Set Specific Size for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used for multi-line text input and display. The width
and height
options allow you to set a specific size for the Text
widget. The width
is measured in characters, and the height
is measured in lines.
To set a specific size for the Text
widget, you need to pass the width
and height
parameters while creating the widget.
In this tutorial, we will explore how to use the width
and height
options to control the size of a Tkinter Text Widget.
Examples
1. Setting Specific Width and Height for Text Widget
In this example, we create a Text
widget with a width of 40 characters and a height of 10 lines.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Text Widget - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with specific size
text_widget = tk.Text(root, width=40, height=10)
text_widget.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
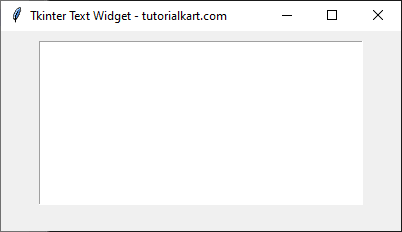
You can enter some text into this Text widget.
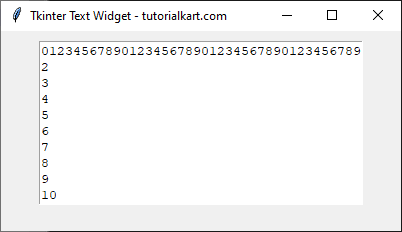
2. Setting Text Widget with Scrollbar
In this example, we create a Text
widget with a fixed width and height, along with a vertical scrollbar.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Text Widget with Scrollbar - tutorialkart.com")
root.geometry("400x200")
# Create a frame to hold the Text widget and Scrollbar
frame = tk.Frame(root)
frame.pack(pady=10)
# Create a Text widget with a fixed size
text_widget = tk.Text(frame, width=40, height=8, wrap="word")
text_widget.pack(side="left", fill="both", expand=True)
# Create a vertical scrollbar
scrollbar = tk.Scrollbar(frame, command=text_widget.yview)
scrollbar.pack(side="right", fill="y")
# Attach the scrollbar to the Text widget
text_widget.config(yscrollcommand=scrollbar.set)
# Run the main event loop
root.mainloop()
Output in Windows:
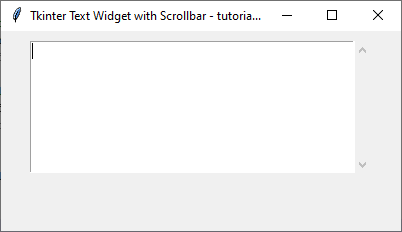
Enter some text that spans more lines than that of Text widget, then we could see the scroll bar.
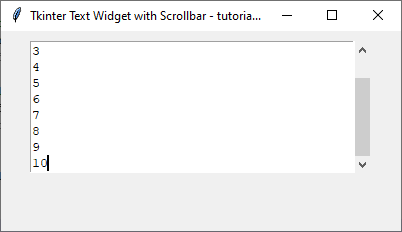
Conclusion
In this tutorial, we explored how to set a specific size for the Text
widget in Tkinter by using the width
and height
options. We also demonstrated how to add a scrollbar to a Text
widget.
- Width is measured in characters.
- Height is measured in lines.
- A scrollbar can be added to handle larger text content.