React – How to Read JSON File
Reading JSON files in React is a common task when dealing with external data sources, configuration files, or static data. React provides various methods to import and use JSON files in your components.
In this tutorial, we will guide you through the process of reading a JSON file in a React application and using the data in a component. We will cover direct imports and fetching JSON dynamically.
Steps to Read a JSON File in React
Static JSON File
First, create or obtain the JSON file that you want to read. JSON (JavaScript Object Notation) is a lightweight data-interchange format. Save the file in your React project directory. For example, you can save it in a folder named data
with the name example.json
.
If the JSON file is static and will not change during runtime, you can import it directly into your React component. To do this, use an import
statement at the top of your file. Once imported, the JSON data is available as a JavaScript object and can be used directly in the component’s state or JSX.
JSON File via API
If the JSON file is dynamic or located on a server, you can fetch it at runtime using the fetch
API or an HTTP client like axios
. Use the useEffect
hook to perform the fetch operation when the component mounts. Parse the response to extract the JSON data and update the component’s state with the fetched data.
Examples
Example 1: Importing a Static JSON File
Here, we demonstrate how to directly import and use a JSON file in a React component.
Please note that the data/exmaple.json file is present in the src folder.
Files Structure
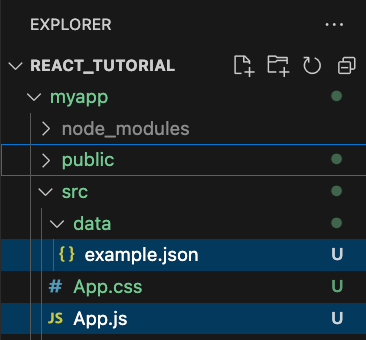
Filename: example.json
{
"name": "Arjun",
"age": 30,
"profession": "Developer"
}
Filename: App.js
import React from 'react';
import jsonData from './data/example.json';
const App = () => {
return (
<div>
<h1>User Information</h1>
<p>Name: {jsonData.name}</p>
<p>Age: {jsonData.age}</p>
<p>Profession: {jsonData.profession}</p>
</div>
);
};
export default App;
Explanation: The JSON file is imported as jsonData
and used directly in the component’s JSX. This method is suitable for static data that doesn’t change at runtime.
Output
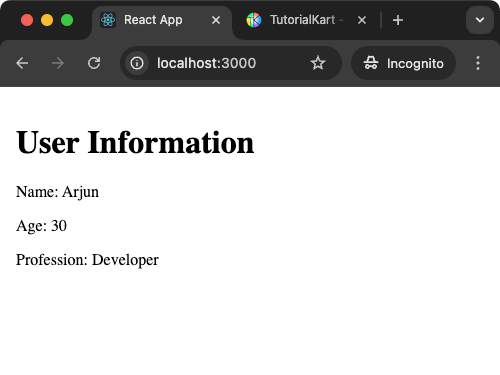
Example 2: Fetching a JSON File Dynamically
In this example, we fetch a JSON file dynamically using the fetch
API.
Please note that the data/exmaple.json file is present in the public folder.
Files Structure
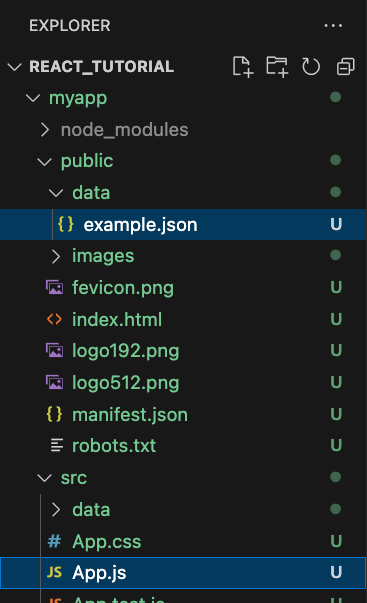
Filename: App.js
import React, { useEffect, useState } from 'react';
const App = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('/data/example.json')
.then((response) => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then((jsonData) => setData(jsonData))
.catch((error) => console.error('Error fetching JSON:', error));
}, []);
return (
<div>
<h1>User Information</h1>
{data ? (
<div>
<p>Name: {data.name}</p>
<p>Age: {data.age}</p>
<p>Profession: {data.profession}</p>
</div>
) : (
<p>Loading...</p>
)}
</div>
);
};
export default App;
Explanation: The useEffect
hook is used to fetch the JSON file when the component mounts. The fetched data is parsed and stored in the data
state variable. The component renders the data once it’s available.
Output
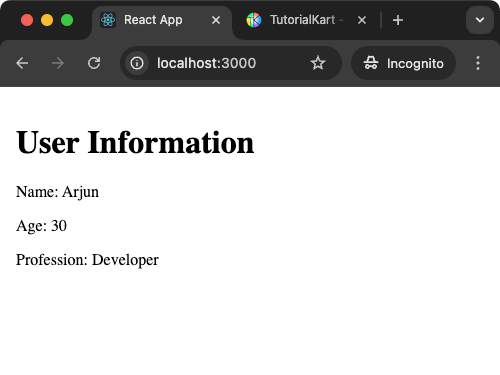
Conclusion
In this tutorial, you learned how to read JSON files in React. For static JSON files, direct imports are the simplest and most efficient approach. For dynamic or server-hosted JSON files, fetching the data at runtime using fetch
or axios
is more appropriate. Both methods allow you to effectively use JSON data in your React applications based on the specific requirements of your project.