React – How to Add Multiple Class Names
In React, managing multiple class names for an element is used when we need to dynamically style components. We can add multiple class names to an element based on conditions, or just like that.
In this tutorial, we will learn about different methods to add multiple class names for an element, with examples.
Ways to Add Multiple Class Names
Here are different approaches to apply multiple class names to an element:
- String concatenation.
- Template literals.
- Using the
classnames
library. - Conditional logic with JavaScript.
Method 1: Using String Concatenation
In this example, multiple class names are concatenated into a single string using the +
operator or array join.
App.css
.button {
padding: 10px 20px;
}
.primary {
background-color: lightskyblue;
}
.secondary {
background-color: lightpink;
}
App.js
import React from 'react';
import './App.css';
function App() {
const baseClass = "button";
const additionalClass = "primary";
return (
<div>
<button className={baseClass + " " + additionalClass}>Click Me</button>
</div>
);
}
export default App;
Output
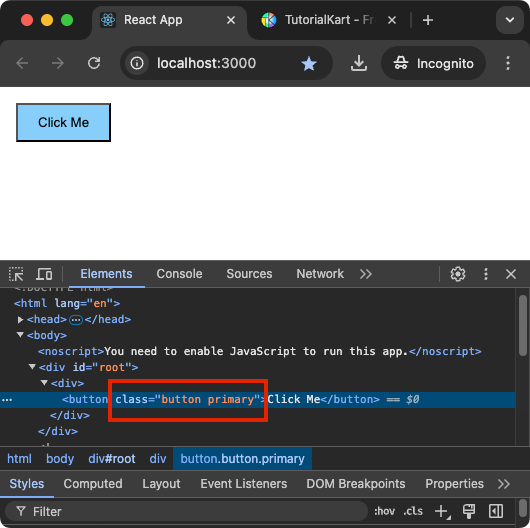
Method 2: Using Template Literals
This example demonstrates how to use JavaScript template literals to dynamically add multiple class names.
App.css
.button {
padding: 10px 20px;
}
.active {
background-color: lightgreen;
}
.inactive {
background-color: gray;
}
App.js
import React from 'react';
import './App.css';
function App() {
const isActive = true;
const baseClass = "button";
const dynamicClass = isActive ? "active" : "inactive";
return (
<div>
<button className={`${baseClass} ${dynamicClass}`}>Click Me</button>
</div>
);
}
export default App;
Output

Method 3: Using the classnames
Library
The classnames
library simplifies the process of managing multiple class names, especially with conditional logic.
Installation:
To install the classnames library, Open a Terminal or Command Prompt, and run the following command.
npm install classnames
In the following example, we use class names library to add multiple classnames to a button element.
App.css
.button {
padding: 10px 20px;
}
.active {
background-color: lightgreen;
}
.inactive {
background-color: gray;
}
App.js
import React from 'react';
import classNames from 'classnames';
import './App.css';
function App() {
const isActive = true;
const buttonClass = classNames("button", { active: isActive, disabled: !isActive });
return (
<div>
<button className={buttonClass}>Click Me</button>
</div>
);
}
export default App;
Output

Summary of All Methods to Use Multiple Class Names
- String Concatenation: Manually concatenates class names using
+
. - Template Literals: Uses JavaScript template literals for cleaner syntax when combining class names.
- classnames Library: Dynamically constructs class names based on conditions, making it more readable and manageable for complex scenarios.
Best Practices
- Use the
classnames
library for better readability and scalability. - Always ensure class names are meaningful and follow a consistent naming convention.
- Avoid hardcoding multiple class names in JSX if they are conditionally applied.
Conclusion
Adding multiple class names in React is flexible and can be achieved using various methods, including string concatenation, template literals, and libraries like classnames
. Choosing the right method depends on the complexity of your styling logic and the scalability of your application.