Setup Change Event for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to accept single-line text input. To detect changes in the input field, we can use a Tkinter StringVar
variable with a trace callback.
By setting up a change event, we can execute a function whenever the user types or modifies the input in the Entry
widget.
Examples
1. Using StringVar with trace_add for Entry Widget
Another approach to detect text changes is by using a Tkinter StringVar
variable. The trace_add
method monitors changes and executes a callback function.
import tkinter as tk
def on_text_change(*args):
print("Updated text:", text_var.get())
root = tk.Tk()
root.title("Entry Change Event - tutorialkart.com")
root.geometry("400x200")
# StringVar to track changes
text_var = tk.StringVar()
text_var.trace_add("write", on_text_change)
entry = tk.Entry(root, textvariable=text_var)
entry.pack(pady=10)
root.mainloop()
Output in Windows:
As the user types, the updated text is printed to the console.
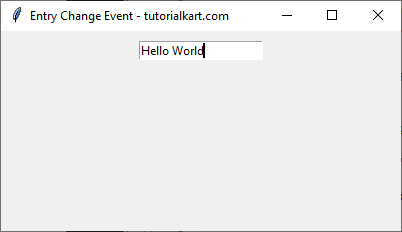
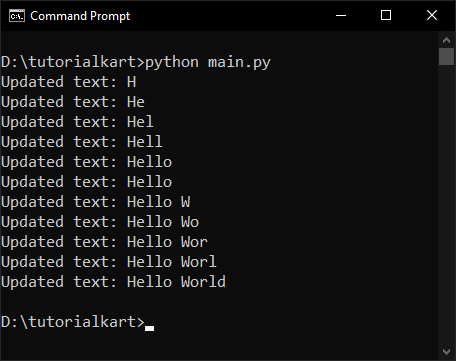
2. Displaying Live Changes in a Label when Value in Entry Widget Changes
We can also update a Label
dynamically as the user types in the Entry
widget.
import tkinter as tk
def on_text_change(*args):
label.config(text="You typed: " + text_var.get())
root = tk.Tk()
root.title("Entry Change Event - tutorialkart.com")
root.geometry("400x200")
text_var = tk.StringVar()
text_var.trace_add("write", on_text_change)
entry = tk.Entry(root, textvariable=text_var)
entry.pack(pady=10)
label = tk.Label(root, text="You typed: ")
label.pack(pady=10)
root.mainloop()
Output in Windows:
As the user types, the label updates dynamically to reflect the input.
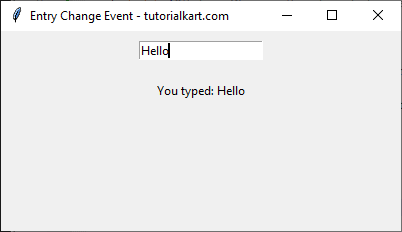
Conclusion
In this tutorial, we explored different methods to detect and handle change events in Tkinter’s Entry
widget:
- Using the
<<Modified>>
event to trigger changes. - Using a
StringVar
withtrace_add
to monitor text updates. - Displaying live updates using a
Label
.
These methods help in real-time text validation, search suggestions, and dynamic UI updates in Tkinter applications.