Set the Size of Button in Tkinter
In Tkinter, you can control the size of a Button widget using the width
and height
parameters. The width
parameter sets the button’s width in terms of the number of characters, while the height
parameter sets the button’s height in terms of the number of text lines.
For buttons with images, the width
and height
values are measured in pixels.
In this tutorial, we will go through examples demonstrating how to adjust the size of a Tkinter Button using both text and images.
Examples
1. Setting Button Size Using width
and height
In this example, we create two buttons with different sizes. The first button has a width of 15 characters and a height of 2 text lines, while the second button has a width of 20 characters and a height of 3 text lines.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Button Size Example - tutorialkart.com")
root.geometry("400x200")
# Create buttons with different sizes
btn_small = tk.Button(root, text="Small Button", width=15, height=2)
btn_small.pack(pady=10)
btn_large = tk.Button(root, text="Large Button", width=20, height=3)
btn_large.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
Two buttons with different widths and heights are displayed.
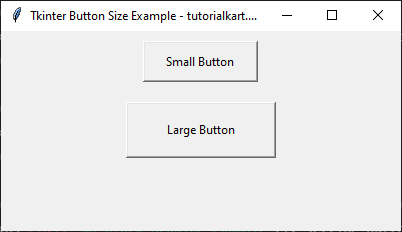
2. Setting Button Size for an Image Button
In this example, we create a Button with an image. The button size is set to 100 pixels in width and 50 pixels in height.
main.py
import tkinter as tk
from tkinter import PhotoImage
# Create the main window
root = tk.Tk()
root.title("Image Button Example - tutorialkart.com")
root.geometry("400x200")
def on_click():
print("Image Button Clicked!")
# Load an image
image = PhotoImage(file="button_icon.png") # Replace with a valid image path
# Create an image button with specified size
button = tk.Button(root, image=image, command=on_click, width=100, height=50)
button.pack(pady=20)
root.mainloop()
Output:
An image button with a size of 100×50 pixels is displayed.
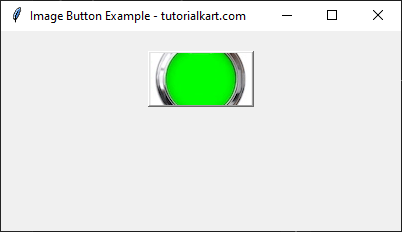
Conclusion
In this tutorial, we explored how to control the size of a Tkinter Button using the width
and height
options. For text-based buttons, the values are measured in characters and text lines, while for image buttons, they are measured in pixels.
By adjusting these parameters, you can customize the appearance of buttons to fit your application’s design.