Set Specific Height for Label in Tkinter Python
In Tkinter, the height
option of a Label widget allows you to control its height. The height is measured in text lines when displaying text and in pixels when using an image. The value assigned to the height
option must be an integer.
To set a specific height for a Label in Tkinter, pass the height
parameter with the required number of lines or pixels while creating the Label.
In this tutorial, we will go through examples using the height
option to modify the size of a Tkinter Label.
Examples
1. Setting Label Height in Tkinter (Text Mode)
In this example, we will create a Tkinter Label and set its height using text lines.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Set Label Height - tutorialkart.com")
root.geometry("400x200")
# Create a label with specific height
label = tk.Label(root, text="This is a label", height=3, bg="lightgray")
label.pack(pady=10)
root.mainloop()
Output in Windows:
A label with a height of 3 text lines appears in the window.
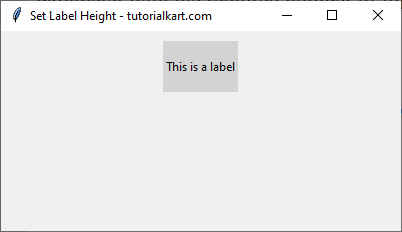
2. Setting Label Height in Pixels
In this example, we will set the height of a Label using pixels by placing it inside a Frame
with a fixed height.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Set Label Height - tutorialkart.com")
root.geometry("400x200")
# Create a Frame with a fixed height
frame = tk.Frame(root, height=50, bg="lightblue")
frame.pack(fill="x", pady=10)
# Place a label inside the frame
label = tk.Label(frame, text="Fixed Height Label", bg="yellow")
label.pack(expand=True, fill="both")
root.mainloop()
Output in Windows:
A label with a height of 50 pixels appears inside a light blue frame.
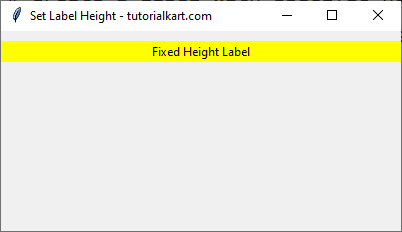
3. Setting Height for Multi-Line Text Label
For multi-line text labels, the height parameter can be adjusted to fit the content properly.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Set Label Height - tutorialkart.com")
root.geometry("400x200")
# Create a multi-line label with increased height
label = tk.Label(root, text="This is a\nmulti-line\nlabel", height=5, bg="lightgreen")
label.pack(pady=10)
root.mainloop()
Output in Windows:
A label with three lines of text and a height of 5 text lines appears.
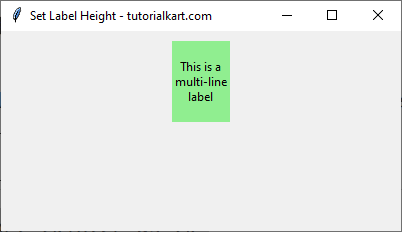
4. Setting Label Height with an Image
When using an image in a Label, the height is measured in pixels. Here’s how to set a specific height for a Label with an image.
import tkinter as tk
from tkinter import PhotoImage
# Create main window
root = tk.Tk()
root.title("Set Label Height - tutorialkart.com")
root.geometry("400x200")
# Load an image
image = PhotoImage(file="background-image.png") # Replace with a valid image path
# Create a label with an image and set height
label = tk.Label(root, image=image, height=100)
label.pack(pady=10)
root.mainloop()
Output in Windows:
A label containing an image appears, with its height set to 100 pixels.
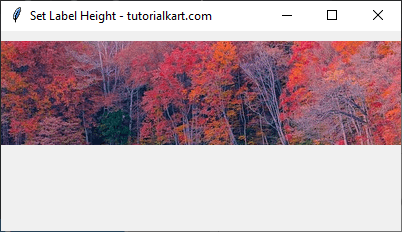
Conclusion
In this tutorial, we explored how to use the height
option in Tkinter’s Label widget to control its height. The height is measured in text lines for text labels and in pixels for image labels. By setting different height values, you can adjust the appearance of labels in your Tkinter application.