Set Specific Width for Label in Tkinter Python
In Tkinter, the width
option of a Label widget allows you to control its width. The width is measured in characters when using text and in pixels when using an image. The value assigned to the width
option must be an integer.
To set a specific width for a Label in Tkinter, pass the width
parameter with the required number of character spaces or pixels while creating the Label.
In this tutorial, we will go through examples using the width
option to modify the size of a Tkinter Label.
Examples
1. Setting Label Width in Tkinter
In this example, we will create a Tkinter window and set different width values for Label widgets.
The first label will have a width of 10 characters.
tk.Label(root, text="Short Text", width=10)
And the second label will have a width of 20 characters.
tk.Label(root, text="Longer Text Example", width=20)
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Label Width Example - tutorialkart.com")
root.geometry("400x200")
# Create labels with different widths
label1 = tk.Label(root, text="Short Text", width=10, bg="lightgray")
label1.pack(pady=10)
label2 = tk.Label(root, text="Longer Text Example", width=20, bg="lightblue")
label2.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
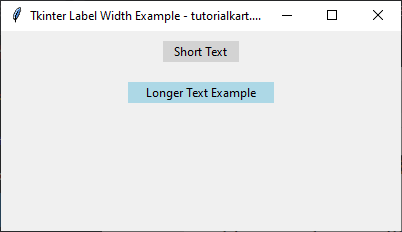
2. Setting Label Width in Pixels for Image
In this example, we will set the width of a Label containing an image. The width is measured in pixels.
tk.Label(root, image=image, width=200)
The actual width of the image is 100 pixels. Since we have specified a width of 200 pixels for the label, the image is placed in the center of the label.
main.py
import tkinter as tk
from tkinter import PhotoImage
root = tk.Tk()
root.title("Image Label Example - tutorialkart.com")
root.geometry("400x200")
# Load an image
image = PhotoImage(file="background-image.png") # Replace with a valid image path
# Create an image label with specific width
label = tk.Label(root, image=image, width=200)
label.pack(pady=20)
root.mainloop()
Output in Windows:
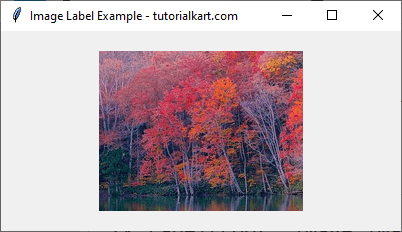
Conclusion
In this tutorial, we explored how to use the width
option in Tkinter’s Label widget to control its width. The width is measured in characters for text labels and in pixels for image labels. By setting different width values, you can adjust the appearance of labels in your Tkinter application.