Set Specific Height for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used for multi-line text input. The height of a Text widget can be controlled using the height
parameter, which determines the number of text lines displayed.
By default, the Text widget has a height of 24 lines. You can customize this by specifying the number of lines you want while creating the widget.
In this tutorial, we will go through examples of setting a specific height for a Tkinter Text Widget.
Examples
1. Setting a Fixed Height for Text Widget
In this example, we will create a Tkinter window with a Text widget of height 5 lines.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Text Widget Height Example - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with a height of 5 lines
text_widget = tk.Text(root, height=5)
text_widget.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
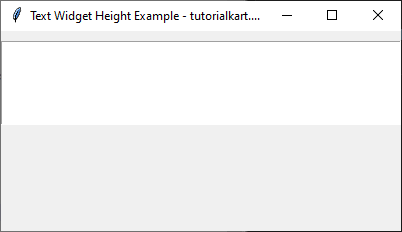
You can enter some text into this Text widget.
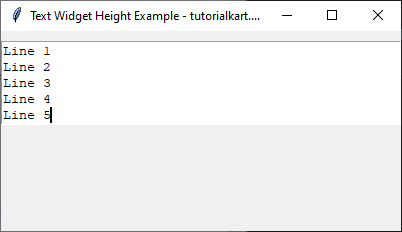
2. Setting a Larger Height for Text Widget
In this example, we will create a larger Text widget with a height of 10 lines.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Large Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with a height of 10 lines
text_widget = tk.Text(root, height=10)
text_widget.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
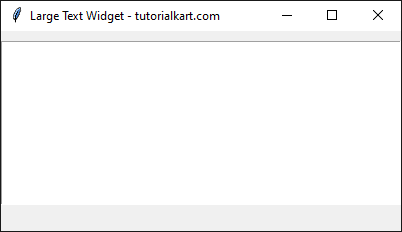
You can enter some text into this Text widget.
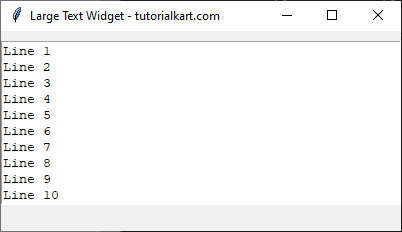
Conclusion
In this tutorial, we explored how to use the height
option in Tkinter’s Text widget to control the number of lines displayed. The height value represents the number of text lines visible in the widget. By setting different height values, you can adjust the appearance of the Text widget in your Tkinter application.