Setup Validation for Text Widget in Tkinter Python
In Tkinter, the Text
widget is used to accept multiline user input. To ensure the validity of user input, we can apply validation using event bindings and the validate
and validatecommand
options.
Validation helps restrict input based on certain rules, such as allowing only numeric values, limiting character count, or preventing unwanted characters.
In this tutorial, we will explore how to set up validation for a Text
widget in a Tkinter application.
Examples
1. Restricting Input to Numeric Values in Text Widget
In this example, we will create a Text
widget and restrict its input to numeric values only.
We will bind KeyRelease
event with validate_numeric
function. This function is called whenever the key-release event happens in the Text Widget. When this function is called, it reads the input from the text widget and validates its. If the input is not a digit, then the function deletes that character.
import tkinter as tk
# Function to validate numeric input
def validate_numeric(event):
text = text_widget.get("1.0", "end-1c") # Get text content
if not text.isdigit(): # Check if input is not numeric
text_widget.delete("1.0", tk.END) # Clear invalid input
text_widget.insert("1.0", "".join(filter(str.isdigit, text))) # Keep only digits
# Create main window
root = tk.Tk()
root.title("Text Widget Validation - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=3, width=30)
text_widget.pack(pady=10)
# Bind the validation function
text_widget.bind("<KeyRelease>", validate_numeric)
root.mainloop()
Output in Windows:
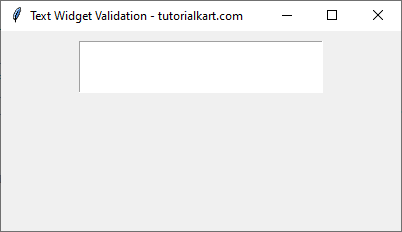
The text widget only accepts numeric values. If the user enters letters or symbols, they are automatically removed.
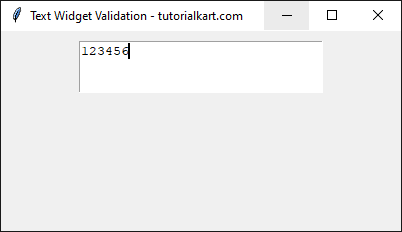
2. Limiting Character Count in Text Widget
In this example, we will limit the input in the Text
widget to a maximum of 20 characters.
import tkinter as tk
# Function to limit text length
def limit_text(event):
text = text_widget.get("1.0", "end-1c") # Get text content
if len(text) > 20: # Limit to 20 characters
text_widget.delete("1.0", tk.END) # Clear existing text
text_widget.insert("1.0", text[:20]) # Allow only 20 characters
# Create main window
root = tk.Tk()
root.title("Text Widget Validation - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=3, width=30)
text_widget.pack(pady=10)
# Bind the validation function
text_widget.bind("<KeyRelease>", limit_text)
root.mainloop()
Output in Windows:
The user cannot enter more than 20 characters in the text widget. If they attempt to enter additional characters, the input is automatically truncated.
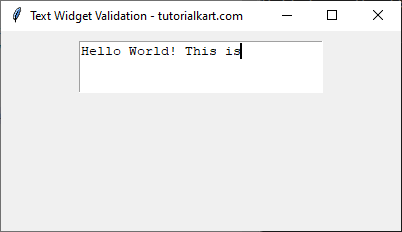
Conclusion
In this tutorial, we explored how to apply validation to the Text
widget in Tkinter. We covered:
- Restricting input to numeric values
- Limiting the number of characters entered