Tkinter Text Widget
The Text
widget in Tkinter is a multiline text area where users can enter and edit text. Unlike the Entry
widget, which is limited to a single line, the Text
widget allows for multiple lines of text and supports various text formatting features.
The Text
widget is useful for implementing text editors, chat applications, and log viewers in a Tkinter-based GUI application.
Syntax
tk.Text(master, **options)
Parameters
Parameter | Type | Description |
---|---|---|
master | Widget | The parent widget, usually a frame or root window. |
height | Integer | Specifies the number of lines the text widget should have. |
width | Integer | Specifies the number of characters per line. |
bg | String | Sets the background color of the text widget. |
fg | String | Sets the text color. |
font | Tuple | Defines the font type, size, and style. |
wrap | String | Defines text wrapping behavior (none , char , or word ). |
Examples
1. Creating a Basic Text Widget
In this example, we create a simple Text
widget inside a Tkinter window.
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Example - tutorialkart.com")
root.geometry("400x200")
# Create a Text widget
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
root.mainloop()
Output in Windows:
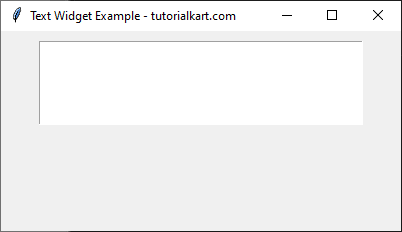
A blank text area appears, allowing users to type multiple lines of text.
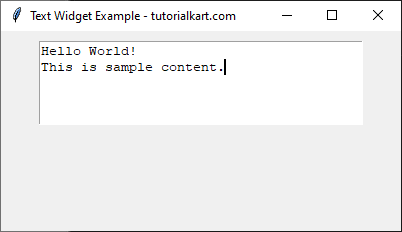
2. Setting Default Text in Text Widget
We can insert default text into the Text
widget using the insert
method.
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Example - tutorialkart.com")
root.geometry("400x200")
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Insert default text
text_widget.insert("1.0", "Welcome to the Tkinter Text Widget!")
root.mainloop()
Output in Windows:
The text area appears with the default text “Welcome to the Tkinter Text Widget!” already inserted.
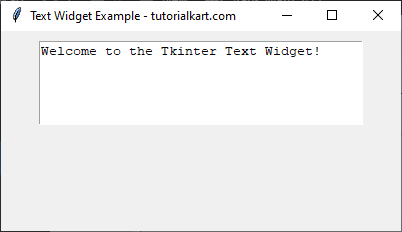
3. Retrieving Text from the Text Widget
We can retrieve text from the Text
widget using the get
method. Here’s an example where we display the entered text in the console.
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Example - tutorialkart.com")
root.geometry("400x200")
def get_text():
text = text_widget.get("1.0", "end-1c") # Retrieve text from the widget
print("Entered text:", text)
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
# Create a button to retrieve text
btn = tk.Button(root, text="Get Text", command=get_text)
btn.pack()
root.mainloop()
Output in Windows:
When the user clicks the “Get Text” button, the entered text is printed in the console.
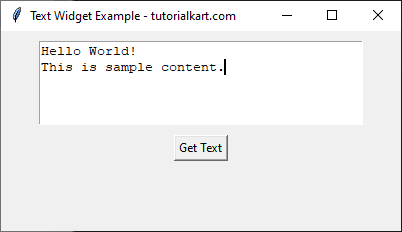
Enter some text in the input field and click on the button.
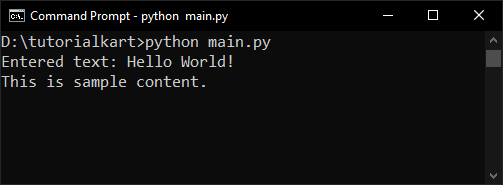
4. Disabling Editing in the Text Widget
To make the text widget read-only, we can set the state
property to disabled
.
import tkinter as tk
root = tk.Tk()
root.title("Text Widget Example - tutorialkart.com")
root.geometry("400x200")
text_widget = tk.Text(root, height=5, width=40)
text_widget.pack(pady=10)
text_widget.insert("1.0", "This text is read-only.")
text_widget.config(state="disabled") # Disable editing
root.mainloop()
Output in Windows:
A text area appears with pre-filled text that users cannot edit.
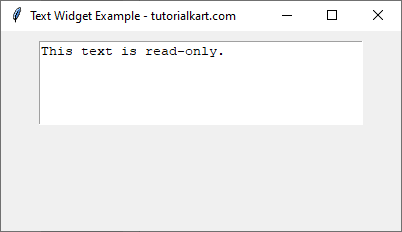
Conclusion
In this tutorial, we explored the Tkinter Text
widget, including:
- Creating a basic text widget
- Setting default text
- Retrieving text entered by users
- Disabling text editing