React – How to Lazy Load Images
You can lazy load images to improve application performance by deferring the loading of those images until they are needed.
In React, lazy loading images can significantly enhance the user experience, especially in applications with a lot of media content, by reducing initial load times and bandwidth usage.
This tutorial will guide you through the process of implementing lazy-loaded images in React. By the end of this guide, you will know how to:
- Use native lazy loading with the
loading="lazy"
attribute - Implement lazy loading with the Intersection Observer API
- Leverage libraries like
react-lazy-load-image-component
Methods to Lazy Load Images in React
1: Native Lazy Loading with the loading="lazy"
Attribute
The simplest way to lazy load images in modern browsers is to use the loading="lazy"
attribute on the <img>
element. This is supported natively and requires minimal setup.
2: Implement Lazy Loading with the Intersection Observer API
For more control or support in older browsers, use the Intersection Observer API to detect when an image enters the viewport and then load it. This method requires more setup but provides flexibility for custom behavior.
3: Use a Library for Simplification
Libraries like react-lazy-load-image-component
offer pre-built components and features to make lazy loading images easier to implement and maintain.
Examples
Example 1: Native Lazy Loading
This example demonstrates the use of the loading="lazy"
attribute to implement native lazy loading.
Filename: App.js
import React from 'react';
const App = () => {
return (
<div>
<h1>Native Lazy Loading Example</h1>
<img
src="https://www.tutorialkart.com/img/hummingbird.png"
alt="Placeholder"
loading="lazy"
width="600"
/>
</div>
);
};
export default App;
Explanation: The loading="lazy"
attribute ensures that the image is only loaded when it is about to appear in the viewport. This is the simplest and most efficient way to implement lazy loading in modern browsers.
Output
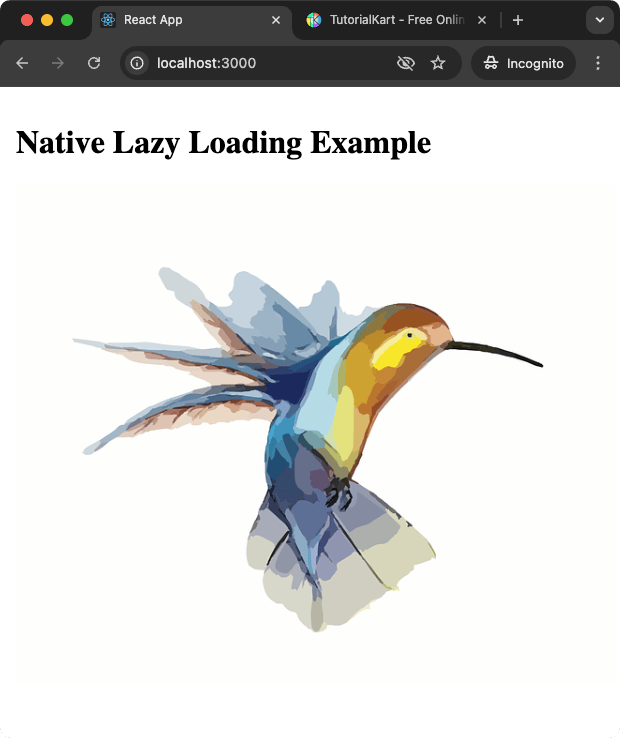
Example 2: Lazy Loading with Intersection Observer API
Here, we use the Intersection Observer API to lazy load images dynamically.
Filename: LazyImage.js
import React, { useEffect, useState } from 'react';
const LazyImage = ({ src, alt }) => {
const [isVisible, setIsVisible] = useState(false);
const imgRef = React.useRef();
useEffect(() => {
const observer = new IntersectionObserver(
([entry]) => {
if (entry.isIntersecting) {
setIsVisible(true);
observer.disconnect();
}
},
{ threshold: 0.1 }
);
if (imgRef.current) {
observer.observe(imgRef.current);
}
return () => {
if (observer && imgRef.current) {
observer.disconnect();
}
};
}, []);
return (
<img
ref={imgRef}
src={isVisible ? src : ''}
alt={alt}
style={{ width: '600px', height: '400px', backgroundColor: '#ddd' }}
/>
);
};
export default LazyImage;
Filename: App.js
import React from 'react';
import LazyImage from './LazyImage';
const App = () => {
return (
<div>
<h1>Intersection Observer Lazy Loading</h1>
<LazyImage src="https://www.tutorialkart.com/img/hummingbird.png" alt="Humming Bird" />
</div>
);
};
export default App;
Explanation: The LazyImage
component uses the Intersection Observer API to detect when the image is in the viewport and then sets the image source dynamically. This provides more control and works well for complex scenarios.
Output
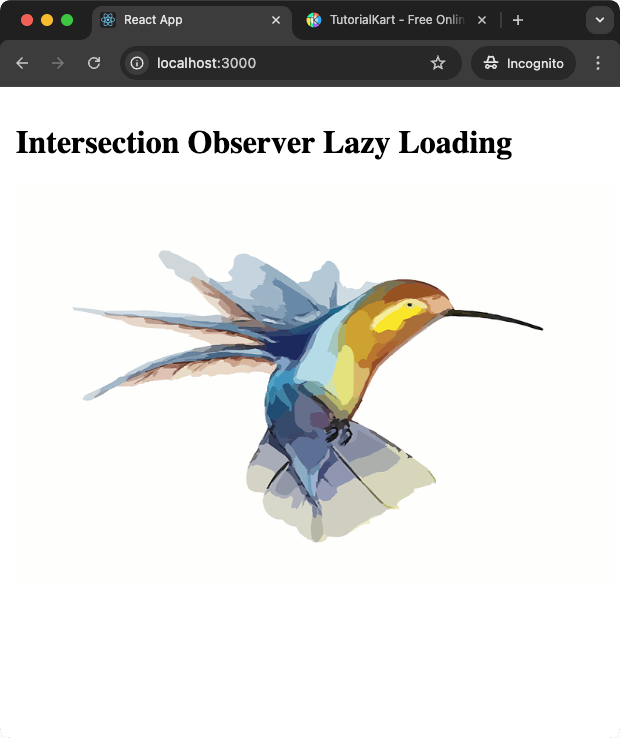
Example 3: Using a Library
In this example, we use the react-lazy-load-image-component
library to simplify lazy loading.
Install the library:
npm install react-lazy-load-image-component
Filename: App.js
import React from 'react';
import { LazyLoadImage } from 'react-lazy-load-image-component';
import 'react-lazy-load-image-component/src/effects/blur.css';
const App = () => {
return (
<div>
<h1>Lazy Loading with Library</h1>
<LazyLoadImage
src="https://www.tutorialkart.com/img/hummingbird.png"
alt="Placeholder"
effect="blur"
width="600"
/>
</div>
);
};
export default App;
Explanation: The LazyLoadImage
component from the library provides built-in features like placeholder effects and smooth transitions, making it easier to implement lazy loading with minimal code.
Output
Conclusion
In this tutorial, you learned how to lazy load images in React using native browser features, the Intersection Observer API, and a third-party library. Each approach has its benefits, and you can choose the method that best suits your application’s requirements. Lazy loading images improves performance and ensures a better user experience, especially in media-heavy applications.