Set Width for Entry Widget in Tkinter Python
In Tkinter, the width
option of the Entry
widget allows you to specify the width of the input field. The width is measured in characters, meaning it determines how many characters can be displayed in the entry box at a time.
To set a specific width for an Entry widget in Tkinter, pass the width
parameter with the required number of characters when creating the Entry widget.
In this tutorial, we will go through examples using the width
option to modify the size of a Tkinter Entry widget.
Examples
1. Setting Entry Widget Width in Tkinter
In this example, we will create a Tkinter window and define Entry widgets with different widths.
The first Entry widget will have a width of 10 characters.
tk.Entry(root, width=10)
The second Entry widget will have a width of 20 characters.
tk.Entry(root, width=20)
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Entry Widget Width Example - tutorialkart.com")
root.geometry("400x200")
# Create Entry widgets with different widths
entry_small = tk.Entry(root, width=10)
entry_small.pack(pady=10)
entry_large = tk.Entry(root, width=20)
entry_large.pack(pady=10)
# Run the main event loop
root.mainloop()
Output in Windows:
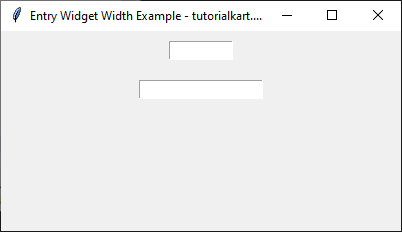
2. Setting Entry Widget Width with Placeholder Text
In this example, we will set a default text (placeholder) in the Entry widget and adjust its width.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Entry Width with Placeholder - tutorialkart.com")
root.geometry("400x200")
# Create an Entry widget with width and default text
entry = tk.Entry(root, width=25)
entry.insert(0, "Enter your name here") # Placeholder text
entry.pack(pady=10)
root.mainloop()
Output in Windows:
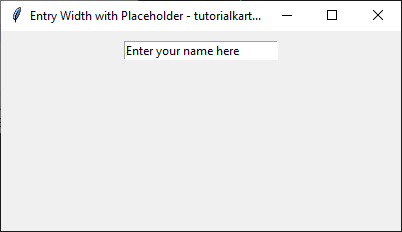
3. Setting Entry Width Dynamically Using Grid Layout
In this example, we will dynamically adjust the Entry widget width using the columnspan
option in a grid
layout.
import tkinter as tk
root = tk.Tk()
root.title("Entry Width in Grid - tutorialkart.com")
root.geometry("400x200")
# Label
label = tk.Label(root, text="Username:")
label.grid(row=0, column=0, padx=10, pady=10)
# Entry field with dynamic width
entry = tk.Entry(root, width=30)
entry.grid(row=0, column=1, columnspan=2, padx=10, pady=10)
root.mainloop()
Output in Windows:
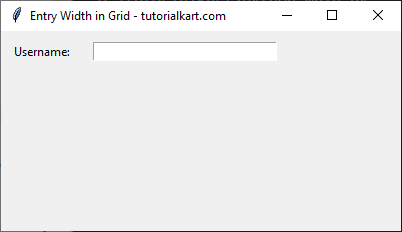
Conclusion
In this tutorial, we explored how to use the width
option in Tkinter’s Entry widget to control the input field size. The width is measured in characters. By adjusting the width, you can customize the appearance of input fields in your Tkinter application.