Set Specific Width for Text Widget in Tkinter Python
In Tkinter, the width
option of a Text
widget allows you to control its width. The width is measured in characters, meaning that it specifies how many average-sized characters can fit in a single line of the text box.
To set a specific width for a Text widget in Tkinter, pass the width
parameter with the required number of character spaces while creating the Text widget.
In this tutorial, we will go through examples using the width
option to modify the size of a Tkinter Text Widget.
Examples
1. Setting a Fixed Width for a Text Widget
In this example, we will create a Tkinter window with a Text widget of a fixed width. The width is set to 40
characters.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Text Width Example - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget with a fixed width of 40 characters
text_widget = tk.Text(root, width=40, height=5)
text_widget.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
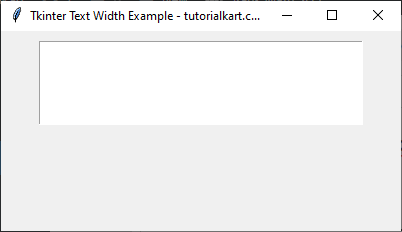
The Text widget has a width of 40 characters and allows multi-line input.
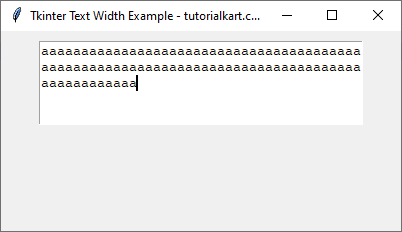
2. Setting a Dynamic Width for a Text Widget with Different Font Sizes
In this example, we will create two Text widgets with different font sizes to see how the width affects different font sizes.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Dynamic Text Width - tutorialkart.com")
root.geometry("500x200")
# Create a Text widget with a standard font size
text_widget1 = tk.Text(root, width=30, height=3, font=("Arial", 12))
text_widget1.insert("1.0", "This is a normal font text box.")
text_widget1.pack(pady=5)
# Create another Text widget with a larger font size
text_widget2 = tk.Text(root, width=30, height=3, font=("Arial", 20))
text_widget2.insert("1.0", "This is a large font text box.")
text_widget2.pack(pady=5)
# Run the main event loop
root.mainloop()
Output in Windows:
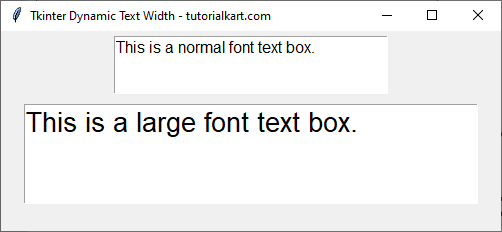
In this example, both Text widgets have the same width (30 characters), but the one with a larger font size appears wider due to increased character size.
Conclusion
In this tutorial, we explored how to use the width
option in Tkinter’s Text widget to control its width. The width is measured in characters, meaning it determines how many characters fit per line. We demonstrated how different font sizes affect the appearance of the Text widget while maintaining the specified width.