React – How to Get Base URL
In React applications, the base URL represents the root path of your application, which is especially useful when dealing with API requests, routing, or generating dynamic links. The base URL can be obtained and managed effectively using built-in JavaScript methods, environment variables, or React Router configurations.
This tutorial will explain how to retrieve the base URL in a React application and use it to streamline development. By the end of this guide, you will know how to:
- Use the browser’s
window.location
object to determine the base URL - Set and access a base URL using environment variables
- Retrieve the base URL in the context of React Router
Getting Base URL in React
Using the window.location
Object
The simplest way to get the base URL is by using the window.location
object. This object contains information about the current URL, including the protocol, hostname, and port. You can combine these properties to construct the base URL dynamically. For example, window.location.origin
gives you the base URL, including the protocol and host. This method is effective for applications deployed to a root domain without a subdirectory.
Setting a Base URL with Environment Variables
For a more flexible and configurable approach, use environment variables to define and manage the base URL. Create a variable such as REACT_APP_BASE_URL
in your .env
file. This method is especially useful for handling different environments like development, staging, and production. Access the base URL in your React components using process.env.REACT_APP_BASE_URL
.
Configuring the Base URL in React Router
If you are using React Router, the base URL can be managed using the basename
property in the BrowserRouter
or HashRouter
. This allows you to set a custom base path for your routes. It is particularly useful when deploying your application to a subdirectory on a domain. For example, you can configure React Router to use /myapp
as the base path for all routes.
Examples
Example 1: Using window.location
Object
This example demonstrates how to dynamically retrieve the base URL using the window.location
object.
Filename: App.js
import React from 'react';
const App = () => {
const baseURL = window.location.origin; // Gets the base URL (e.g., https://example.com)
return (
<div>
<h1>Base URL</h1>
<p>The base URL of this application is: {baseURL}</p>
</div>
);
};
export default App;
Explanation: The window.location.origin
property dynamically retrieves the protocol, hostname, and port of the current URL. This method works well for most applications deployed without subdirectory structures.
Output
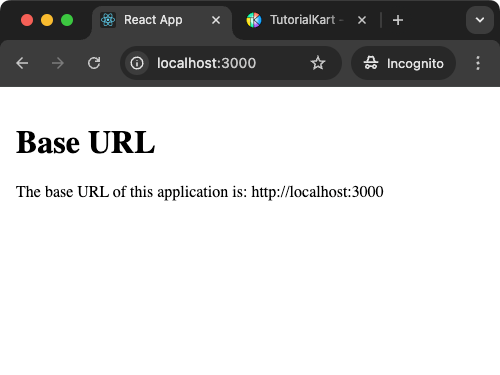
Example 2: Using Environment Variables
In this example, we define the base URL in an environment variable and access it within the React component.
Filename: .env
REACT_APP_BASE_URL=https://www.example.com
Filename: App.js
import React from 'react';
const App = () => {
const baseURL = process.env.REACT_APP_BASE_URL;
return (
<div>
<h1>Base URL</h1>
<p>The base URL of this application is: {baseURL}</p>
</div>
);
};
export default App;
Explanation: The environment variable REACT_APP_BASE_URL
is defined in the .env
file. In React, all environment variables must be prefixed with REACT_APP_
. This approach allows you to manage different base URLs for development and production environments.
Example 3: Using React Router’s basename
In this example, we configure the base URL using React Router’s basename
property.
Filename: App.js
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import Home from './Home';
const App = () => {
return (
<Router basename="/myapp">
<Routes>
<Route path="/" element=<Home /> />
</Routes>
</Router>
);
};
export default App;
Filename: Home.js
import React from 'react';
const Home = () => {
return (
<div>
<h1>Home Page</h1>
<p>Welcome to the base route of the application.</p>
</div>
);
};
export default Home;
Explanation: The basename
property of BrowserRouter
sets the base URL for routing. In this case, all routes will be prefixed with /myapp
, which is useful when deploying your app to a subdirectory.
Conclusion
In this tutorial, you learned how to get the base URL in a React application using the window.location
object, environment variables, and React Router’s basename
property. Choose the method that best fits your application’s requirements.