Font Size for Text Widget in Tkinter Python
In Tkinter, the Text
widget allows users to input and edit multi-line text. You can customize the font size using the font
option, which accepts a tuple containing the font name and size.
The font size in the Text
widget can be set in two ways:
- Using a tuple:
font=("Arial", 14)
- Using the
tkinter.font
module:font=custom_font
In this tutorial, we will go through examples using different methods to set the font size in a Tkinter Text Widget.
Examples
1. Setting Font Size for Text Widget Using Tuple Notation
In this example, we create a Tkinter window with a Text
widget and set the font size using a tuple.
The Text
widget is assigned the font ("Arial", 16)
, meaning the text will be displayed in Arial with a size of 16. We use root.geometry("400x200")
to define the window size and root.title("Text Widget Font Size - tutorialkart.com")
to set the title.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Text Widget Font Size - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with font size 16
text_widget = tk.Text(root, font=("Arial", 16))
text_widget.pack(pady=10)
# Run the application
root.mainloop()
Output in Windows:
A text box appears in the window with Arial font size 16.
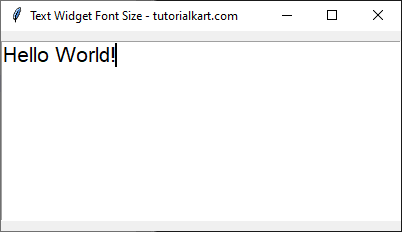
2. Setting Font Size for Text Widget Using tkinter.font Module
In this example, we create a Tkinter window with a Text
widget and set the font size using the tkinter.font
module.
We first import font
from tkinter
and define a custom font using custom_font = tk.font.Font(family="Courier", size=18)
. This sets the font to “Courier” with a size of 18. We then assign this custom font to the Text
widget.
main.py
import tkinter as tk
import tkinter.font as font
# Create the main window
root = tk.Tk()
root.title("Text Widget Font Size - tutorialkart.com")
root.geometry("400x200")
# Define a custom font
custom_font = font.Font(family="Courier", size=18)
# Create a Text widget with custom font
text_widget = tk.Text(root, font=custom_font)
text_widget.pack(pady=10)
# Run the application
root.mainloop()
Output in Windows:
A text box appears in the window with text displayed in Courier font, size 18.
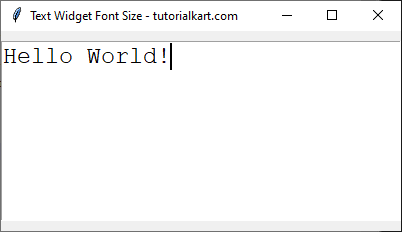
Conclusion
In this tutorial, we explored different ways to set the font size in the Tkinter Text
widget:
- Using a tuple notation:
font=("Arial", 16)
- Using the
tkinter.font
module:font=custom_font