Set Placeholder for Text Widget in Tkinter Python
In Tkinter, a Text
widget is used for multi-line text input. However, unlike the Entry
widget, it does not have a built-in placeholder
attribute. To add a placeholder text inside a Text
widget, we can use event bindings to insert default text when the widget is empty and clear it when the user starts typing.
This tutorial will demonstrate different ways to set a placeholder for a Text
widget in a Tkinter application.
Examples
1. Setting a Simple Placeholder in a Text Widget
In this example, we will create a Text
widget with a placeholder that disappears when the user starts typing and reappears if the field is empty.
import tkinter as tk
# Function to handle focus in event
def on_focus_in(event):
if text_widget.get("1.0", "end-1c") == "Enter your text here...":
text_widget.delete("1.0", "end")
text_widget.config(fg="black")
# Function to handle focus out event
def on_focus_out(event):
if text_widget.get("1.0", "end-1c") == "":
text_widget.insert("1.0", "Enter your text here...")
text_widget.config(fg="gray")
# Create main window
root = tk.Tk()
root.title("Placeholder in Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create Text widget with placeholder
text_widget = tk.Text(root, height=5, width=40, fg="gray")
text_widget.insert("1.0", "Enter your text here...")
text_widget.bind("<FocusIn>", on_focus_in)
text_widget.bind("<FocusOut>", on_focus_out)
text_widget.pack(pady=20)
root.mainloop()
Output in Windows:
The placeholder text Enter your text here...
is displayed inside the Text
widget.
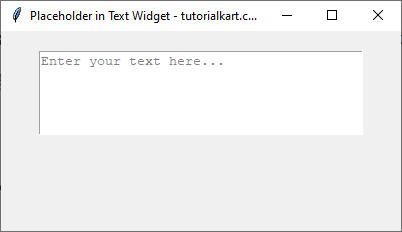
When the user clicks inside the widget, the placeholder disappears.
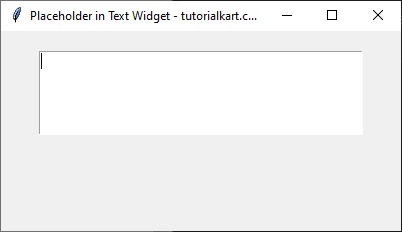
If the user leaves the widget empty and clicks elsewhere, the placeholder reappears.
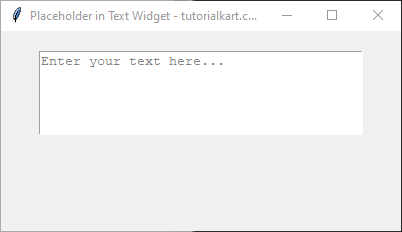
2. Placeholder for Text Widget with Custom Font and Background
In this example, we will style the placeholder with a different font, background color, and text color.
import tkinter as tk
# Function to remove placeholder text
def on_focus_in(event):
if text_widget.get("1.0", "end-1c") == "Type your message...":
text_widget.delete("1.0", "end")
text_widget.config(fg="black", bg="white")
# Function to restore placeholder text
def on_focus_out(event):
if text_widget.get("1.0", "end-1c") == "":
text_widget.insert("1.0", "Type your message...")
text_widget.config(fg="gray", bg="#f0f0f0")
# Create main window
root = tk.Tk()
root.title("Styled Placeholder in Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create Text widget with styled placeholder
text_widget = tk.Text(root, height=5, width=40, font=("Arial", 12), fg="gray", bg="#f0f0f0")
text_widget.insert("1.0", "Type your message...")
text_widget.bind("<FocusIn>", on_focus_in)
text_widget.bind("<FocusOut>", on_focus_out)
text_widget.pack(pady=20)
root.mainloop()
Output in Windows:
The placeholder text Type your message...
is displayed in gray color with a light gray background.
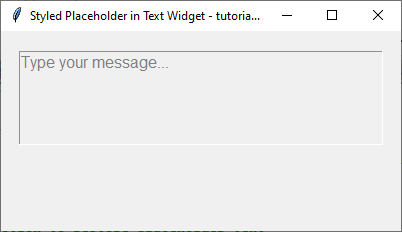
When the user clicks inside the widget, the placeholder disappears, and the text color changes to black with a white background.
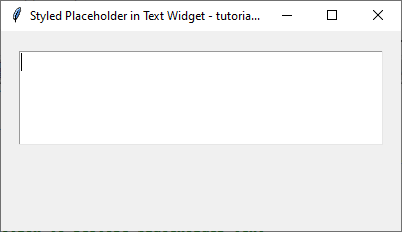
Conclusion
In this tutorial, we explored how to create placeholder text for a Text
widget in Tkinter using event bindings. The placeholder disappears when the user clicks inside the widget and reappears when the widget is left empty. Additionally, we demonstrated how to customize the placeholder with a different font, background, and color.