React – How to Get URL Parameters
When developing React applications, there are situations where you need to access the parameters embedded in the URL. URL parameters are often used to pass data between routes or to customise the content displayed on a page. React provides tools to extract these parameters efficiently, particularly through libraries like react-router-dom
.
This tutorial explains how to get URL parameters in React using useParams
from react-router-dom
, including step-by-step instructions and practical examples.
By the end of this tutorial, you will learn:
- How to set up React Router in your application
- How to access dynamic URL parameters using
useParams
- How to handle query parameters using JavaScript’s URL API
Steps to Get URL Parameters in React
Step 1: Install React Router
Ensure that the react-router-dom
package is installed in your project.
npm install react-router-dom
Step 2: Define Routes with Parameters
Use <Route>
components in your application to define paths that include dynamic segments (e.g., :id
).
Step 3: Access Parameters with useParams
Extract the parameters from the URL in the relevant component using the useParams
hook.
Step 4: Handle Query Parameters (Optional)
For query parameters (e.g., ?key=value
), use the URLSearchParams
API.
Examples
Example 1: Accessing Dynamic URL Parameters with useParams
In this example, we create a route that displays a user’s details based on their ID, which is passed as a dynamic URL parameter.
Filename: App.js
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import UserDetails from './UserDetails';
const App = () => {
return (
<Router>
<Routes>
<Route path="/user/:id" element=<UserDetails /> />
</Routes>
</Router>
);
};
export default App;
Filename: UserDetails.js
import React from 'react';
import { useParams } from 'react-router-dom';
const UserDetails = () => {
const { id } = useParams();
return (
<div>
<h1>User Details</h1>
<p>User ID: {id}</p>
</div>
);
};
export default UserDetails;
Explanation: In this example, the useParams
hook is used to extract the id
parameter from the URL. When the user navigates to a URL like /user/123
, the id
variable will hold the value 123
, which is displayed on the page.
Output:
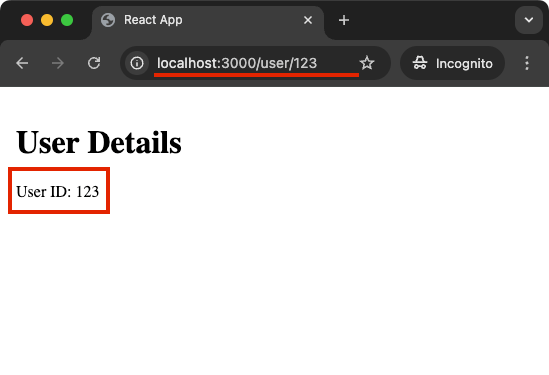
Example 2: Handling Query Parameters
Here, we demonstrate how to extract query parameters (e.g., ?name=John
) using the URLSearchParams
API.
Filename: App.js
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import SearchPage from './SearchPage';
const App = () => {
return (
<Router>
<Routes>
<Route path="/search" element=<SearchPage /> />
</Routes>
</Router>
);
};
export default App;
Filename: SearchPage.js
import React from 'react';
import { useLocation } from 'react-router-dom';
const SearchPage = () => {
const location = useLocation();
const queryParams = new URLSearchParams(location.search);
const name = queryParams.get('name');
return (
<div>
<h1>Search Page</h1>
{name ? <p>Searching for: {name}</p> : <p>No search term provided.</p>}
</div>
);
};
export default SearchPage;
Explanation: The useLocation
hook is used to access the current URL, and URLSearchParams
is used to parse the query parameters. If the user navigates to /search?name=Arjun
, the name
variable will hold the value Arjun
.
Output:
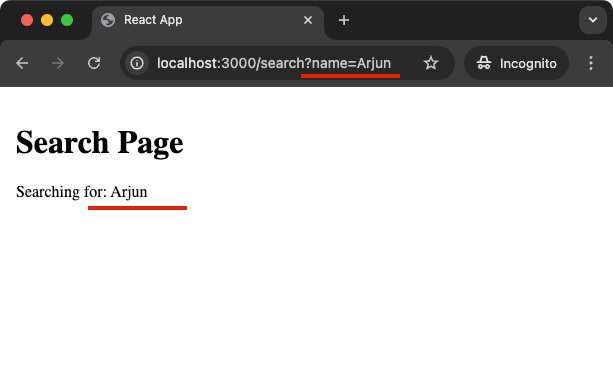
Conclusion
In this tutorial, we explored how to retrieve URL parameters in React using useParams
for dynamic segments and URLSearchParams
for query parameters.