React Events
Handling events in React is similar to handling events in plain JavaScript, but with some syntax differences. React events are written in camelCase (e.g., onClick
instead of onclick
) and are passed as functions instead of strings. React also ensures event handling is consistent across all browsers.
1 Handling Button Click
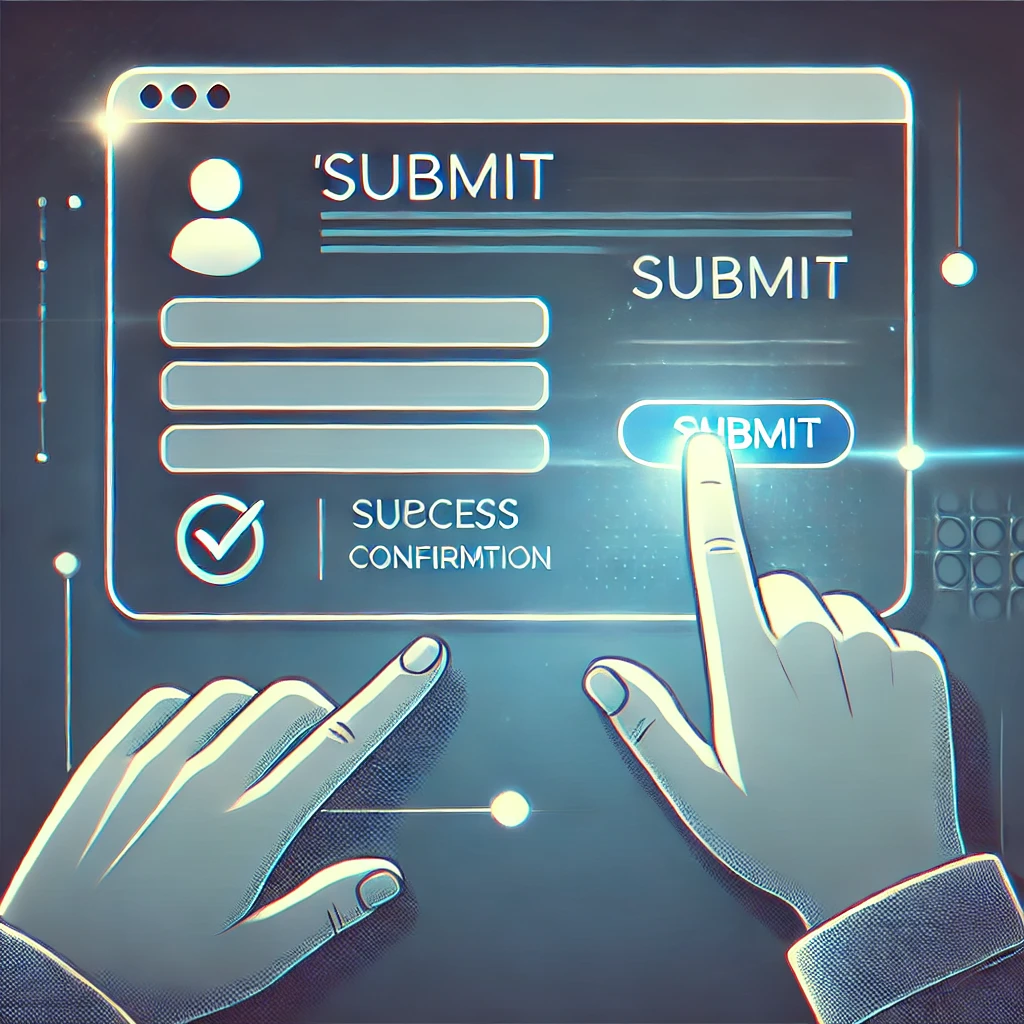
Buttons are often used to trigger actions like submitting a form, updating state, or performing calculations. React simplifies this process by allowing you to attach event handlers to buttons using the onClick
attribute.
<button onClick={handleClick}>Click Me</button>
These event handlers can be functions that define what happens when the button is clicked.
const handleClick = () => {
setCount(count + 1);
};
Example
In this example, we demonstrate how to handle a simple button click event in React. The event triggers a function that updates the state.
App.js
import React, { useState } from 'react';
function ButtonClick() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<p>You clicked {count} times.</p>
<button onClick={handleClick}>Click Me</button>
</div>
);
}
export default ButtonClick;
Explanation:
onClick
: Attaches the event handler to the button.handleClick
: Increments the state variablecount
when the button is clicked.- This approach is straightforward and demonstrates how to bind event handlers in React.
Output:
2 Passing Arguments to Event Handlers
Passing arguments to event handlers is a common requirement, especially when dealing with dynamic data or multiple elements. React allows you to pass arguments using inline arrow functions or by binding arguments explicitly.
Example
In this example, we will see how to pass parameters to a function using an inline arrow function.
App.js
import React from 'react';
function Greet() {
const sayHello = (name) => {
alert(`Hello, ${name}!`);
};
return (
<div>
<button onClick={() => sayHello('Alice')}>Greet Alice</button>
<button onClick={() => sayHello('Bob')}>Greet Bob</button>
</div>
);
}
export default Greet;
Explanation:
- Inline Arrow Function: Allows you to pass arguments to the event handler. For example,
() => sayHello('Alice')
is an inline function. - The
sayHello
function displays a personalised greeting in an alert box. - This approach is useful for dynamic content or when the handler requires specific parameters.
Output:
3 Handling Input Change
Input fields, such as text boxes, checkboxes, and dropdowns, require user interactions to capture and process data. React simplifies this with the onChange
event, which allows you to update the component’s state as the user types or interacts with the input field. This approach ensures that the input value stays in sync with the state, enabling controlled components for better data handling and validation.
Example
In this example, we demonstrate how to handle user input changes in a text field. The input value is stored in the component state and updated dynamically.
App.js
import React, { useState } from 'react';
function InputChange() {
const [value, setValue] = useState('');
const handleChange = (e) => {
setValue(e.target.value);
};
return (
<div>
<input type="text" value={value} onChange={handleChange} placeholder="Type something..." />
<p>You typed: {value}</p>
</div>
);
}
export default InputChange;
Explanation:
onChange
: Triggers thehandleChange
function whenever the input value changes.setValue
: Updates the state with the current value of the input field.- This approach is essential for creating controlled components in forms.
Output:
4 Handling Form Submission
In React, form submission events are managed using the onSubmit
attribute.
<form onSubmit={handleSubmit}>
This allows you to control what happens when a form is submitted, such as validating input data, preventing the default browser behaviour, and processing or sending the data to a server.
Example
This example shows how to handle a form submission event and prevent the default behaviour. The form data is processed and displayed in an alert.
App.js
import React, { useState } from 'react';
function FormSubmit() {
const [name, setName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
alert(`Form submitted with name: ${name}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={name} onChange={(e) => setName(e.target.value)} />
</label>
<button type="submit">Submit</button>
</form>
);
}
export default FormSubmit;
Explanation:
onSubmit
: Attaches an event handler to the form’s submit event.e.preventDefault()
: Prevents the default form submission behavior (e.g., page reload).- This approach is commonly used to handle and validate form data before processing.
Output:
5 Handling Mouse Events
React can handle mouse events using onMouseEnter
, onMouseLeave
, onClick
, and more, which can be used to trigger dynamic UI updates or execute specific logic based on user actions.
Example
In this example, we handle mouse events such as hovering over an element. A message is displayed dynamically based on mouse interactions.
App.js
import React, { useState } from 'react';
function MouseEvents() {
const [message, setMessage] = useState('Hover over the box');
const handleMouseEnter = () => {
setMessage('Mouse is inside!');
};
const handleMouseLeave = () => {
setMessage('Mouse left the box');
};
return (
<div>
<div
onMouseEnter={handleMouseEnter}
onMouseLeave={handleMouseLeave}
style={{ width: '100px', height: '100px', backgroundColor: 'lightblue', textAlign: 'center', lineHeight: '100px' }}
>
Hover Me
</div>
<p>{message}</p>
</div>
);
}
export default MouseEvents;
Explanation:
onMouseEnter
andonMouseLeave
: Handle mouse hover events for the box.setMessage
: Dynamically updates the message based on mouse interactions.- This approach is useful for creating interactive UI elements.
Output:
Conclusion
React provides a robust event-handling system that simplifies handling user interactions. By understanding how to handle events like clicks, input changes, form submissions, and mouse interactions, you can build dynamic and interactive React applications. Practice these examples to master event handling in React.