React – Import CSS
In React, styling your components with CSS is a straightforward process. You can import CSS files into your React components to apply styles globally or locally.
In this tutorial, we will see how to import CSS file in your React application using import statement, and we will cover some examples for better understanding.
Steps to Import CSS in React
- Create a CSS file with the desired styles.
- Import the CSS file into your React component using
import
statement. - Apply the styles using the
className
attribute in your JSX.
Example 1: Importing a Global CSS File
Global CSS files define styles that apply to the entire application.
Consider the following css file.
styles.css
</>
Copy
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 10px;
background-color: #f4f4f4;
}
h1 {
color: red;
}
Inside the JavaScript file, we use import statement and provide the path to the CSS file. In this example, styles.css
is present in the same folder as that of App.js,
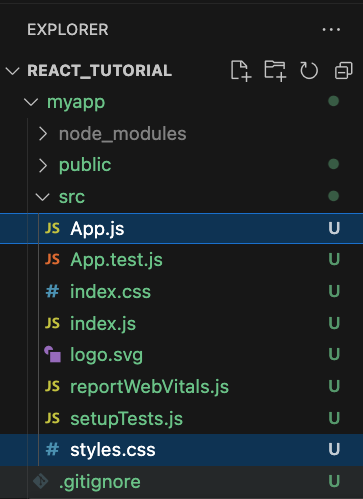
Therefore we used the path './styles.css'
in the import statement.
App.js
</>
Copy
import React from 'react';
import './styles.css';
function App() {
return (
<div>
<h1>Welcome to My React App</h1>
</div>
);
}
export default App;
Output
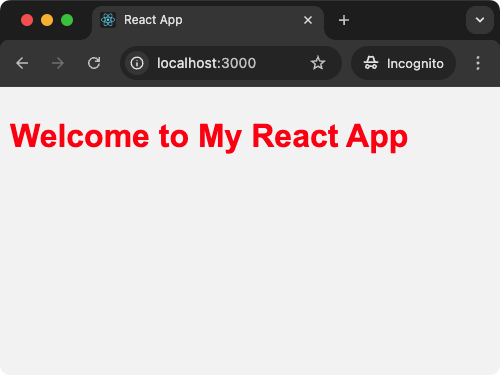