Update Text in a Label on Button Click in Tkinter Python
In Tkinter, a Label
widget can display text, and you can update the text dynamically when a Button
is clicked. This is achieved using the config()
method or the textvariable
option with a Tkinter StringVar.
In this tutorial, we will go through multiple examples to demonstrate how to update the text of a Label widget on a Button click in a Tkinter application.
Examples
1. Updating Label Text Using the config()
Method
In this example, we will update the label text using the config()
method inside a button click function.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Update Label Example - tutorialkart.com")
root.geometry("400x200")
# Function to update label text
def update_text():
label.config(text="Text Updated!")
# Create a label
label = tk.Label(root, text="Original Text", font=("Arial", 14))
label.pack(pady=20)
# Create a button to update the label
button = tk.Button(root, text="Click to Update", command=update_text)
button.pack()
# Run the main event loop
root.mainloop()
Output in Windows:
A window with a button and label appears.
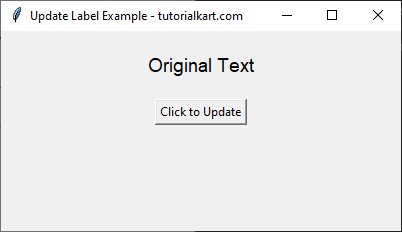
Clicking the button updates the label text from “Original Text” to “Text Updated!”.
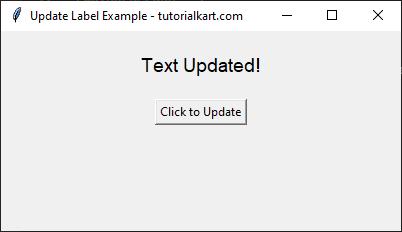
2. Updating Label Text Using a Tkinter StringVar
In this example, we use a Tkinter StringVar
to store and update the label text.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Update Label Example - tutorialkart.com")
root.geometry("400x200")
# StringVar to store label text
label_text = tk.StringVar()
label_text.set("Initial Text")
# Function to update label text
def update_text():
label_text.set("Text Updated!")
# Create a label using StringVar
label = tk.Label(root, textvariable=label_text, font=("Arial", 14))
label.pack(pady=20)
# Create a button to update the label
button = tk.Button(root, text="Click to Update", command=update_text)
button.pack()
# Run the main event loop
root.mainloop()
Output in Windows:
A window with a label and button appears.
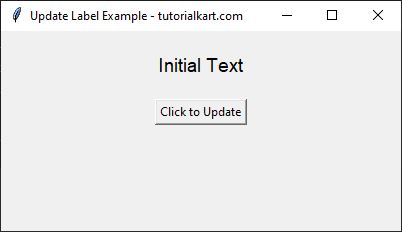
Clicking the button updates the label text using StringVar
.
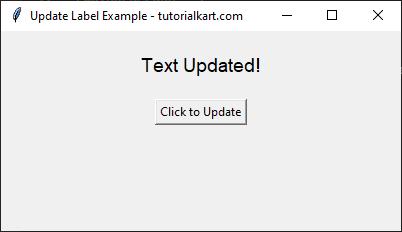
3. Updating Label Text with User Input
In this example, the label text updates dynamically based on user input from an entry field.
main.py
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Update Label with Input - tutorialkart.com")
root.geometry("400x200")
# Function to update label text
def update_text():
label.config(text=entry.get())
# Create an entry field
entry = tk.Entry(root, font=("Arial", 12))
entry.pack(pady=10)
# Create a label
label = tk.Label(root, text="Enter text above", font=("Arial", 14))
label.pack(pady=10)
# Create a button to update the label
button = tk.Button(root, text="Update Label", command=update_text)
button.pack()
# Run the main event loop
root.mainloop()
Output in Windows:
A window with an entry field, label, and button appears.
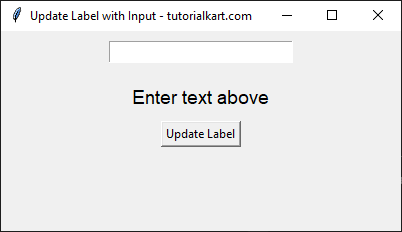
Typing in the entry field and clicking the button updates the label with the entered text.
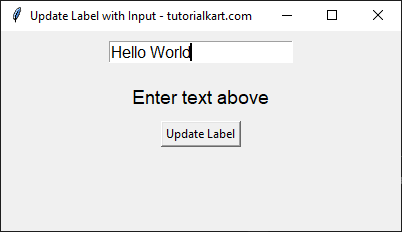
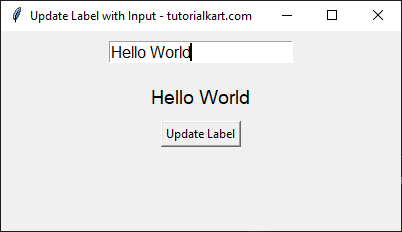
Conclusion
In this tutorial, we explored different ways to update the text of a Label widget in Tkinter when a Button is clicked:
- Using the
config()
method - Using a Tkinter
StringVar
- Updating the label based on user input
These methods allow for dynamic text updates in Tkinter applications, improving interactivity and usability.