Using Entry Widget for Password in Tkinter Python
In Tkinter, the Entry
widget is used to take user input. When dealing with passwords, we can mask the input using the show
parameter. This prevents the password from being visible as the user types.
By setting show="*"
or any other character, we can hide the password input while still allowing user interaction.
Examples
1. Basic Password Entry Field in Tkinter
In this example, we create a simple password entry field where the input is hidden using show="*"
.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Password Entry Example - tutorialkart.com")
root.geometry("400x200")
# Create password label and entry
tk.Label(root, text="Enter Password:").pack(pady=5)
password_entry = tk.Entry(root, show="*") # Mask input with '*'
password_entry.pack(pady=5)
# Run the application
root.mainloop()
Output in Windows:
A password entry field appears, and the input is masked with “*”.
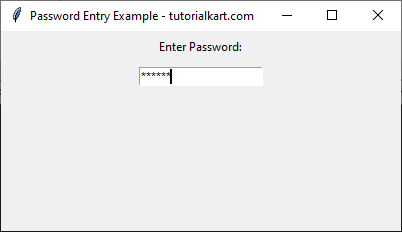
2. Display Entered Password in Entry Using a Button in Tkinter
This example adds a button that reveals the entered password when clicked.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Show Password Example - tutorialkart.com")
root.geometry("400x200")
# Function to display password
def show_password():
password = password_entry.get()
label_result.config(text=f"Entered Password: {password}")
# Create password label and entry
tk.Label(root, text="Enter Password:").pack(pady=5)
password_entry = tk.Entry(root, show="*")
password_entry.pack(pady=5)
# Button to reveal password
btn_show = tk.Button(root, text="Show Password", command=show_password)
btn_show.pack(pady=5)
# Label to display password
label_result = tk.Label(root, text="")
label_result.pack(pady=5)
# Run the application
root.mainloop()
Output in Windows:
The entered password is displayed in a label when the button is clicked.
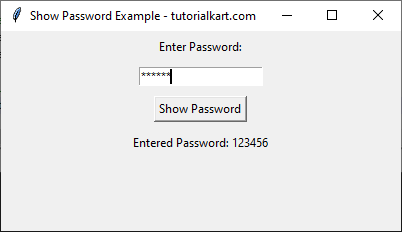
3. Toggle Password Visibility in Entry Widget
This example allows users to toggle password visibility by clicking a checkbox.
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Toggle Password Visibility - tutorialkart.com")
root.geometry("400x200")
# Function to toggle password visibility
def toggle_password():
if password_entry.cget("show") == "*":
password_entry.config(show="")
else:
password_entry.config(show="*")
# Create password label and entry
tk.Label(root, text="Enter Password:").pack(pady=5)
password_entry = tk.Entry(root, show="*")
password_entry.pack(pady=5)
# Checkbox to toggle password visibility
chk_show = tk.Checkbutton(root, text="Show Password", command=toggle_password)
chk_show.pack(pady=5)
# Run the application
root.mainloop()
Output in Windows:
Clicking the checkbox toggles between hiding and showing the password input.
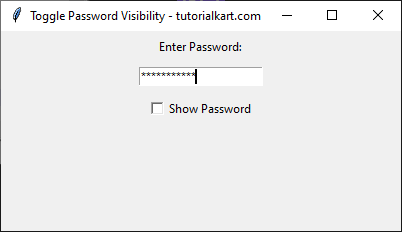
If you click on the ‘Show Password’ checkbox, the password is displayed.
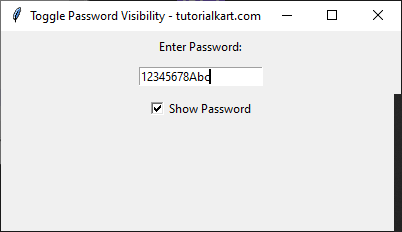
Conclusion
In this tutorial, we explored different ways to use the Entry
widget in Tkinter for password input:
- Masking password input using
show="*"
- Displaying the entered password with a button
- Toggling password visibility using a checkbox