React – How to Get Cookies
Cookies are small pieces of data stored on the client side that can be used to maintain user sessions, preferences, or other data required for a seamless user experience. In React applications, you may need to retrieve cookies to authenticate users, personalise content, or manage state across sessions.
In this tutorial, we will explore different ways to get cookies in React, including using JavaScript’s built-in document.cookie
property and external libraries like js-cookie
. By the end of this tutorial, you will be able to:
- Read cookies using JavaScript
- Use the
js-cookie
library for managing cookies - Handle edge cases such as decoding cookie values
Steps to Get Cookies in React
Step 1: Understand Cookies
Cookies are key-value pairs stored in the browser. They can be accessed using JavaScript, but handling them manually may require additional parsing.
Step 2: Read Cookies withdocument.cookie
Use JavaScript’s built-in document.cookie
to retrieve cookies. Note that this method returns all cookies as a single string.
Install and use a library like js-cookie
to make cookie handling easier and more reliable.
Examples
Example 1: Getting Cookies with document.cookie
In this example, we use the document.cookie
property to retrieve cookies and parse them manually.
Filename: App.js
import React, { useEffect, useState } from 'react';
const App = () => {
const [cookies, setCookies] = useState({});
useEffect(() => {
const parseCookies = () => {
const cookieString = document.cookie;
const cookies = {};
cookieString.split('; ').forEach(cookie => {
const [name, value] = cookie.split('=');
cookies[name] = decodeURIComponent(value);
});
cookies['sample'] = "dummy value"; // just to display some cookies in the UI, otherwise this line is not necessary
return cookies;
};
setCookies(parseCookies());
}, []);
return (
<div>
<h1>Cookies</h1>
<pre>{JSON.stringify(cookies, null, 2)}</pre>
</div>
);
};
export default App;
Explanation: The document.cookie
property retrieves all cookies as a single string. The code splits the string into individual cookies, parses each one, and decodes their values using decodeURIComponent
. The parsed cookies are then displayed in a JSON format.
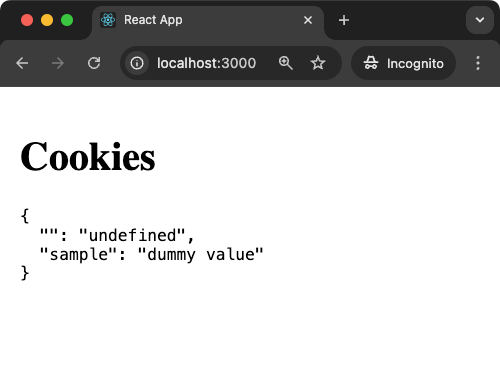
Example 2: Using js-cookie
to Simplify Cookie Handling
The js-cookie
library provides a simple API for working with cookies, including reading, setting, and removing cookies.
Install the library:
Run the following npm command to install js-cookie library.
npm install js-cookie
Filename: App.js
import React, { useEffect, useState } from 'react';
import Cookies from 'js-cookie';
const App = () => {
const [cookieValue, setCookieValue] = useState('');
useEffect(() => {
// we are setting this cookie for demo purpose
Cookies.set('myCookie', 'test-cookie-value', { expires: 7 }); // Expires in 7 days
const value = Cookies.get('myCookie');
setCookieValue(value || 'Cookie not found');
}, []);
return (
<div>
<h1>Cookie Value</h1>
<p>{cookieValue}</p>
</div>
);
};
export default App;
Explanation: The js-cookie
library provides a get
method to easily retrieve cookies by name. In this example, the value of a cookie named myCookie
is retrieved and displayed. If the cookie does not exist, a default message is shown.
Output
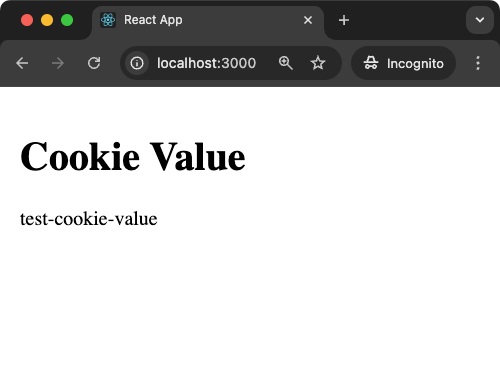
If the cookie is not found, you would get the following output.
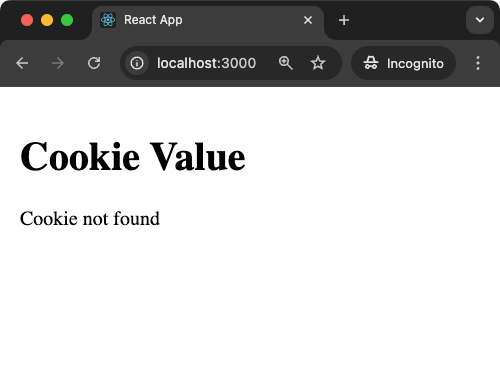
Conclusion
In this tutorial, we covered how to get cookies in React using two approaches: manually parsing cookies with document.cookie
and using the js-cookie
library for convenience. While the manual method provides full control, the js-cookie
library simplifies cookie management and is recommended for most use cases.