Set Placeholder for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget does not have a built-in placeholder (hint text) feature like modern GUI frameworks. However, you can create a placeholder effect by inserting default text and binding events to clear or restore it when the user interacts with the widget.
To set a placeholder in an Entry
widget, we can use the following approach:
- Insert the placeholder text when the widget is created.
- Bind the
<FocusIn>
event to clear the text when the user clicks inside the entry. - Bind the
<FocusOut>
event to restore the placeholder if the field is empty.
In this tutorial, we will go through multiple examples demonstrating how to implement a placeholder in the Tkinter Entry widget.
Examples
1. Basic Placeholder for Entry Widget
In this example, we create an entry field with a placeholder that disappears when clicked and reappears if left empty.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Placeholder Entry Example - tutorialkart.com")
root.geometry("400x200")
def on_entry_click(event):
if entry.get() == "Enter your name":
entry.delete(0, tk.END)
entry.config(fg="black")
def on_focus_out(event):
if not entry.get():
entry.insert(0, "Enter your name")
entry.config(fg="grey")
entry = tk.Entry(root, fg="grey")
entry.insert(0, "Enter your name")
entry.bind("<FocusIn>", on_entry_click)
entry.bind("<FocusOut>", on_focus_out)
entry.pack(pady=20)
root.mainloop()
Output in Windows:
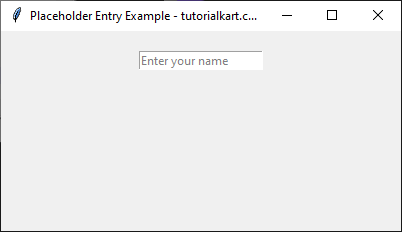
When user clicks on the Entry widget, the placeholder disappears and a cursor appears as shown in the following screenshot.
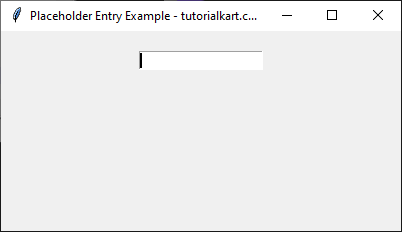
2. Placeholder for Entry with Custom Font and Color
This example extends the previous one by adding a custom font and modifying the placeholder color.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Custom Placeholder Example - tutorialkart.com")
root.geometry("400x200")
def on_entry_click(event):
if entry.get() == "Enter email address":
entry.delete(0, tk.END)
entry.config(fg="black", font=("Arial", 12))
def on_focus_out(event):
if not entry.get():
entry.insert(0, "Enter email address")
entry.config(fg="grey", font=("Arial", 12, "italic"))
entry = tk.Entry(root, fg="grey", font=("Arial", 12, "italic"))
entry.insert(0, "Enter email address")
entry.bind("<FocusIn>", on_entry_click)
entry.bind("<FocusOut>", on_focus_out)
entry.pack(pady=20)
root.mainloop()
Output in Windows:
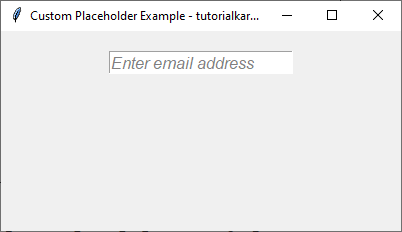
3. Multiple Entry Widgets with Different Placeholders
This example demonstrates how to use placeholders for multiple Entry widgets in a Tkinter form.
main.py
import tkinter as tk
root = tk.Tk()
root.title("Multiple Placeholder Example - tutorialkart.com")
root.geometry("400x200")
def add_placeholder(entry, placeholder_text):
def on_entry_click(event):
if entry.get() == placeholder_text:
entry.delete(0, tk.END)
entry.config(fg="black")
def on_focus_out(event):
if not entry.get():
entry.insert(0, placeholder_text)
entry.config(fg="grey")
entry.insert(0, placeholder_text)
entry.config(fg="grey")
entry.bind("<FocusIn>", on_entry_click)
entry.bind("<FocusOut>", on_focus_out)
# Create Entry widgets with placeholders
entry_name = tk.Entry(root)
add_placeholder(entry_name, "Full Name")
entry_name.pack(pady=5)
entry_email = tk.Entry(root)
add_placeholder(entry_email, "Email Address")
entry_email.pack(pady=5)
entry_password = tk.Entry(root)
add_placeholder(entry_password, "Password")
entry_password.pack(pady=5)
root.mainloop()
Output in Windows:
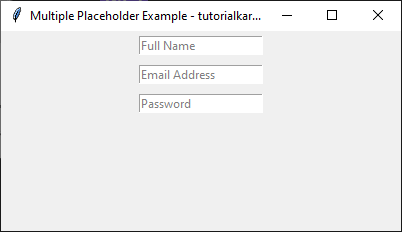
Conclusion
In this tutorial, we explored how to implement placeholders in Tkinter’s Entry
widget. Since Tkinter does not provide a built-in placeholder feature, we used event bindings to create one. The placeholder disappears when the user clicks inside the entry field and reappears if the field is left empty.
- Example 1 demonstrated a basic placeholder effect.
- Example 2 added custom font and styling.
- Example 3 implemented placeholders for multiple entry fields.