React Coin Flip App
The React Coin Flip App is an interactive project to practice React state management, event handling, and CSS animations. This app simulates a coin flip with a visually appealing animation and displays the result (Heads or Tails).
Features of the Coin Flip App
- Interactive button to flip the coin.
- CSS animations for the flipping effect.
- Dynamic result display: Heads or Tails.
- Visually appealing design with a circular coin representation.
Explanation of the Program
1. State Management
useState
is used to track the coin’s current face (“Heads” or “Tails”) and whether the animation is in progress. Refer React useState Hook.const [result, setResult] = useState('Heads');
: Stores the current result of the coin flip.const [flipping, setFlipping] = useState(false);
: Tracks whether the coin is currently flipping.
2. Event Handling
- The
flipCoin
function is triggered when the user clicks the “Flip Coin” button. - The function randomly determines “Heads” or “Tails” and updates the state after a brief animation.
Complete React Calculator Application
Create an empty React Application, and edit the App.js, and App.css files.
The following is the screenshot of project explorer in Visual Studio Code.
Explorer
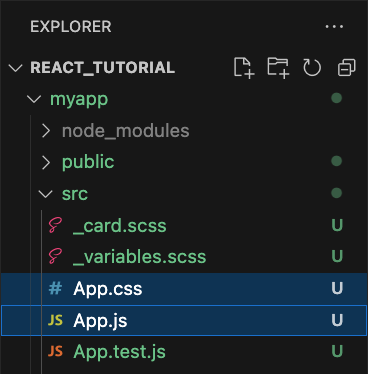
App.js
import React, { useState } from 'react';
import './App.css';
function CoinFlipApp() {
const [result, setResult] = useState('Heads');
const [flipping, setFlipping] = useState(false);
const flipCoin = () => {
if (flipping) return; // Prevent multiple flips
setFlipping(true);
setTimeout(() => {
const outcomes = ['Heads', 'Tails'];
const newResult = outcomes[Math.floor(Math.random() * outcomes.length)];
setResult(newResult);
setFlipping(false);
}, 1000); // Duration of the flip animation
};
return (
<div className="coin-flip-app">
<h1>React Coin Flip</h1>
<div className={`coin ${flipping ? 'flipping' : ''}`}>
<div className="coin-face">{result}</div>
</div>
<button onClick={flipCoin} disabled={flipping}>
{flipping ? 'Flipping...' : 'Flip Coin'}
</button>
</div>
);
}
export default CoinFlipApp;
App.css
.coin-flip-app {
font-family: Arial, sans-serif;
margin: 50px auto;
max-width: 300px;
text-align: center;
}
h1 {
color: #333;
margin-bottom: 20px;
}
.coin {
width: 200px;
height: 200px;
border-radius: 50%;
background-image: linear-gradient(to right, #3ab5b0 0%, #3d99be 31%, #56317a 100%);
display: flex;
align-items: center;
justify-content: center;
margin: 20px auto;
font-size: 24px;
font-weight: bold;
color: #fff;
transform: perspective(600px);
}
.coin.flipping {
animation: flip 0.95s forwards; /* Increased duration and set animation to loop */
}
@keyframes flip {
0% {transform: scale(1) rotateX(0deg);}
50% {transform: scale(1.5) rotateX(1800deg);}
100% {transform: scale(1) rotateX(3600deg);}
}
.coin-face {
display: flex;
align-items: center;
justify-content: center;
width: 100%;
height: 100%;
backface-visibility: hidden;
}
button {
padding: 10px 15px;
border: none;
border-radius: 4px;
background-color: #007bff;
color: white;
font-size: 16px;
cursor: pointer;
}
button:hover:not(:disabled) {
background-color: #0056b3;
}
button:disabled {
background-color: #ccc;
cursor: not-allowed;
}
Output
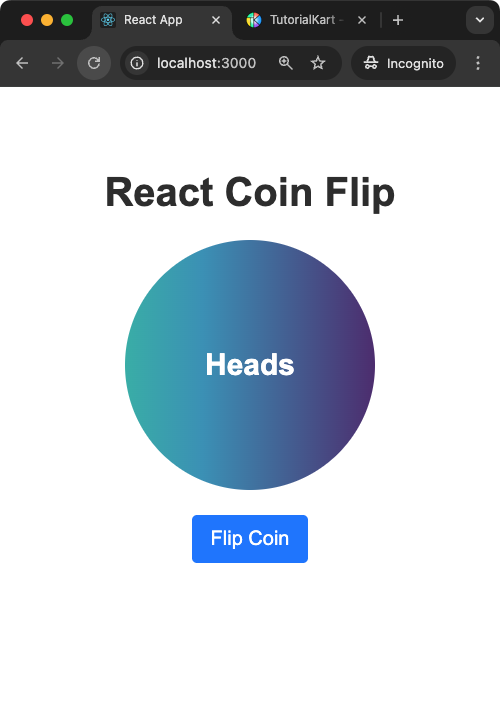
How It Works
- When the user clicks the “Flip Coin” button, the
flipCoin
function is called. - The coin enters a “flipping” animation state by applying a CSS class.
- After 1 second, the animation ends, and a random outcome (Heads or Tails) is displayed.
- The “Flip Coin” button is disabled during the animation to prevent multiple flips.
Reference:
- Coin Flip Online Tool by ConvertOnline.org.
Conclusion
The React Coin Flip App is a fun and interactive project that demonstrates key concepts of React, including state management, conditional rendering, and animations. It can be further enhanced with additional features, such as tracking the number of flips or displaying a history of outcomes. Experiment with the app to deepen your understanding of React!