React – How to Access Public Folder
In a React application, the public
folder is a special directory used for storing static assets such as images, videos, and files. These assets are served directly by the web server without being processed by the build tool, making them accessible using relative URLs.
In this tutorial, we will learn how to access the public folder, and use the assets in the public folder.
Why Use the Public Folder?
- To include static assets that do not require processing by Webpack or other bundlers.
- To serve files that are referenced globally, such as
favicon.ico
, robots.txt, or large media files.
How to Access Files in the Public Folder
Files in the public
folder are accessible via a relative path starting from the root URL (e.g., /
). You can reference these files directly in your JSX or HTML.
Example: Accessing an Image in the Public Folder
In this example, we demonstrate how to use an image stored in the public
folder and display it in a React component. This method is ideal for assets that do not change during the application’s lifecycle.
Project Structure:
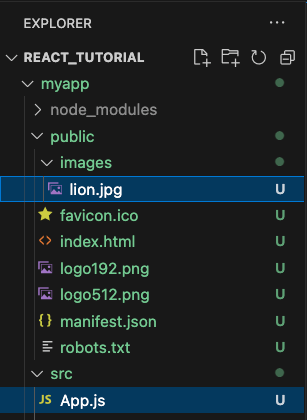
App.js
In this example, we reference the image using a relative path from the public
folder.
import React from 'react';
function App() {
return (
<div>
<h1>Accessing Public Folder Example</h1>
<img src="/images/lion.jpg" alt="Lion Closeup Image" />
</div>
);
}
export default App;
Output
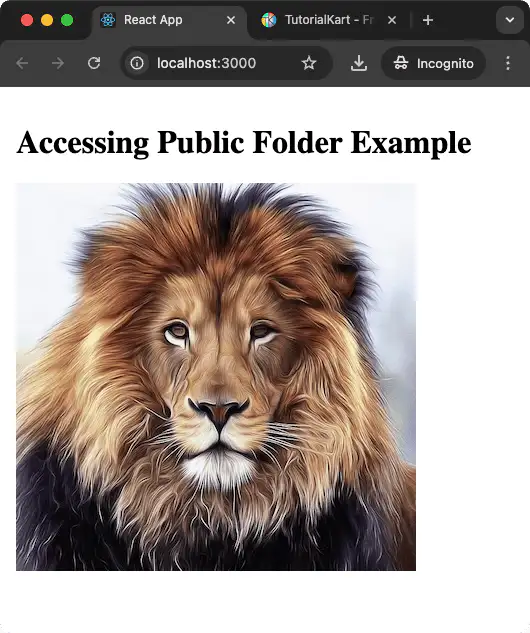
Explanation
- The
src
attribute of the<img>
tag references the image using a relative path starting from thepublic
folder. - The
/images/lion.jpg
URL directly serves the image without being processed by React’s build tools. - This method is useful for static assets that do not need to be bundled with the application.
Best Practices
- Use the
public
folder for assets that do not require dynamic imports or processing. - Avoid overusing the
public
folder for application-specific assets; prefersrc
for modularity. - Always provide an
alt
attribute for images to improve accessibility and SEO.
Conclusion
The public
folder in React is a convenient place for storing and serving static files. By referencing these files directly, you can efficiently include global assets in your application.