React Lists
In React, you can render lists of data dynamically. Lists are a common feature in React applications, such as displaying user data, product catalogs, or items in a to-do list. React uses JavaScript’s map()
method to render lists efficiently, and each list item should have a unique key
prop to ensure proper performance and rendering.
1 Rendering a Simple List
In this example, we will render a list of fruits dynamically using the map()
function. Each item in the list will be displayed as an HTML <li>
element.
App.js
import React from 'react';
function FruitList() {
const fruits = ['Apple', 'Banana', 'Cherry', 'Date', 'Elderberry'];
return (
<ul>
{fruits.map((fruit, index) => (
<li key={index}>{fruit}</li>
))}
</ul>
);
}
export default FruitList;
Explanation:
fruits
: An array containing the list of fruit names.map()
: Iterates over thefruits
array and creates an<li>
element for each fruit.key
: Provides a unique identifier for each list item. In this case, theindex
is used as the key.
Output:
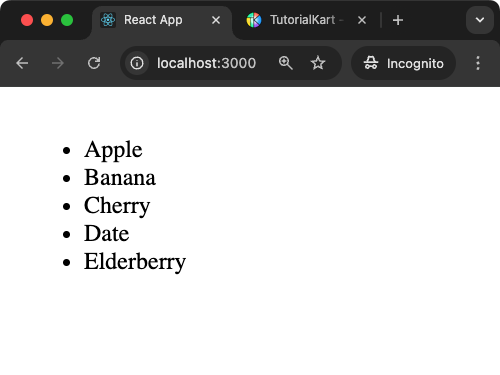
2 Rendering a List of Objects
This example demonstrates how to render a list of objects. Each object contains additional details, such as the fruit’s name and color.
App.js
import React from 'react';
function FruitDetails() {
const fruits = [
{ id: 1, name: 'Apple', color: 'Red' },
{ id: 2, name: 'Banana', color: 'Yellow' },
{ id: 3, name: 'Cherry', color: 'Red' },
{ id: 4, name: 'Date', color: 'Brown' },
{ id: 5, name: 'Elderberry', color: 'Purple' }
];
return (
<ul>
{fruits.map((fruit) => (
<li key={fruit.id}>
{fruit.name} is {fruit.color}
</li>
))}
</ul>
);
}
export default FruitDetails;
Explanation:
fruits
: An array of objects where each object containsid
,name
, andcolor
properties.map()
: Iterates over the array to create a detailed description of each fruit.key
: Uses the uniqueid
property of each object for React’s internal optimization.
Output:
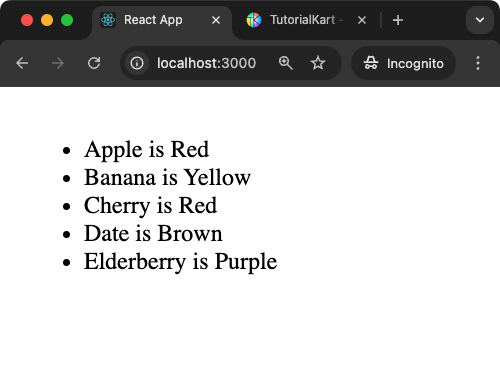
3 Rendering a Nested List
In this example, we render a nested list where each item can contain a sublist. This approach is useful for hierarchical data like categories or folders.
App.js
import React from 'react';
function CategoryList() {
const categories = [
{ name: 'Fruits', items: ['Apple', 'Banana', 'Cherry'] },
{ name: 'Vegetables', items: ['Carrot', 'Potato', 'Spinach'] },
{ name: 'Grains', items: ['Rice', 'Wheat', 'Oats'] }
];
return (
<div>
{categories.map((category, index) => (
<div key={index}>
<h3>{category.name}</h3>
<ul>
{category.items.map((item, subIndex) => (
<li key={subIndex}>{item}</li>
))}
</ul>
</div>
))}
</div>
);
}
export default CategoryList;
Explanation:
categories
: An array of objects where each object has a name and a sublist of items.- Nested
map()
: Handles rendering both the category name and its items in a nested structure. - Separate
key
: Ensures unique keys for both parent and child elements.
Output:
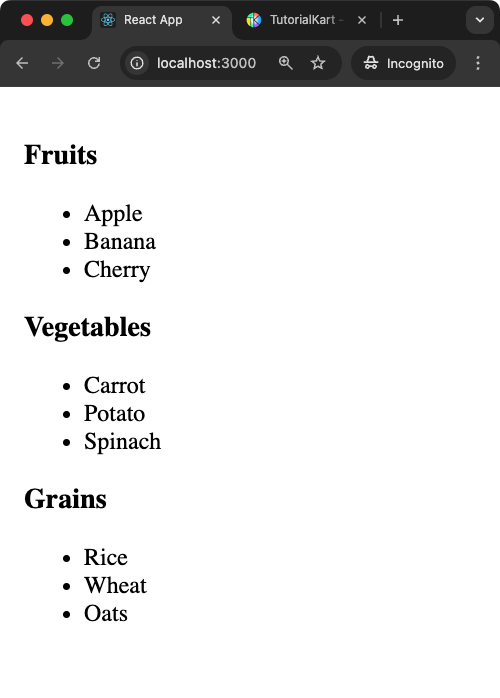
4 Conditional Rendering in Lists
Here, we render a list with conditional logic to style items differently or hide specific items. This is useful when dealing with dynamic or filtered data.
App.js
import React from 'react';
function ConditionalList() {
const fruits = [
{ id: 1, name: 'Apple', isFavorite: true },
{ id: 2, name: 'Banana', isFavorite: false },
{ id: 3, name: 'Cherry', isFavorite: true },
{ id: 4, name: 'Date', isFavorite: false }
];
return (
<ul>
{fruits.map((fruit) => (
fruit.isFavorite ? (
<li key={fruit.id} style={{ color: 'green' }}>
{fruit.name} (Favorite)
</li>
) : (
<li key={fruit.id}>{fruit.name}</li>
)
))}
</ul>
);
}
export default ConditionalList;
Explanation:
isFavorite
: A boolean property in the objects determines whether the fruit is a favorite.- Conditional Logic: Styles favorite fruits differently by changing the text color to green.
- This approach allows dynamic styling and content filtering within a list.
Output:
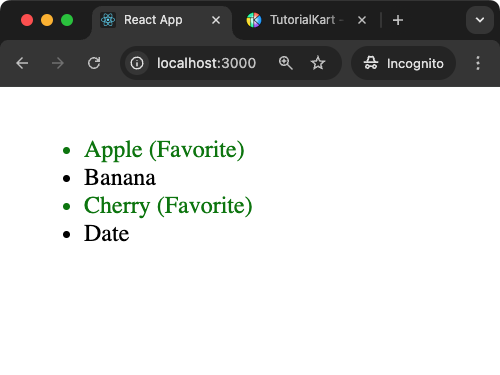
Conclusion
React provides powerful tools for rendering lists dynamically using the map()
function. Whether rendering simple lists, nested data, or applying conditional logic, lists in React are highly flexible and easy to manage. Practice these examples to build efficient and dynamic list-based UIs in your React applications.