Python Tkinter Listbox
Tkinter Listbox widget allows user to select one or more items from a list.
In this tutorial, we will learn how to create a Listbox widget in Python GUI application, and the options available for Listbox widget class.
Example 1 – Tkinter Listbox
In the following program, we will create a Tkinter Listbox widget with five items.
example.py – Python Program
import tkinter as tk
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry("400x200")
listbox_1 = tk.Listbox(window_main)
listbox_1.insert(1, "Apple")
listbox_1.insert(2, "Banana")
listbox_1.insert(3, "Cherry")
listbox_1.insert(4, "Mango")
listbox_1.insert(5, "Orange")
listbox_1.pack()
window_main.mainloop()
Output
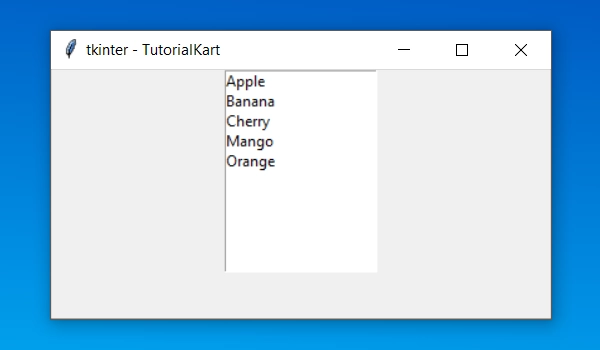
Example 2 – Tkinter Listbox – Get Selection
In this tutorial, we will create a Listbox, and read what user has selected from the list of items.
Listbox.curselection() function returns a tuple containing index of selected items.
example.py – Python Program
import tkinter as tk
window_main = tk.Tk(className='Tkinter - TutorialKart')
window_main.geometry('400x200')
listbox_1 = tk.Listbox(window_main, selectmode=tk.EXTENDED)
listbox_1.insert(1, 'Apple')
listbox_1.insert(2, 'Banana')
listbox_1.insert(3, 'Cherry')
listbox_1.insert(4, 'Mango')
listbox_1.insert(5, 'Orange')
listbox_1.pack()
def submitFunction():
selection = listbox_1.curselection()
print('Listbox selection :', selection)
submit = tk.Button(window_main, text='Submit', command=submitFunction)
submit.pack()
window_main.mainloop()
Output
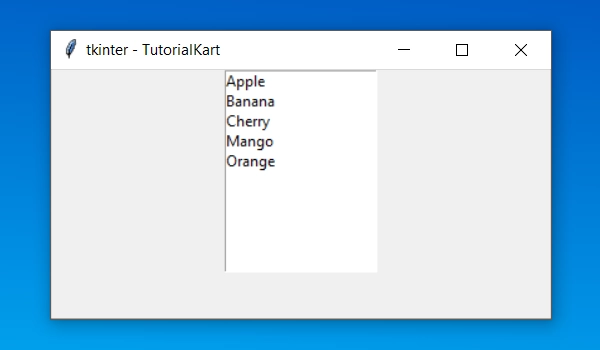
Select one ore more items as shown below.
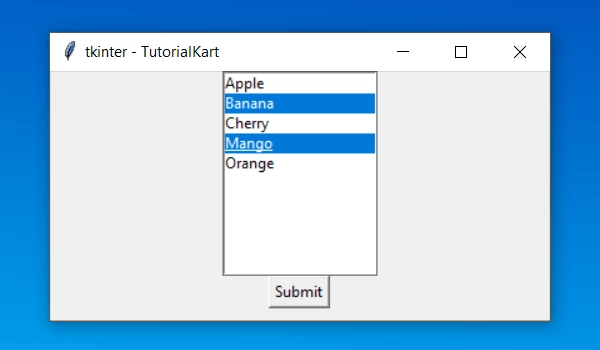
Click on Submit button. When user clicks on Submit button, we are calling the function submitFunction() where we read the Listbox selection using curselection() function.
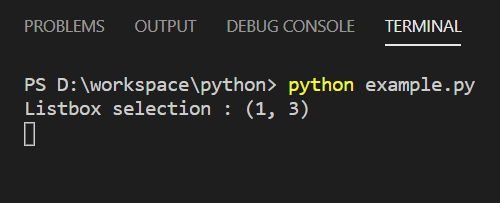
Conclusion
In this Python Tutorial, we learned how to create a Listbox widget in our GUI application, and how to work with it for user interaction.