React Keys
In React, keys
are used to uniquely identify elements in a list. Keys help React efficiently update and render lists by identifying which items have changed, added, or removed. Without keys, React would have to re-render the entire list every time there is a change, which can impact performance.
1 Why Keys Are Important
Keys are essential for lists where items can be dynamically added, removed, or updated. React uses keys to identify which list items correspond to each rendered element. This makes updates faster and ensures consistent behaviour.
2 Example: Using Array Index as Keys
In this example, we use the array index as a key to render a list. This approach works for simple lists where the items do not change dynamically.
App.js
import React from 'react';
function SimpleList() {
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
export default SimpleList;
Explanation:
key={index}
: Uses the array index as a key.- This approach is sufficient for static lists but may cause issues if the list items are reordered or dynamically updated.
Output:
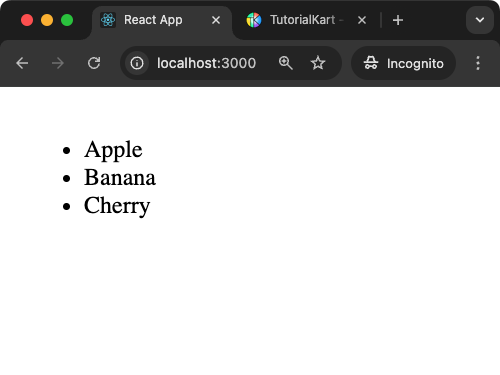
3 Example: Using Unique IDs as Keys
Here, we use unique IDs from an object array as keys. This is the recommended approach for lists where items can change dynamically.
App.js
import React from 'react';
function UniqueKeysList() {
const items = [
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Cherry' }
];
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
export default UniqueKeysList;
Explanation:
key={item.id}
: Uses the uniqueid
property of each item as the key.- This approach ensures React can efficiently manage updates, even if the order of items changes or items are added/removed.
Output:
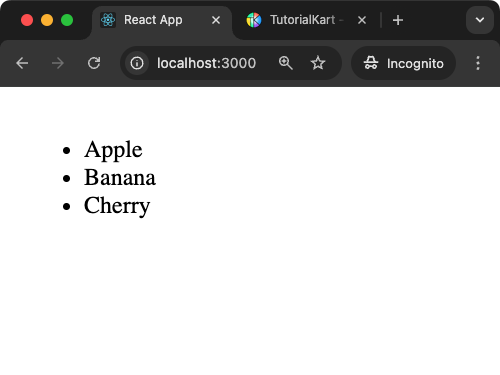
4 Example: Keys for Nested Lists
In this example, we render a nested list, where both the parent and child elements have unique keys. This demonstrates how to handle hierarchical data structures.
App.js
import React from 'react';
function NestedList() {
const categories = [
{ id: 1, name: 'Fruits', items: ['Apple', 'Banana', 'Cherry'] },
{ id: 2, name: 'Vegetables', items: ['Carrot', 'Potato', 'Spinach'] }
];
return (
<div>
{categories.map(category => (
<div key={category.id}>
<h3>{category.name}</h3>
<ul>
{category.items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
))}
</div>
);
}
export default NestedList;
Explanation:
key={category.id}
: Uniquely identifies each category in the parent list.key={index}
: Identifies each item within a category. For nested lists, ensure both levels have unique keys.
Output:
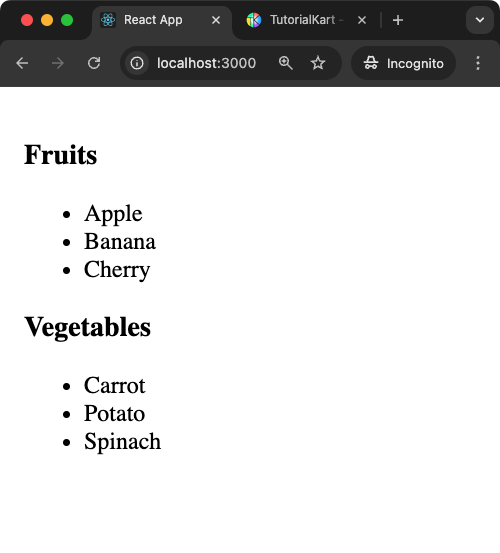
5 Common Mistakes with Keys
Using keys incorrectly can lead to unexpected behavior in React. Here are some common mistakes:
- Using non-unique keys, such as the array index, in a dynamic list where items can change order.
- Not providing a key at all, which will cause React to issue a warning in the console.
Conclusion
Keys are essential for efficient and predictable list rendering in React. Always ensure keys are unique and stable to avoid rendering issues.