What Are React Components?
React components are the building blocks of any React application. They encapsulate the UI and behavior of an element and can be reused throughout the app.
Types of React Components
- Functional Components: Simple JavaScript functions that return JSX.
- Class Components: ES6 classes that extend
React.Component
and include arender
method.
1 Functional Components
Functional components are the most common type of components in modern React applications. They are simple and allow the use of hooks.
App.js
import React from 'react';
function App() {
return (
<h2>Hello World</h2>
);
}
export default App;
Output
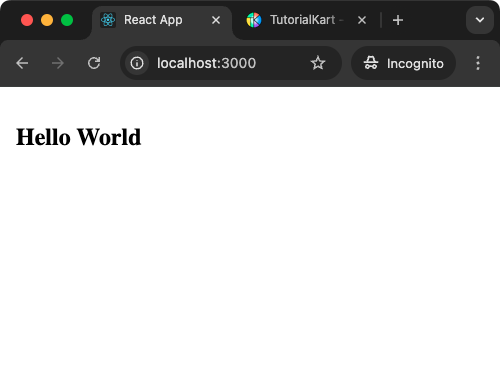
2 Class Components
Class components were the traditional way of creating components before hooks were introduced. They allow you to use lifecycle methods and state.
App.js
import React, { Component } from 'react';
class App extends Component {
render() {
return (
<h2>Hello World 2</h2>
);
}
}
export default App;
Output
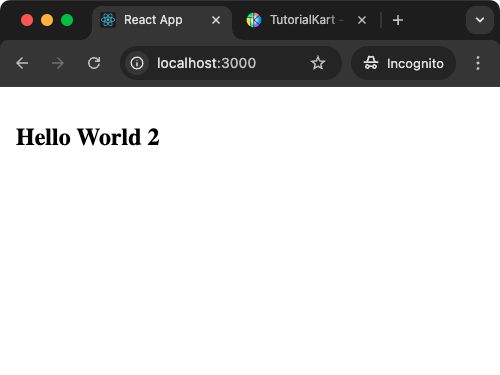
Props in Components
Props (short for “properties”) are used to pass data from one component to another. They are immutable and allow components to be dynamic.
App.js
import React from 'react';
function Greeting(props) {
return <h2>Hello, {props.name}!</h2>;
}
function App() {
return (
<div>
<Greeting name="Arjun" />
<Greeting name="Ram" />
</div>
);
}
export default App;
Output
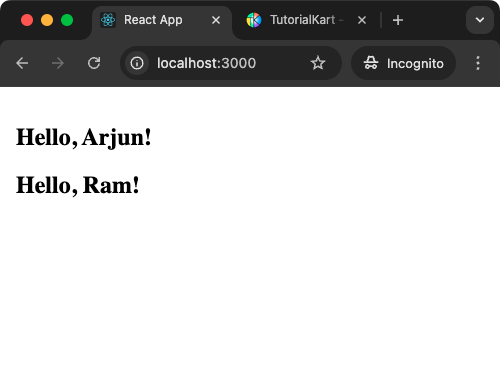
State in Class Components
State is an object that holds dynamic data within a class component. It is mutable and can be updated using setState
.
App.js
import React, { Component } from 'react';
class App extends Component {
constructor() {
super();
this.state = {
count: 0,
};
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<h2>Count: {this.state.count}</h2>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default App;
Output
Hooks in Functional Components
Hooks allow functional components to use state and lifecycle methods. The most common hooks are useState
and useEffect
.
App.js
import React, { useState } from 'react';
function App() {
const [count, setCount] = useState(0);
return (
<div>
<h2>Count: {count}</h2>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default App;
Output
Conclusion
React components are essential for building modular and reusable UI. Functional components with hooks are now the preferred approach due to their simplicity and power.