Setup Validation for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is commonly used for user input. To ensure that users enter valid data, we can use the validate
and validatecommand
options to apply real-time validation.
Entry validation in Tkinter can be set using the following:
validate="key"
– Validates input when a key is pressed.validatecommand
– A function that returnsTrue
for valid input andFalse
otherwise.invalidcommand
– A function that runs when validation fails.
In this tutorial, we will go through multiple examples of setting up validation for an Entry Widget in Tkinter.
Examples
1. Validating Numeric Input
In this example, we allow only numeric input in the Entry widget.
import tkinter as tk
def validate_numeric_input(P):
return P.isdigit() or P == ""
root = tk.Tk()
root.title("Entry Validation - tutorialkart.com")
root.geometry("400x200")
vcmd = root.register(validate_numeric_input)
entry = tk.Entry(root, validate="key", validatecommand=(vcmd, "%P"))
entry.pack(pady=10)
root.mainloop()
Output in Windows:
An Entry widget is displayed. It only accepts numeric values. Even if you try entering alphabets, they won’t appear in the Entry widget.
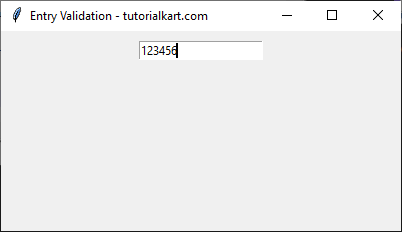
2. Validating Alphabetic Input
In this example, we allow only alphabetic characters (letters) in the Entry widget.
import tkinter as tk
def validate_alpha_input(P):
return P.isalpha() or P == ""
root = tk.Tk()
root.title("Entry Validation - tutorialkart.com")
root.geometry("400x200")
vcmd = root.register(validate_alpha_input)
entry = tk.Entry(root, validate="key", validatecommand=(vcmd, "%P"))
entry.pack(pady=10)
root.mainloop()
Output in Windows:
An Entry widget is displayed. It only allows alphabetic characters.
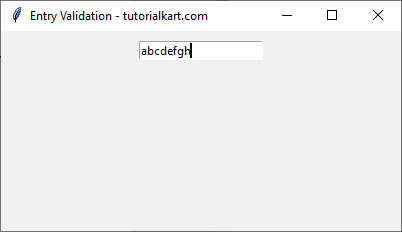
Conclusion
In this tutorial, we explored how to use the validate
and validatecommand
options in Tkinter’s Entry widget to enforce input rules. We covered validation for numeric input and alphabetic input.