Toggle Password Visibility in Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to accept user input. When dealing with password fields, you can use the show
attribute to mask the characters. However, to allow users to toggle password visibility, you need to modify the show
attribute dynamically.
In this tutorial, we will explore different ways to toggle password visibility in the Tkinter Entry
widget using the show
attribute.
Examples
1. Basic Toggle Password Visibility
In this example, we create a password entry field and a button that toggles the visibility of the password.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Toggle Password Visibility - tutorialkart.com")
root.geometry("400x200")
# Function to toggle password visibility
def toggle_password():
if entry_password.cget("show") == "":
entry_password.config(show="*")
btn_toggle.config(text="Show Password")
else:
entry_password.config(show="")
btn_toggle.config(text="Hide Password")
# Create password entry field
entry_password = tk.Entry(root, width=30, show="*")
entry_password.pack(pady=10)
# Create toggle button
btn_toggle = tk.Button(root, text="Show Password", command=toggle_password)
btn_toggle.pack(pady=10)
# Run main loop
root.mainloop()
Output in Windows:
After entering some password in the Entry widget.
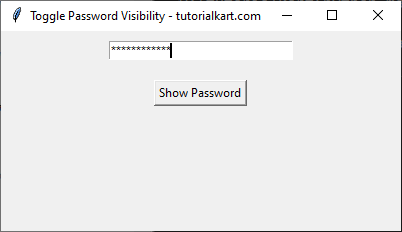
Click on Show Password button.
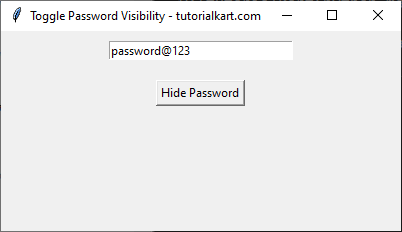
If you click on the Hide Password button, the password shall be hidden.
2. Toggle Password Visibility Using a Checkbox
This example uses a checkbox instead of a button to toggle password visibility.
import tkinter as tk
# Create main window
root = tk.Tk()
root.title("Toggle Password Visibility - tutorialkart.com")
root.geometry("400x200")
# Function to toggle password visibility
def toggle_password():
if var.get():
entry_password.config(show="")
else:
entry_password.config(show="*")
# Create password entry field
entry_password = tk.Entry(root, width=30, show="*")
entry_password.pack(pady=10)
# Create checkbox
var = tk.BooleanVar()
chk_toggle = tk.Checkbutton(root, text="Show Password", variable=var, command=toggle_password)
chk_toggle.pack(pady=10)
# Run main loop
root.mainloop()
Output in Windows:
When you run this program, an Entry field appears with a Checkbox as shown in the following.
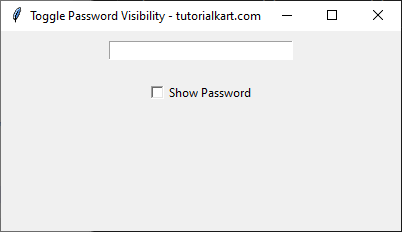
When you enter a password, it is hidden by default.
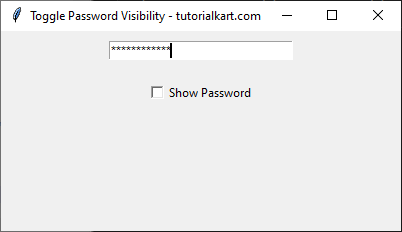
If you check the Show Password checkbox, then the password is displayed.
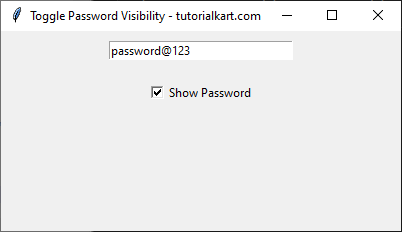
If you uncheck the Show Password checkbox, then the password shall be hidden.
3. Toggle Password Visibility with an Eye Icon
In this example, we use an eye icon button to toggle password visibility.


import tkinter as tk
from tkinter import PhotoImage
# Create main window
root = tk.Tk()
root.title("Toggle Password Visibility - tutorialkart.com")
root.geometry("400x200")
# Function to toggle password visibility
def toggle_password():
if entry_password.cget("show") == "*":
entry_password.config(show="")
btn_toggle.config(image=eye_closed)
else:
entry_password.config(show="*")
btn_toggle.config(image=eye_open)
# Load eye icon images
eye_open = PhotoImage(file="eye_open.png") # Replace with a valid image path
eye_closed = PhotoImage(file="eye_closed.png") # Replace with a valid image path
# Create password entry field
entry_password = tk.Entry(root, width=30, show="*")
entry_password.pack(pady=10)
# Create toggle button with image
btn_toggle = tk.Button(root, image=eye_open, command=toggle_password)
btn_toggle.pack(pady=10)
# Run main loop
root.mainloop()
Output in Windows:
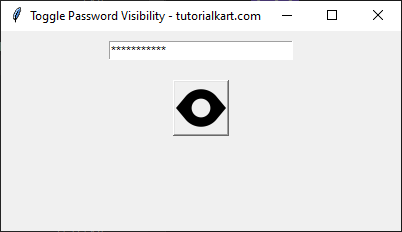
Click on the ‘eye_open’ button.
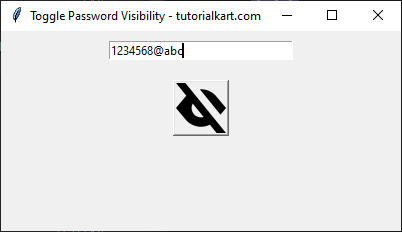
The password is shown and the button is toggled.
Conclusion
In this tutorial, we explored different ways to toggle password visibility in a Tkinter Entry
widget:
- Using a button to toggle visibility.
- Using a checkbox to control visibility.
- Using an eye icon for a more user-friendly approach.