React Hooks
React Hooks are functions that allow you to use state and other React features in functional components. Introduced in React 16.8, they eliminate the need for class components and make function components more powerful.
1 useState Hook
The useState
hook lets you add state to functional components. It returns an array with two elements: the current state and a function to update it.
App.js
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // Initialize state
return (
<div>
<h2>Count: {count}</h2>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
Explanation:
useState(0)
: Initializes the state with a value of0
.- The value returned by
useState()
is stored in[count, setCount]
. Therefore,count
holds the current state, andsetCount
is the function to update it. - We are calling the
setCount
function to update thecount
tocount+1
, when the button is clicked. We pass the new valuecount+1
as argument to thesetCount
function. - The component re-renders whenever the state changes.
Output Video:
2 useEffect Hook
The useEffect
hook allows you to perform side effects in functional components, such as data fetching, subscriptions, or directly updating the DOM.
App.js
import React, { useState, useEffect } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(seconds => seconds + 1);
}, 1000);
return () => clearInterval(interval); // Cleanup on unmount
}, []); // Empty array ensures it runs only once
return <h2>Elapsed Time: {seconds} seconds</h2>;
}
export default Timer;
Explanation:
- The
useEffect
hook sets up a timer usingsetInterval
. - The cleanup function ensures the timer is cleared when the component unmounts.
- An empty dependency array (
[]
) ensures the effect runs only once.
Output Video:
3 useContext Hook
The useContext
hook provides an easy way to consume context values without needing to wrap components in a Consumer
.
App.js
import React, { createContext, useContext } from 'react';
const UserContext = createContext();
function UserProfile() {
const user = useContext(UserContext);
return <h2>User: {user}</h2>;
}
function App() {
return (
<UserContext.Provider value="Alice">
<UserProfile />
</UserContext.Provider>
);
}
export default App;
Explanation:
createContext
: Creates a context for the user.useContext
: Consumes the context value without needing a wrapper component.- In this example, the
UserProfile
component dynamically displays the user’s name.
Output:
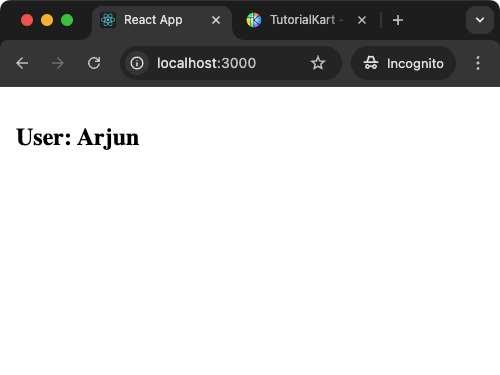
4 useRef Hook
The useRef
hook creates a mutable reference that persists across renders. It’s often used for accessing DOM elements or storing mutable values.
App.js
import React, { useRef, useState } from 'react';
function ReadInput() {
const inputRef = useRef();
const [value, setValue] = useState('');
const handleRead = () => {
setValue(inputRef.current.value); // Read the input value
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={handleRead}>Read Input</button>
<p>You entered: {value}</p>
</div>
);
}
export default ReadInput;
Explanation:
useRef
: Creates a reference to the input element.inputRef.current.focus()
: Directly interacts with the input element to set focus.
Output:
5 useReducer Hook
The useReducer
hook is useful for managing complex state logic in functional components. It is an alternative to useState
.
App.js
import React, { useReducer } from 'react';
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<div>
<h2>Count: {state.count}</h2>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
}
export default Counter;
Explanation:
reducer
: A function that determines state changes based on the action.useReducer
: Manages the state and dispatches actions.- In this example, the counter is incremented or decremented based on the dispatched action.
Output:
Conclusion
React Hooks make functional components more powerful by providing features like state, side effects, context, and more. Start practicing these hooks to build clean and efficient React applications.