React – How to Get Form Data
Forms are essential in any web application for collecting user input. In React, managing and retrieving form data is a common task. React provides various approaches to handle form data, including controlled components, uncontrolled components, and working directly with the DOM.
This tutorial explains how to get form data in React, with practical examples and best practices. By the end, you will understand how to:
- Retrieve form data using controlled components
- Work with uncontrolled components and refs
- Handle form submission and extract all data dynamically
Different Methods to Get Form Data in React
Method 1: Using Controlled Components
In controlled components, form elements are controlled by React state. The value of each input field is stored in the component’s state, and updates are handled by event handlers.
Method 2: Using Uncontrolled Components and Refs
Uncontrolled components rely on the DOM to manage form state. React refs are used to directly access the values of form elements. This approach is simpler for basic forms but provides less control than controlled components.
Method 3: Dynamic Approach
You can also extract and process the form data dynamically during submission without manually managing each input.
Examples
Example 1: Get Form Data Using Controlled Components
This example demonstrates how to get form data using controlled components. We take a form with two input fields to read name and email of the user, and a submit button.
Filename: App.js
import React, { useState } from 'react';
const App = () => {
const [formData, setFormData] = useState({
name: '',
email: '',
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData({
...formData,
[name]: value,
});
};
const handleSubmit = (e) => {
e.preventDefault();
console.log('Form Data:', formData);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
</div>
<div>
<label>Email:</label>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
</div>
<button type="submit">Submit</button>
</form>
);
};
export default App;
Explanation:
- The form fields are controlled by the
formData
state. TheformData
state contains two properties:name
,email
. - Whenever input value is updated
onChange
handler, which ishandleChange
function, is called, and theformData
state is updated. - When user submits the form,
onSubmit={handleSubmit}
makeshandleSubmit
function execute. In this code,handleSubmit
function just logs the form data to the console. But, it is upto you what you would like to do with this form data.
Output
Example 2: Using Uncontrolled Components and Refs
This example shows how to retrieve form data using uncontrolled components and refs.
Filename: App.js
import React, { useRef } from 'react';
const App = () => {
const nameRef = useRef();
const emailRef = useRef();
const handleSubmit = (e) => {
e.preventDefault();
const formData = {
name: nameRef.current.value,
email: emailRef.current.value,
};
console.log('Form Data:', formData);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input type="text" ref={nameRef} />
</div>
<div>
<label>Email:</label>
<input type="email" ref={emailRef} />
</div>
<button type="submit">Submit</button>
</form>
);
};
export default App;
Explanation: Refs are used to directly access the input fields’ values. This approach is simple and avoids managing state but is less flexible than controlled components for dynamic forms.
Output
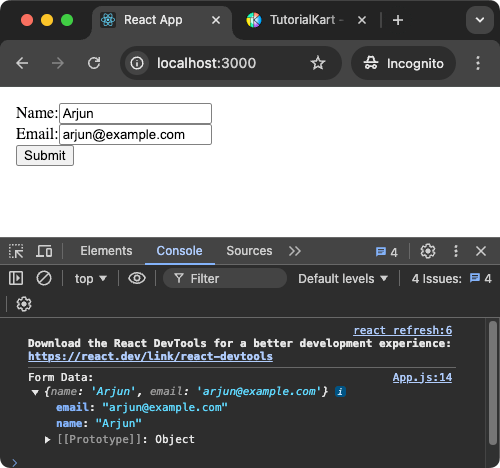
Example 3: Handling All Form Data on Submission
In this example, we extract all form data dynamically during submission without manually managing each input.
Filename: App.js
import React from 'react';
const App = () => {
const handleSubmit = (e) => {
e.preventDefault();
const formData = new FormData(e.target);
const data = Object.fromEntries(formData.entries());
console.log('Form Data:', data);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input type="text" name="name" />
</div>
<div>
<label>Email:</label>
<input type="email" name="email" />
</div>
<button type="submit">Submit</button>
</form>
);
};
export default App;
Explanation: The FormData
object collects all form field values during submission. The Object.fromEntries
method converts the form data into a key-value object, which is logged to the console.
Output
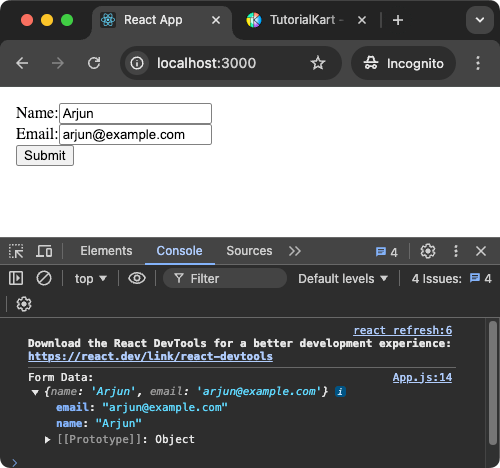
Conclusion
In this tutorial, you learned how to get form data in React using controlled components, uncontrolled components, and form submission handlers. Controlled components provide better control and flexibility, while refs simplify handling basic forms. Choose the method that best suits your application’s requirements.