React Function Components
Function components are one of the fundamental ways to create components in React. They are simpler than class components and have become the preferred approach, especially with the introduction of React Hooks.
1 What Are Function Components?
A function component is a simple JavaScript function that returns JSX, which is the syntax used to describe what the UI should look like. Unlike class components, function components do not require extending React.Component
and do not include lifecycle methods by default.
The following is a basic function component that returns 'Hello, World!'
Heading 2.
App.js
import React from 'react';
function Greeting() {
return <h2>Hello, World!</h2>;
}
export default Greeting;
Explanation:
function Greeting()
: A JavaScript function that returns JSX.return <h2>Hello, React!</h2>
: Specifies what the component should render.- Use
export default
to make the component reusable in other files.
Output
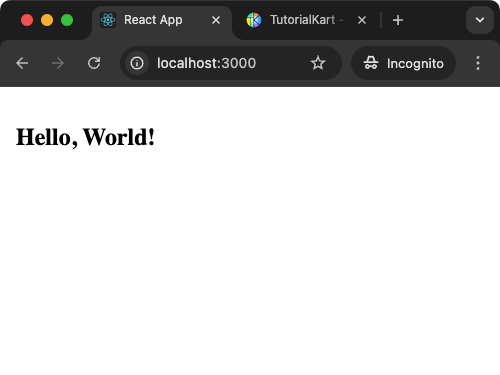
2 Using Props in Function Components
Props (short for properties) are used to pass data from parent components to child components. They allow function components to be dynamic and reusable.
The following is an example, where we have two function components: Greeting
and App
. Greeting
is the child component and App
is the parent component, and we pass properties from App
to Greeting
.
App.js
import React from 'react';
function Greeting(props) {
return <h2>Hello, {props.name}!</h2>;
}
function App() {
return (
<div>
<Greeting name="Arjun" />
<Greeting name="Ram" />
</div>
);
}
export default App;
Explanation:
props
: An object containing data passed from the parent component.<Greeting name="Arjun" />
: Passes thename
prop with a value of"Arjun"
.- The
Greeting
component uses{props.name}
to dynamically render the passed value.
Output:
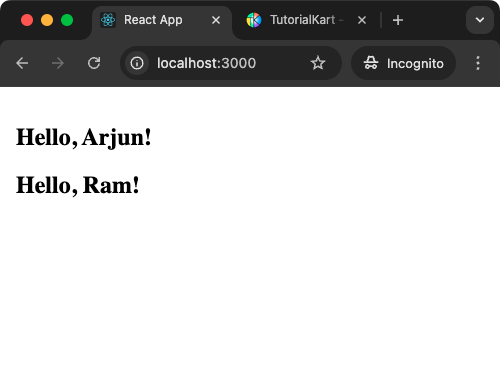
3 Managing State with Hooks
Function components can manage state using the useState
hook. This allows the component to update and re-render based on changes in its state.
Example: Counter Component
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h2>Count: {count}</h2>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
Explanation:
useState(0)
: Initializes the state with a value of0
.setCount
: A function that updates the state.- Each click on the button increments the state by
1
and re-renders the component with the new value.
Output Video:
4 Lifecycle with useEffect
The useEffect
hook enables function components to perform side effects, such as data fetching or subscriptions, that were previously handled using lifecycle methods in class components.
Example: Timer Component
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(seconds => seconds + 1);
}, 1000);
return () => clearInterval(interval); // Cleanup interval on unmount
}, []);
return <h2>Elapsed Time: {seconds} seconds</h2>;
}
export default Timer;
Explanation:
useEffect
: Sets up a timer when the component mounts.- The cleanup function ensures the timer is cleared when the component unmounts.
- An empty dependency array (
[]
) ensures the effect runs only once.
Output Video:
5 Event Handling
Event handling in function components is straightforward and uses JavaScript functions directly.
Example: Button Click
import React from 'react';
function ClickHandler() {
const handleClick = () => {
alert('Button clicked!');
};
return <button onClick={handleClick}>Click Me</button>;
}
export default ClickHandler;
Explanation:
onClick
: Attaches the event handler to the button.handleClick
: A function that executes when the button is clicked.- In this example, clicking the button triggers an alert with the message
"Button clicked!"
.
6 Styling Function Components
You can apply styles to function components using inline styles, CSS classes, or external stylesheets.
Example: Styling with Inline Styles
import React from 'react';
function StyledComponent() {
const styles = {
color: 'blue',
fontSize: '20px',
margin: '10px',
};
return <h2 style={styles}>This is styled text!</h2>;
}
export default StyledComponent;
Explanation:
styles
: An object containing CSS properties and values.- The
style
attribute is used to apply inline styles.
Output:
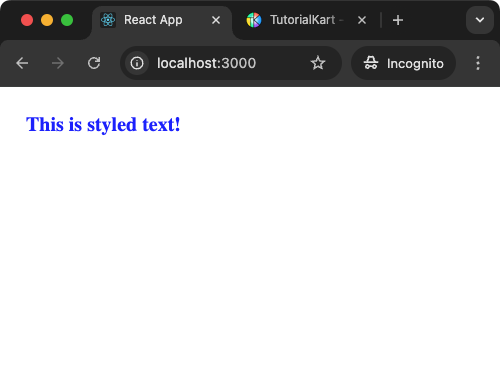
Conclusion
React function components are a simple yet powerful way to build reusable and dynamic UI elements. With the addition of Hooks, function components can handle state, lifecycle events, and more, making them a preferred choice for modern React development.