React CSS Styling
Styling in React can be achieved using various methods such as inline styles, CSS classes, CSS modules, or styled-components. Each approach has its own advantages and use cases.
In this tutorial, we will explore the most common methods to style React components with detailed examples.
1 Inline Styles for CSS Styling
Inline styles are defined directly within the component as a JavaScript object. This method is simple and ensures that the styles are scoped to the specific element.
App.js
import React from 'react';
function InlineStyling() {
const headingStyle = {
color: 'blue',
fontSize: '24px',
textAlign: 'center'
};
return <h2 style={headingStyle}>This is styled using inline styles!</h2>;
}
export default InlineStyling;
Explanation:
headingStyle
: A JavaScript object containing CSS properties and their values.style
: The property used in JSX to apply the inline styles.- This approach is useful for simple, component-specific styles.
Output:
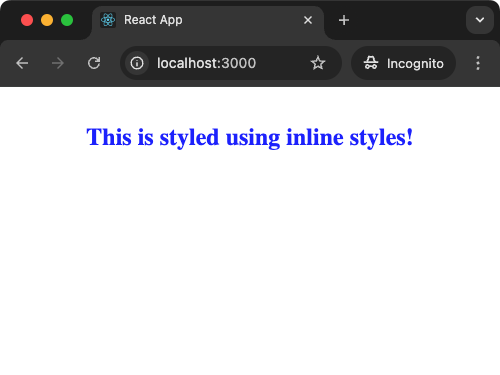
2 CSS Classes for Styling
Using CSS classes is the most common way to style components. The styles are defined in an external CSS file, and the file is specified in the JavaScript file using an import
statement. The styles are then applied using the className
attribute in JSX.
App.css
.title {
color: green;
font-size: 28px;
text-align: center;
}
App.js
import React from 'react';
import './App.css'; // Importing the CSS file
function CSSClasses() {
return <h2 className="title">This is styled using CSS classes!</h2>;
}
export default CSSClasses;
Explanation:
App.css
: The CSS file where styles are defined and stored.import './App.css';
: Specifies the CSS file in the JavaScript file to apply the defined styles to components.className
: The attribute used in JSX to apply a CSS class to an element.- This approach is ideal for global styles or when sharing styles across multiple components.
Output:
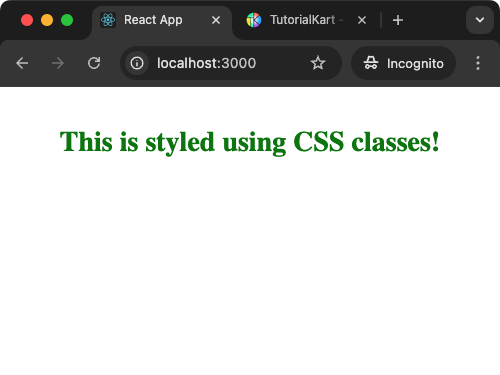
3 CSS Modules for Styling
CSS Modules allow for scoped styling by creating locally scoped CSS files. This prevents styles from leaking across components and ensures better maintainability.
Below is the screenshot of file package explorer containing App.js and Header.module.css in the /src folder. The following are contents of the respective files.
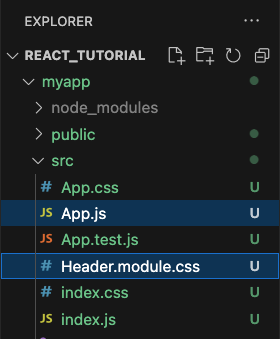
Header.module.css
.header {
color: red;
font-size: 30px;
text-align: center;
}
App.js
import React from 'react';
import headerStyles from './Header.module.css';
function CSSModules() {
return <h2 className={headerStyles.header}>This is styled using CSS Modules!</h2>;
}
export default CSSModules;
Explanation:
Header.module.css
: A CSS module file with scoped styles, and we are importing this asheaderStyles
in the import section.headerStyles.header
in the JSXclassName={headerStyles.header}
generates a dynamic class name unique to this module.- This approach is ideal for large applications where style conflicts are a concern.
Output:
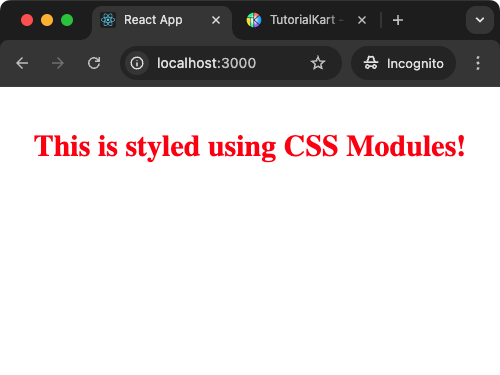
4 Styled-Components
styled-components is a popular library for writing CSS-in-JS. It allows you to define styles directly within your components as tagged template literals, resulting in dynamic, component-scoped styles.
If you have not installed this styled-components library, you may run the following npm command.
npm install styled-components
Or if you are using yarn, run the following command.
yarn add styled-components
App.js
import React from 'react';
import styled from 'styled-components';
const StyledHeading = styled.h2`
color: purple;
font-size: 32px;
text-align: center;
`;
function StyledComponents() {
return <StyledHeading>This is styled using styled-components!</StyledHeading>;
}
export default StyledComponents;
Explanation:
- We have imported
styled
from thestyled-components
library in the import section. styled.h2
: Creates a styled version of the<h2>
tag.- Tagged Template Literals: Define CSS directly within the JavaScript file.
- This approach is great for dynamic styling and maintaining styles within the component file.
Output:
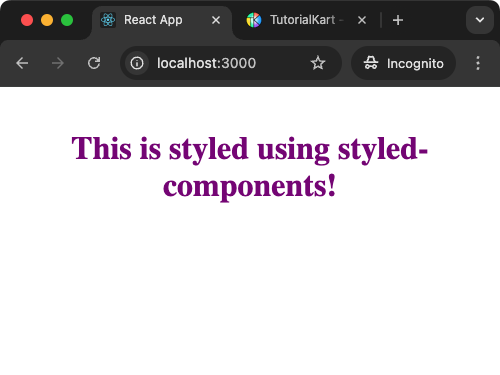
5 Conditional Styling
Conditional styling allows you to dynamically change styles based on props or state. This example uses inline styles for conditional rendering based on a button’s state.
App.js
import React, { useState } from 'react';
function ConditionalStyling() {
const [isActive, setIsActive] = useState(false);
const buttonStyle = {
backgroundColor: isActive ? 'green' : 'gray',
color: 'white',
padding: '10px 20px',
border: 'none',
borderRadius: '5px',
cursor: 'pointer'
};
return (
<button
style={buttonStyle}
onClick={() => setIsActive(!isActive)}
>
{isActive ? 'Active' : 'Inactive'}
</button>
);
}
export default ConditionalStyling;
Explanation:
isActive
: A state variable toggled by the button click.buttonStyle
: Dynamically updates the style object based on the state. Specifically,backgroundColor
is dynamically set to green or grey color based on theisActive
using JavaScript Ternary Operator.- This approach is useful for interactive elements where styles change based on user actions.
Output:
Summary Table for CSS Styling Methods
Here’s a summary table highlighting where each React CSS styling method is commonly used and its benefits:
Styling Method | Use Cases | Advantages | Disadvantages |
---|---|---|---|
Inline Styles | – Quick, small styles for individual components | – Scoped to the component – Easy to use for dynamic styling | – Limited CSS features (e.g., pseudo-classes) – Can become verbose |
CSS Classes | – Global styles – Shared styles across multiple components | – Reusable and easy to maintain – Familiar for most developers | – Potential for style conflicts |
CSS Modules | – Scoped styles for individual components – Large applications | – Prevents style conflicts – Easy to manage in modular projects | – Slightly more complex setup than regular CSS |
Styled-Components | – Dynamic styles – Component-level styling – Modern CSS-in-JS approach | – Fully scoped styles – Supports dynamic and conditional styling – Great for large apps | – Additional library required – Slight learning curve for beginners |
Conditional Styling | – Styling that changes based on state or props | – Highly dynamic and responsive – Ideal for interactive elements | – Can become complex if overused |
Conclusion
React offers a variety of styling methods to suit different needs. Whether you prefer inline styles for simplicity, CSS modules for scoped styles, or libraries like styled-components for dynamic styling, React provides the flexibility to implement and manage styles effectively. Experiment with these methods to find the best fit for your projects!