React Forms
Handling forms is an essential part of building web applications, and React makes it straightforward with its controlled components and state management. React forms allow you to manage user input effectively and validate data dynamically.
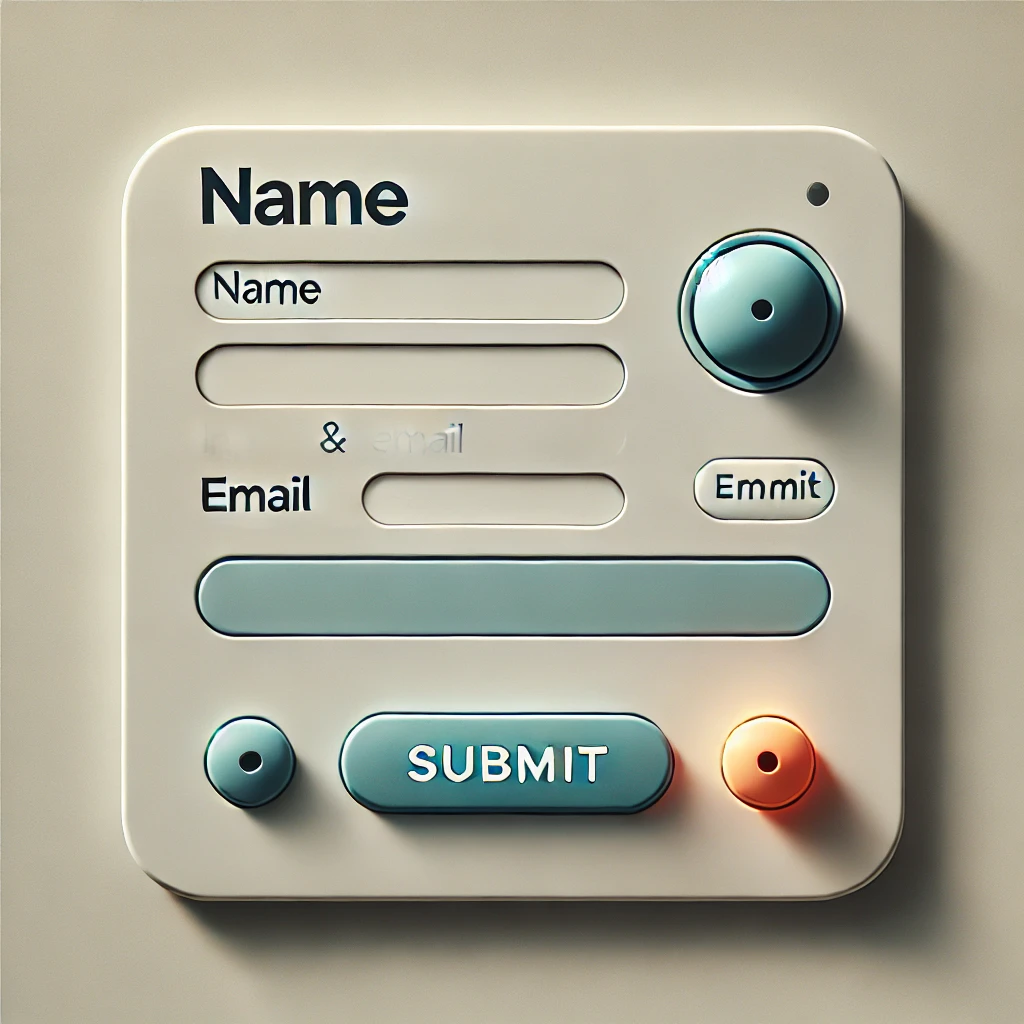
1. Example: Controlled Components
In this example, we use a controlled component where the form input’s value is managed by React state. This ensures that the input value is always in sync with the state.
App.js
import React, { useState } from 'react';
function ControlledForm() {
const [name, setName] = useState('');
const handleChange = (e) => {
setName(e.target.value);
};
const handleSubmit = (e) => {
e.preventDefault();
alert(`Name submitted: ${name}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={name} onChange={handleChange} />
</label>
<button type="submit">Submit</button>
</form>
);
}
export default ControlledForm;
Explanation:
- The
value
prop ensures the input field is controlled by thename
state. onChange
: Updates the state whenever the user types into the input field.- Submitting the form triggers the
handleSubmit
function, which processes the input data.
Output:
2. Example: Multiple Inputs
This example demonstrates how to handle multiple input fields in a form using a single state object. This approach is efficient for forms with multiple fields.
App.js
import React, { useState } from 'react';
function MultiInputForm() {
const [formData, setFormData] = useState({ name: '', email: '' });
const handleChange = (e) => {
const { name, value } = e.target;
setFormData({ ...formData, [name]: value });
};
const handleSubmit = (e) => {
e.preventDefault();
alert(`Form submitted: ${JSON.stringify(formData)}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
</label>
<br />
<label>
Email:
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
</label>
<br />
<button type="submit">Submit</button>
</form>
);
}
export default MultiInputForm;
Explanation:
formData
: A single state object stores values for all form fields.handleChange
: Dynamically updates the corresponding field in the state based on thename
attribute of the input field.- This approach reduces redundancy and simplifies state management for larger forms.
Output:
3. Example: Checkbox Handling
In this example, we handle checkbox inputs to track selected options. This is common in forms where users can select multiple options.
App.js
import React, { useState } from 'react';
function CheckboxForm() {
const [selectedOptions, setSelectedOptions] = useState([]);
const handleChange = (e) => {
const { value, checked } = e.target;
if (checked) {
setSelectedOptions([...selectedOptions, value]);
} else {
setSelectedOptions(selectedOptions.filter((option) => option !== value));
}
};
const handleSubmit = (e) => {
e.preventDefault();
alert(`Selected options: ${selectedOptions.join(', ')}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
<input
type="checkbox"
value="Option 1"
onChange={handleChange}
/>
Option 1
</label>
<br />
<label>
<input
type="checkbox"
value="Option 2"
onChange={handleChange}
/>
Option 2
</label>
<br />
<label>
<input
type="checkbox"
value="Option 3"
onChange={handleChange}
/>
Option 3
</label>
<br />
<button type="submit">Submit</button>
</form>
);
}
export default CheckboxForm;
Explanation:
selectedOptions
: Tracks the values of the selected checkboxes.handleChange
: Adds or removes values from the state based on whether the checkbox is checked or unchecked.- This approach is scalable for forms with multiple selectable options.
Output:
4. Example: Form Validation
In this example, we implement basic form validation to ensure the user submits valid data. Validation checks are performed before processing the form submission.
App.js
import React, { useState } from 'react';
function ValidationForm() {
const [email, setEmail] = useState('');
const [error, setError] = useState('');
const handleChange = (e) => {
setEmail(e.target.value);
};
const handleSubmit = (e) => {
e.preventDefault();
if (!email.includes('@')) {
setError('Please enter a valid email address.');
} else {
setError('');
alert(`Email submitted: ${email}`);
}
};
return (
<form onSubmit={handleSubmit}>
<label>
Email:
<input type="email" value={email} onChange={handleChange} />
</label>
<br />
{error && <p style={{ color: 'red' }}>{error}</p>}
<button type="submit">Submit</button>
</form>
);
}
export default ValidationForm;
Explanation:
error
: Stores error messages for validation feedback.handleSubmit
: Checks if the input value is valid before processing the form submission.- Dynamic validation improves user experience by providing immediate feedback.
Output:
Conclusion
React provides a flexible framework for handling forms with controlled components, state management, and validation. Mastering these techniques enables you to build dynamic and user-friendly forms for your applications.