React – How to Export Multiple Components
React is a popular JavaScript library for building user interfaces. In React, components are the building blocks of an application. A React application often consists of multiple components, and developers may need to share or reuse these components across various files. To achieve this, React allows exporting and importing components efficiently. This tutorial will guide you through the process of exporting multiple components in React with clear steps, examples, and explanations.
By the end of this tutorial, you will understand:
- The difference between default and named exports
- How to export multiple components from a single file
- Best practices for organizing your React components
Steps to Export Multiple Components in React
Create Your Components:Define the components you want to export in a single file or separate files.
Export the Components: Use export
(for named exports) or export default
(for default exports) to make your components reusable in other files.
Import the Components: Use the import
statement in the target file to bring the components into scope.
Examples
Example 1: Exporting Multiple Components Using Named Exports
In this example, we define two components, Header
and Footer
, in a single file and export them using named exports.
Filename: components.js
import React from 'react';
export const Header = () => {
return <header><h1>Welcome to My Website</h1></header>;
};
export const Footer = () => {
return <footer><p>© 2024 My Website</p></footer>;
};
Explanation: Here, we use the export
keyword before each component declaration. This allows us to export multiple components from a single file using named exports.
Filename: App.js
import React from 'react';
import { Header, Footer } from './components';
const App = () => {
return (
<div>
<Header />
<p>This is the main content of the app.</p>
<Footer />
</div>
);
};
export default App;
Explanation: In this file, we import the Header
and Footer
components using their names inside curly braces. This is the syntax for named imports in React.
Output:
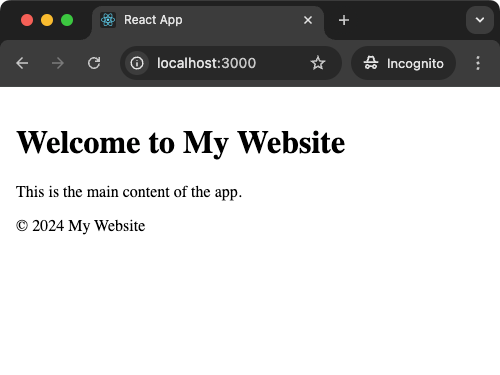
Example 2: Exporting Components Using Default and Named Exports
Here, we demonstrate exporting one component as the default export and another as a named export.
Filename: components.js
import React from 'react';
const Header = () => {
return <header><h1>Welcome to My Website</h1></header>;
};
export const Footer = () => {
return <footer><p>© 2024 My Website</p></footer>;
};
export default Header;
Explanation: In this file, we use export default
for the Header
component, which allows it to be imported without curly braces. The Footer
component is exported as a named export.
Filename: App.js
import React from 'react';
import Header, { Footer } from './components';
const App = () => {
return (
<div>
<Header />
<p>This is the main content of the app.</p>
<Footer />
</div>
);
};
export default App;
Explanation: In this file, we import the Header
component as the default import (no curly braces) and the Footer
component as a named import (with curly braces). This is useful when combining default and named exports in the same file.
Output:
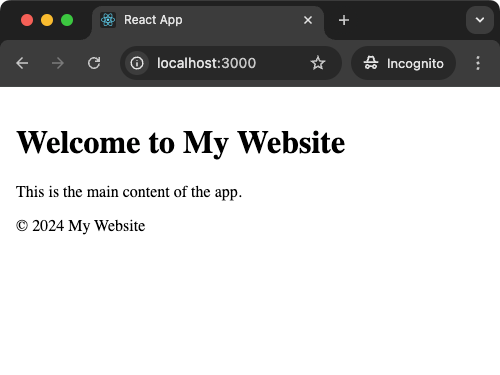
Conclusion
In this tutorial, you learned how to export multiple components in React using named exports, default exports, or a combination of both. These methods enable developers to structure their code better and make components reusable across the application. For larger projects, maintaining organized and reusable components is crucial for scalability and collaboration.