Set Horizontal Scrollbar for Text Widget in Tkinter Python
In Tkinter, the Scrollbar
widget is used to add a scroll bar to other widgets, such as the Text
widget. By default, the Text
widget supports vertical scrolling, but for horizontal scrolling, we need to configure both the Text
widget and the Scrollbar
.
The Scrollbar
must be linked with the Text
widget using the xscrollcommand
property in the Text
widget and the command
property in the Scrollbar
widget.
In this tutorial, we will go through detailed examples demonstrating how to add a horizontal scrollbar to a Tkinter Text Widget.
Examples
1. Adding a Horizontal Scrollbar to a Text Widget
In this example, we will create a Text
widget and add a horizontal scrollbar to allow scrolling through long lines of text.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Horizontal Scrollbar - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, wrap="none", height=5, width=40)
text_widget.pack(side="top", fill="both", expand=True)
# Create a horizontal scrollbar
scroll_x = tk.Scrollbar(root, orient="horizontal", command=text_widget.xview)
scroll_x.pack(side="bottom", fill="x")
# Configure the text widget to use the scrollbar
text_widget.config(xscrollcommand=scroll_x.set)
# Insert long text for testing
text_widget.insert("1.0", "This is a long line of text that extends beyond the default width of the text widget.")
# Run the main loop
root.mainloop()
Output in Windows:
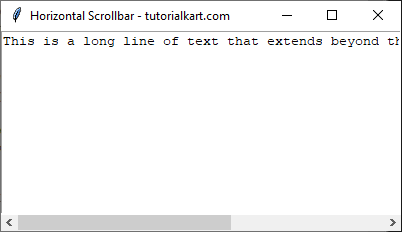
The text widget now allows horizontal scrolling to view the full text.
2. Horizontal and Vertical Scrollbars in Text Widget
In this example, we will create a Text
widget with both horizontal and vertical scrollbars.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Text Widget with Scrollbars - tutorialkart.com")
root.geometry("400x200")
# Create a frame to hold the text widget and scrollbars
frame = tk.Frame(root)
frame.pack(fill="both", expand=True)
# Create a Text widget
text_widget = tk.Text(frame, wrap="none", height=5, width=40)
text_widget.grid(row=0, column=0, sticky="nsew")
# Create a horizontal scrollbar
scroll_x = tk.Scrollbar(frame, orient="horizontal", command=text_widget.xview)
scroll_x.grid(row=1, column=0, sticky="ew")
# Create a vertical scrollbar
scroll_y = tk.Scrollbar(frame, orient="vertical", command=text_widget.yview)
scroll_y.grid(row=0, column=1, sticky="ns")
# Configure the text widget to use both scrollbars
text_widget.config(xscrollcommand=scroll_x.set, yscrollcommand=scroll_y.set)
# Configure grid layout
frame.grid_rowconfigure(0, weight=1)
frame.grid_columnconfigure(0, weight=1)
# Insert long text for testing
text_widget.insert("1.0", "This is a long line of text that extends beyond the default width of the text widget.\n"
"Another line of text to test vertical scrolling.\n"
"More lines...\n"
"Scrolling is enabled both horizontally and vertically.")
# Run the main loop
root.mainloop()
Output in Windows:
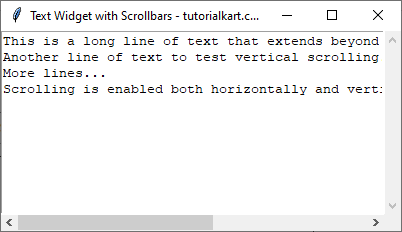
The text widget now allows scrolling both horizontally and vertically.
Conclusion
In this tutorial, we explored how to add a horizontal scrollbar to a Tkinter Text
widget using the Scrollbar
widget. The scrollbar is configured with the xscrollcommand
and allows smooth navigation through long text lines.
Additionally, we demonstrated how to add both a horizontal and vertical scrollbar to enhance text navigation further.