Set Italic Text for Button in Tkinter
In Tkinter, the font
attribute of a Button widget allows you to set the text style, including making the text italic. The font property takes a tuple consisting of:
- Font family (e.g.,
"Arial"
,"Times New Roman"
) - Font size (e.g.,
12
,14
) - Font style (e.g.,
"italic"
)
To set an italic text style for a Tkinter Button, pass ("FontName", Size, "italic")
to the font
attribute.
Examples
1. Setting Italic Text for a Button
In this example, we will create a button with italic text using the font
attribute.
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Italic Text in Tkinter Button")
root.geometry("400x200")
# Create a button with italic text
button = tk.Button(root, text="Click Me", font=("Arial", 14, "italic"))
button.pack(pady=10)
root.mainloop()
Screenshot in Windows
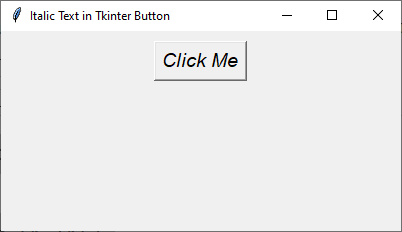
2. Using Different Fonts with Italic Text
We can use different font families along with the italic style.
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Different Italic Fonts in Button")
root.geometry("400x200")
# Button with Times New Roman Italic
btn_times = tk.Button(root, text="Times New Roman", font=("Times New Roman", 16, "italic"))
btn_times.pack(pady=5)
# Button with Courier Italic
btn_courier = tk.Button(root, text="Courier", font=("Courier", 14, "italic"))
btn_courier.pack(pady=5)
root.mainloop()
Screenshot in Windows
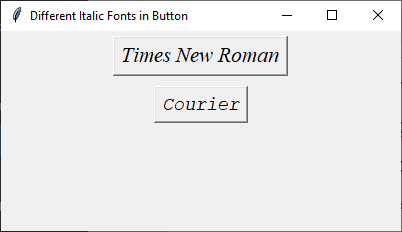
3. Italic Text with Bold Style
We can combine italic with other styles like bold.
</>
Copy
import tkinter as tk
root = tk.Tk()
root.title("Bold and Italic Text in Button")
root.geometry("400x200")
# Create a button with bold and italic text
button = tk.Button(root, text="Bold Italic Text", font=("Verdana", 14, "bold italic"))
button.pack(pady=10)
root.mainloop()
Screenshot in Windows
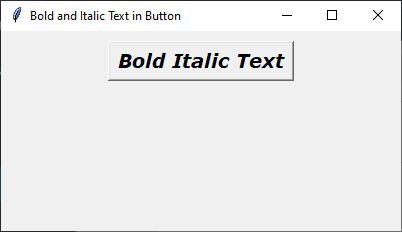
Conclusion
In this tutorial, we explored how to set italic text for a Tkinter Button using the font
attribute. You can customize the font style by:
- Using different font families like
"Arial"
,"Times New Roman"
- Adjusting the font size (e.g.,
14
,16
) - Combining italic with other styles like
"bold"
By styling the text, you can enhance the appearance of buttons in your Tkinter application.