React Array Loop
In React, rendering lists or arrays is a common task, especially when dealing with dynamic data. Using JavaScript’s map()
method, you can loop through arrays and generate elements for each item, making your UI dynamic and efficient.
How to Loop Through Arrays in React
React leverages JavaScript’s map()
function to iterate over arrays and create components or elements for each item in the array. The map()
method returns a new array of React elements which can then be rendered within your component.
Steps to Loop Through Arrays
- Create an array of data.
- Use the
map()
function to iterate over the array. - Return a React element for each item.
- Ensure each item has a unique
key
prop for optimal rendering performance.
Example 1: Rendering an Array of Strings as List
In this example, we take an array of strings, and display them as a list in UI.
App.js
import React from 'react';
function App() {
const fruits = ['Apple', 'Banana', 'Cherry', 'Date', 'Elderberry'];
return (
<div>
<h1>Fruit List</h1>
<ul>
{fruits.map((fruit, index) => (
<li key={index}>{fruit}</li>
))}
</ul>
</div>
);
}
export default App;
Output:
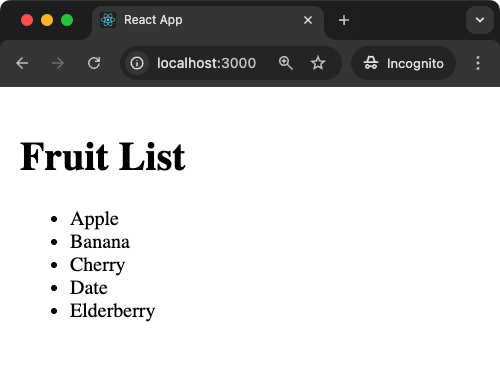
Explanation of the Example
- The
fruits
array contains a list of fruit names. - The
map()
function iterates over thefruits
array and returns an<li>
element for each fruit. - Each
<li>
element is given a uniquekey
prop based on the index of the array item. This helps React identify each list item and optimize rendering.
Example 2: Rendering an Array of Objects as a List
In this example, we take an array of objects, and display them as a list in UI, where each list item displays the information about the respective object.
App.js
import React from 'react';
function App() {
const users = [
{ id: 1, name: 'Alice', age: 25 },
{ id: 2, name: 'Bob', age: 30 },
{ id: 3, name: 'Charlie', age: 35 },
];
return (
<div>
<h1>User List</h1>
<ul>
{users.map((user) => (
<li key={user.id}>
{user.name} - {user.age} years old
</li>
))}
</ul>
</div>
);
}
export default App;
Output:
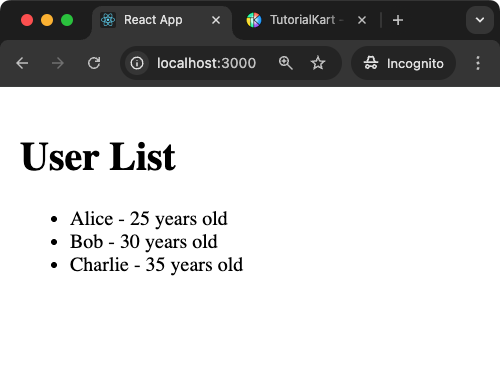
Explanation of the Example
- The
users
array contains objects representing user data, including anid
,name
, andage
. - The
map()
function iterates over theusers
array and returns an<li>
element for each user. - The
key
prop is set to the uniqueid
of each user to ensure optimal rendering.
Best Practices
- Always provide a unique
key
prop for each list item to help React efficiently manage DOM updates. - Use descriptive variable names when iterating over arrays to enhance code readability.
- Avoid using array indices as keys unless the array is static and will not change over time.
Example 3: Rendering an Array of Objects as a Table
In this example, we take an array of objects, and display them as a table in UI, where each table row displays the information about the respective object.
App.js
import React from 'react';
import './App.css'
function App() {
const users = [
{ id: 1, name: 'Alice', age: 25 },
{ id: 2, name: 'Bob', age: 30 },
{ id: 3, name: 'Charlie', age: 35 },
];
return (
<div>
<h1>User List</h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
{users.map((user) => (
<tr key={user.id}>
<td>{user.name}</td>
<td>{user.age}</td>
</tr>
))}
</table>
</div>
);
}
export default App;
App.css
table {
width: 100%;
border-collapse: collapse;
}
td, th {
border: 1px solid #ddd;
padding: 8px;
}
tr:nth-child(even){background-color: #f2f2f2;}
th {
padding-top: 12px;
padding-bottom: 12px;
text-align: left;
background-color: #0454aa;
color: white;
}
Output:
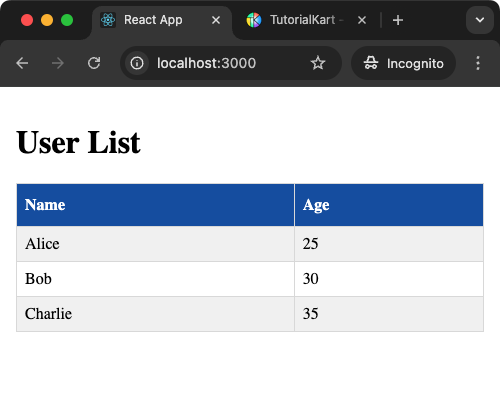
Conclusion
Using the map()
function in React makes it easy to loop through arrays and render lists dynamically. By following best practices and using unique keys, you can ensure optimal performance and maintainable code.