Set Italic Text for Label in Tkinter Python
In Tkinter, the Label
widget is used to display text in a GUI window. You can set the text to be italic by specifying an appropriate font style using the font
option.
To make the text italic in a Tkinter Label
, use the font
parameter with a tuple containing:
- Font family (e.g., “Arial”, “Times New Roman”)
- Font size (e.g., 12, 14, 16, etc.)
- Font style (
"italic"
)
In this tutorial, we will go through examples to set italic text in a Tkinter Label.
Examples
1. Setting Italic Text in Label Using Arial Font
In this example, we will create a Tkinter Label with italic text using the “Arial” font.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Italic Text Example")
root.geometry("400x200")
# Create a label with italic text
label = tk.Label(root, text="This is italic text", font=("Arial", 14, "italic"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with italic text using the Arial font is displayed.
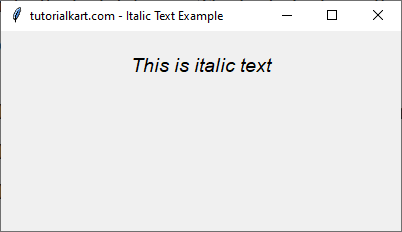
2. Setting Italic Text in Label Using Times New Roman
We will now use the “Times New Roman” font with italic style.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Italic Text Example")
root.geometry("400x200")
# Create a label with italic text using Times New Roman
label = tk.Label(root, text="Italic Text in Times New Roman", font=("Times New Roman", 16, "italic"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with italic text using the “Times New Roman” font is displayed.
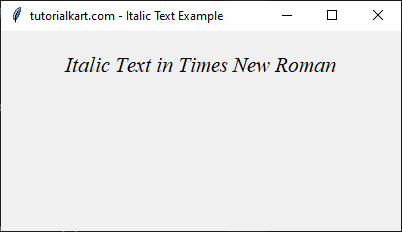
3. Setting Italic Text with Custom Font Size
We will now create a label with a larger font size (20) using the italic style.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Italic Text Example")
root.geometry("400x200")
# Create a label with italic text and large font size
label = tk.Label(root, text="Large Italic Text", font=("Helvetica", 20, "italic"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with large italic text using the “Helvetica” font is displayed.
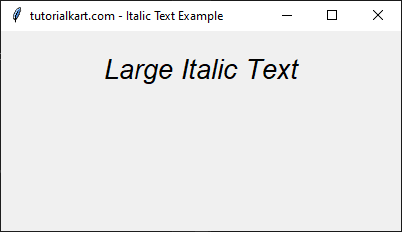
4. Combining Italic with Bold Style
You can also combine italic with bold text in a Label.
import tkinter as tk
root = tk.Tk()
root.title("tutorialkart.com - Italic Bold Text Example")
root.geometry("400x200")
# Create a label with italic and bold text
label = tk.Label(root, text="Bold and Italic Text", font=("Verdana", 18, "bold italic"))
label.pack(pady=20)
root.mainloop()
Output in Windows:
A label with bold and italic text using the “Verdana” font is displayed.
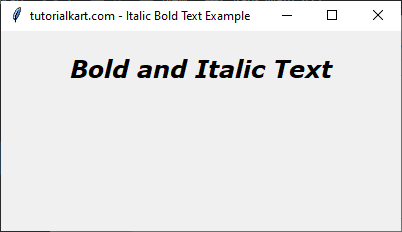
Conclusion
In this tutorial, we explored how to use the font
option in Tkinter’s Label widget to set italic text. By using different font families, sizes, and styles, you can customize text appearance in your GUI applications.