React Error Boundaries
Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of crashing the entire application. They are implemented using React’s class components with lifecycle methods like getDerivedStateFromError
and componentDidCatch
.
How to Implement Error Boundaries in a React Application
To implement error boundaries, you need to follow these steps:
- Create a class component that implements the
getDerivedStateFromError
andcomponentDidCatch
methods. - Wrap the error-prone components within this boundary component.
- Provide a fallback UI for displaying errors to the user.
Example: Implementing Error Boundaries
- Create a new class component called
ErrorBoundary
. - Define the
getDerivedStateFromError
lifecycle method to update the state when an error occurs. - Use the
componentDidCatch
method to log the error information. - Render a fallback UI if an error is detected.
File Explorer:
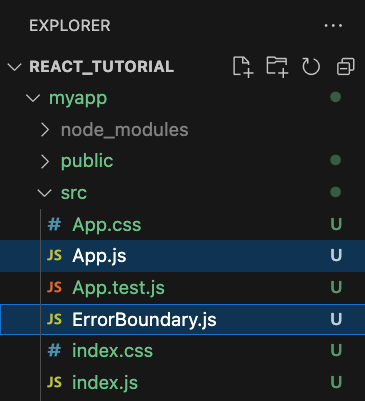
ErrorBoundary.js
</>
Copy
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render shows the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// Log the error to an error reporting service
console.error("Error caught by ErrorBoundary: ", error, errorInfo);
}
render() {
if (this.state.hasError) {
// Render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
App.js
Wrap the components that may throw errors within the ErrorBoundary
component.
</>
Copy
import React from 'react';
import ErrorBoundary from './ErrorBoundary';
function ProblematicComponent() {
throw new Error("I crashed!");
return <div>This will not render.</div>;
}
function App() {
return (
<div>
<h1>React Error Boundaries Example</h1>
<ErrorBoundary>
<ProblematicComponent />
</ErrorBoundary>
</div>
);
}
export default App;
Output:
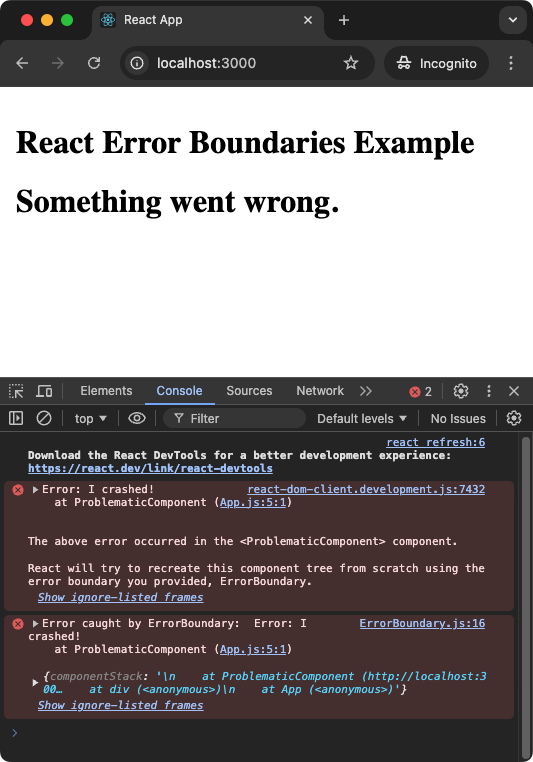
Explanation of the Example
- The
ErrorBoundary
component catches errors thrown by its child components and displays a fallback UI (“Something went wrong”). - The
getDerivedStateFromError
method updates the state to indicate that an error has occurred. - The
componentDidCatch
method logs error details for debugging or reporting purposes. - The
ProblematicComponent
simulates an error to demonstrate the functionality of the error boundary.
Important Notes
- Error boundaries only catch errors in the component tree below them. They do not catch errors in event handlers, asynchronous code, or server-side rendering.
- For event handler errors, use
try-catch
blocks within the handler. - Only class components can be error boundaries. Functional components cannot implement error boundaries as of React 18.
Conclusion
Error boundaries are essential for building resilient React applications by ensuring that errors do not crash the entire application. Implementing them helps improve the user experience and debugging process.