Set Vertical Scrollbar for Text Widget in Tkinter Python
In Tkinter, a Scrollbar
widget can be used to add scrolling capability to other widgets, such as the Text
widget. By default, a Text
widget does not include scrollbars, so we need to attach a vertical scrollbar manually.
To add a vertical scrollbar to a Text
widget, we use the Scrollbar
class and link it using the yscrollcommand
parameter.
In this tutorial, we will explore different ways to add a vertical scrollbar to a Tkinter Text Widget.
Examples
1. Adding a Vertical Scrollbar to a Text Widget
In this example, we will create a Text
widget and attach a vertical scrollbar to it.
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Text Scrollbar Example - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, wrap="word", height=5, width=40)
# Create a vertical scrollbar
scrollbar = tk.Scrollbar(root, orient="vertical", command=text_widget.yview)
# Link the scrollbar to the Text widget
text_widget.config(yscrollcommand=scrollbar.set)
# Pack widgets
text_widget.pack(side="left", fill="both", expand=True)
scrollbar.pack(side="right", fill="y")
# Insert sample text
text_widget.insert("1.0", "This is an example of a Text widget with a vertical scrollbar.\n" * 10)
# Run the main loop
root.mainloop()
Output in Windows:
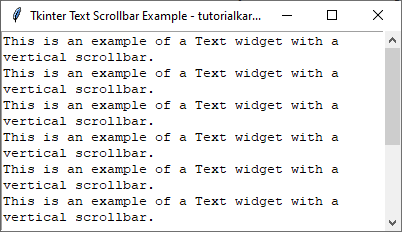
2. Adding Scrollbar Inside a Frame for Text Widget
In this example, we will place the Text
widget and its scrollbar inside a Frame
for better layout control.
</>
Copy
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tkinter Text Scrollbar in Frame - tutorialkart.com")
root.geometry("400x200")
# Create a Frame to hold the Text widget and Scrollbar
frame = tk.Frame(root)
frame.pack(expand=True, fill="both", padx=10, pady=10)
# Create a Text widget
text_widget = tk.Text(frame, wrap="word", height=5, width=40)
# Create a vertical scrollbar
scrollbar = tk.Scrollbar(frame, orient="vertical", command=text_widget.yview)
# Link the scrollbar to the Text widget
text_widget.config(yscrollcommand=scrollbar.set)
# Place widgets inside the frame
text_widget.grid(row=0, column=0, sticky="nsew")
scrollbar.grid(row=0, column=1, sticky="ns")
# Configure grid resizing
frame.columnconfigure(0, weight=1)
frame.rowconfigure(0, weight=1)
# Insert sample text
text_widget.insert("1.0", "This is a Text widget inside a Frame with a scrollbar.\n" * 10)
# Run the main loop
root.mainloop()
Output in Windows:
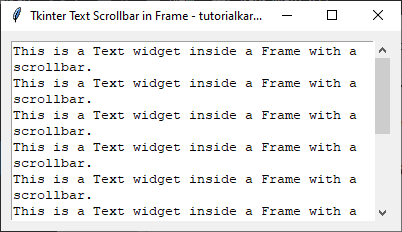
Conclusion
In this tutorial, we explored how to add a vertical scrollbar to a Tkinter Text
widget. We covered:
- How to link a
Scrollbar
to aText
widget using theyscrollcommand
option. - How to structure the
Text
widget and scrollbar inside aFrame
for better layout management.