React – How to Log to Console
You can log messages to the console as part of debugging and monitoring in React applications. Whether you’re troubleshooting a problem or verifying application behaviour, using console.log
effectively can make your work easier and more efficient.
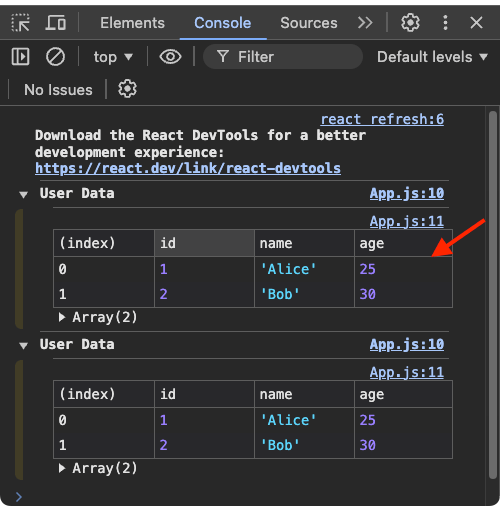
In this tutorial, we will guide you through different scenarios and best practices for logging messages to the console in React. By the end of this guide, you will understand how to:
- Use
console.log
in functional components - Log data during lifecycle events
- Format and filter console messages
Approaches for Logging to Console in React
1. Logging in Functional Components
In functional components, you can use console.log
directly within the component body or within event handlers to log data. This approach is helpful for debugging variable values, state changes, and function outputs during the component’s rendering cycle or user interactions.
2. Logging During Lifecycle Events
To log messages during specific lifecycle events, you can use hooks like useEffect
. This is particularly useful for monitoring component mount, update, or unmount behaviors. Place the console.log
statements inside the useEffect
callback to capture relevant events.
3. Formatting and Filtering Console Messages
You can enhance the clarity of your logs by formatting them using template literals, string interpolation, or console methods like console.table
and console.group
. Use browser developer tools to filter messages by type (e.g., log, warn, error) for better debugging focus.
Examples
Example 1: Basic Logging in a Functional Component
This example demonstrates how to log a message and variable values in a functional component.
Filename: App.js
import React, { useState } from 'react';
const App = () => {
const [count, setCount] = useState(0);
const handleClick = () => {
console.log('Button clicked!');
console.log(`Current count: ${count}`);
setCount(count + 1);
};
return (
<div>
<h1>Console Logging Example</h1>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
};
export default App;
Explanation: The handleClick
function logs a message and the current count whenever the button is clicked. This provides insight into the state value before the update.
Output
Example 2: Logging in Lifecycle Events Using useEffect
In this example, we log messages during the component’s mount and update events using the useEffect
hook.
Filename: App.js
import React, { useState, useEffect } from 'react';
const App = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Component mounted or updated');
console.log(`Count value: ${count}`);
return () => {
console.log('Component will unmount');
};
}, [count]);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<h1>useEffect Logging Example</h1>
<p>Count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
};
export default App;
Explanation: The useEffect
hook logs messages whenever the component mounts or the count
value updates. The cleanup function logs a message when the component unmounts, helping to track the component’s lifecycle.
Output
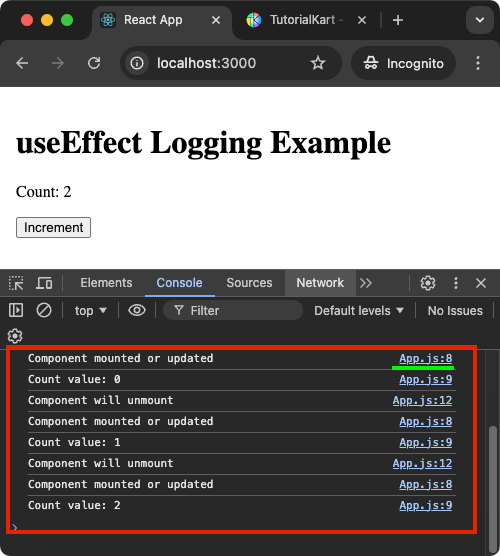
Example 3: Enhanced Logging with Formatting
This example demonstrates how to format logs for improved readability using console.table
and console.group
.
Filename: App.js
import React from 'react';
const App = () => {
const data = [
{ id: 1, name: 'Alice', age: 25 },
{ id: 2, name: 'Bob', age: 30 },
];
console.group('User Data');
console.table(data);
console.groupEnd();
return (
<div>
<h1>Formatted Logging Example</h1>
<p>Check the console for formatted logs.</p>
</div>
);
};
export default App;
Explanation: The console.table
method displays array data in a tabular format, while console.group
groups related logs together. This approach improves log organisation and readability.
Output
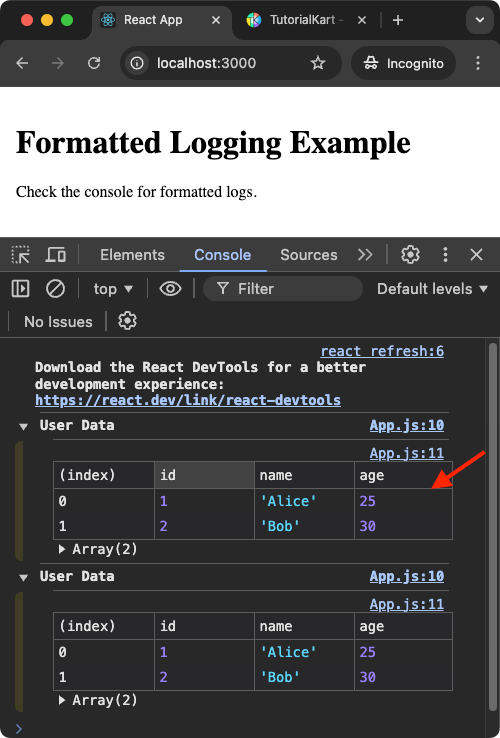
Conclusion
In this tutorial, you learned how to log messages to the console in React. By understanding how to log in functional components, during lifecycle events, and with enhanced formatting, you can improve your debugging and monitoring practices.