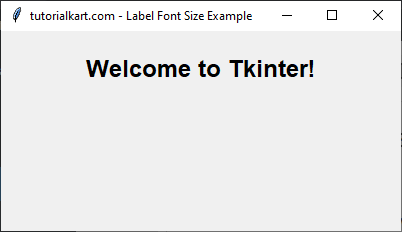
3. Setting Font Size Dynamically in Tkinter
We can change the font size dynamically using the config()
method.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Dynamic Font Size Example")
root.geometry("400x200")
# Function to increase font size
def increase_font():
label.config(font=("Verdana", 24))
# Create a Label with default font size
label = tk.Label(root, text="Click button to enlarge text", font=("Verdana", 16))
label.pack(pady=10)
# Create a Button to increase font size
btn = tk.Button(root, text="Increase Font", command=increase_font)
btn.pack()
root.mainloop()
Output in Windows:
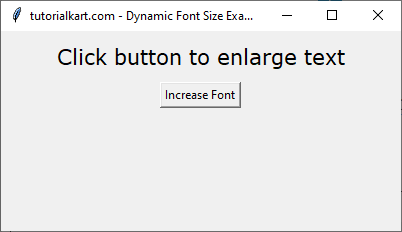
Clicking the button increases the font size of the label dynamically.
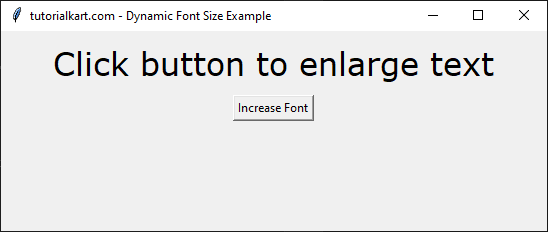
Conclusion
In this tutorial, we explored different ways to set the font size for Label text in Tkinter using the font
option. Font sizes can be set using:
- Tuple format:
("FontFamily", Size, "Style")
- String format:
"FontFamily Size Style"
- Dynamic font resizing using
config()
By adjusting font sizes, you can enhance the readability and appearance of your Tkinter GUI.