Events Available for Entry Widget in Tkinter Python
In Tkinter, the Entry
widget is used to accept user input. Various events can be bound to the Entry
widget to detect user interactions, such as key presses, focus changes, and mouse clicks.
Events in Tkinter are triggered by user actions, and we can use the bind()
method to execute specific functions when an event occurs. Some common events for the Entry
widget include:
<KeyPress>
– Detects when a key is pressed.<KeyRelease>
– Detects when a key is released.<FocusIn>
– Detects when the Entry widget gains focus.<FocusOut>
– Detects when the Entry widget loses focus.<Button-1>
– Detects when the left mouse button is clicked inside the Entry widget.<Return>
– Detects when the Enter key is pressed.
In this tutorial, we will explore how to use these events with examples.
Examples
1. Detecting Key Press in Entry Widget
In this example, we bind the <KeyPress>
event to detect when a key is pressed inside the Entry widget.
import tkinter as tk
def on_key_press(event):
label.config(text=f"Key Pressed: {event.char}")
root = tk.Tk()
root.title("Entry Key Press Event - tutorialkart.com")
root.geometry("400x200")
entry = tk.Entry(root)
entry.pack(pady=10)
label = tk.Label(root, text="Press a key in the Entry box")
label.pack(pady=10)
entry.bind("<KeyPress>", on_key_press)
root.mainloop()
Output in Windows:
Initial Screen.
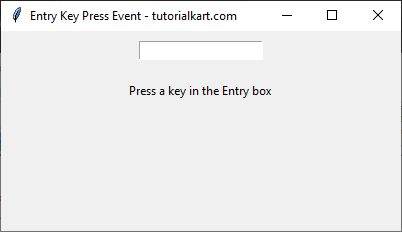
When a key is pressed inside the Entry widget, the key’s character is displayed below.
2. Detecting Entry Widget Focus
In this example, we bind <FocusIn>
and <FocusOut>
events to detect when the Entry widget gains or loses focus.
import tkinter as tk
def on_focus_in(event):
entry.config(bg="lightyellow")
def on_focus_out(event):
entry.config(bg="white")
root = tk.Tk()
root.title("Entry Focus Events - tutorialkart.com")
root.geometry("400x200")
entry = tk.Entry(root)
entry.pack(pady=10)
entry.bind("<FocusIn>", on_focus_in)
entry.bind("<FocusOut>", on_focus_out)
root.mainloop()
Output in Windows:
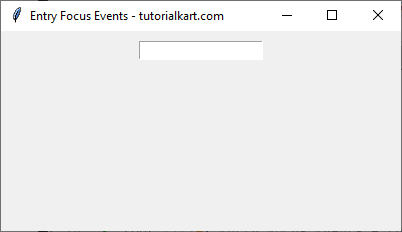
When the Entry widget gains focus, it turns yellow.
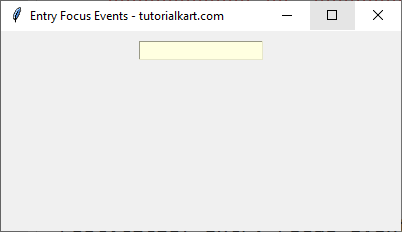
When it loses focus, it returns to white.
3. Detecting Mouse Click Inside Entry Widget
In this example, we bind the <Button-1>
event to detect when the user clicks inside the Entry widget.
import tkinter as tk
def on_mouse_click(event):
label.config(text="Entry Box Clicked!")
root = tk.Tk()
root.title("Entry Mouse Click Event - tutorialkart.com")
root.geometry("400x200")
entry = tk.Entry(root)
entry.pack(pady=10)
label = tk.Label(root, text="Click inside the Entry box")
label.pack(pady=10)
entry.bind("<Button-1>", on_mouse_click)
root.mainloop()
Output in Windows:
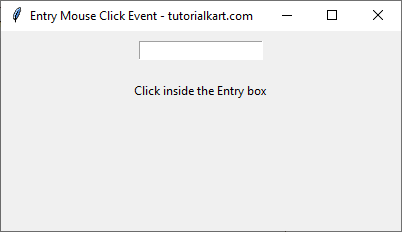
When the Entry widget is clicked, the label updates to “Entry Box Clicked!”.
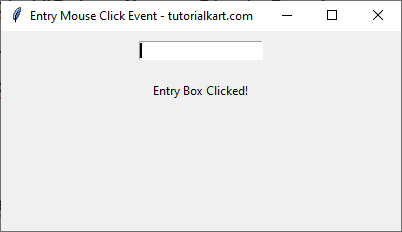
4. Detecting Enter Key Press in Entry Widget
In this example, we bind the <Return>
event to detect when the Enter key is pressed inside the Entry widget.
import tkinter as tk
def on_enter_pressed(event):
label.config(text=f"You entered: {entry.get()}")
root = tk.Tk()
root.title("Entry Return Key Event - tutorialkart.com")
root.geometry("400x200")
entry = tk.Entry(root)
entry.pack(pady=10)
label = tk.Label(root, text="Press Enter after typing")
label.pack(pady=10)
entry.bind("<Return>", on_enter_pressed)
root.mainloop()
Output in Windows:
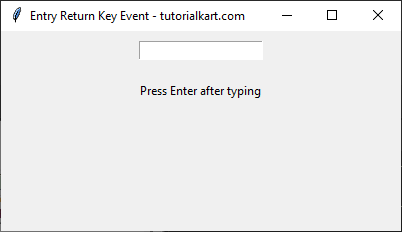
When the user types inside the Entry widget and presses Enter, the label updates to display the entered text.
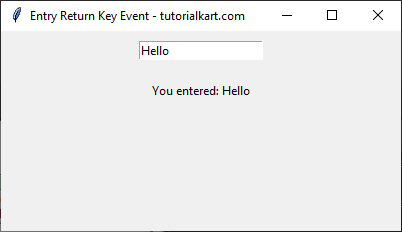
Conclusion
In this tutorial, we explored various events available for the Entry
widget in Tkinter and learned how to use them with examples. The key events include:
<KeyPress>
– Detects when a key is pressed inside the Entry widget.<FocusIn>
and<FocusOut>
– Detects when the Entry widget gains or loses focus.<Button-1>
– Detects when the user clicks inside the Entry widget.<Return>
– Detects when the Enter key is pressed.