React Props
In React, props (short for properties) are a mechanism for passing data from a parent component to a child component. Props are read-only and allow components to be dynamic by providing them with inputs that can change. They are passed to child components as attributes in JSX and can be accessed in the child component using the props
object.
Syntax of Props
Props are passed as attributes in JSX and can be accessed in the child component as follows:
// Parent Component
function Parent() {
return <Child name="Alice" age={25} />;
}
// Child Component
function Child(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
Passing Props: In the parent component, props are passed to the child component as attributes in JSX. For example, name="Alice"
and age={25}
are props passed to Child
.
Accessing Props: In the child component, the props are accessed using the props
object. For instance, props.name
retrieves the value of the name
prop, and props.age
retrieves the value of the age
prop.
Dynamic Rendering: The child component dynamically uses the values of props
to display data, making the component reusable with different inputs.
This approach demonstrates how props enable parent-to-child communication in React components.
1 Passing Basic Props
In this example, we demonstrate how to pass and use basic props. The parent component sends a name and age to the child component.
App.js
import React from 'react';
function Child(props) {
return (
<div>
<p>Hello, {props.name}! You are {props.age} years old.</p>
</div>
);
}
function Parent() {
return <Child name="Arjun" age={25} />;
}
export default Parent;
Explanation:
- The parent component passes
name
andage
as props to the child component. - The child component accesses these props using
props.name
andprops.age
. - Props make the child component reusable with different data.
Output:
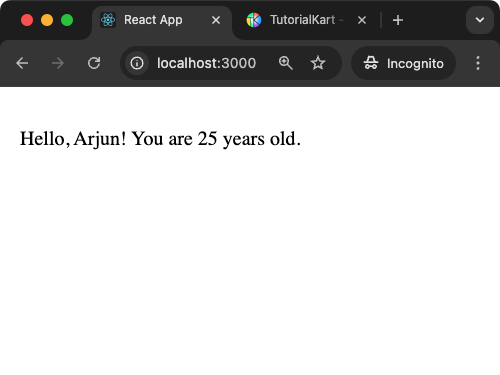
2 Using Destructuring with Props
Destructuring props makes the code cleaner and easier to read.
Example
In this example, we refactor the previous example using destructuring.
App.js
import React from 'react';
function Child({ name, age }) {
return (
<div>
<p>Hello, {name}! You are {age} years old.</p>
</div>
);
}
function Parent() {
return <Child name="Ram" age={30} />;
}
export default Parent;
We have rewritten Child(props)
that we used in the previous example with Child({ name, age })
, which is the destructuring props.
Explanation:
- The
Child
component uses destructuring to extractname
andage
directly from the props object. - This approach improves readability and makes the code cleaner.
Output:
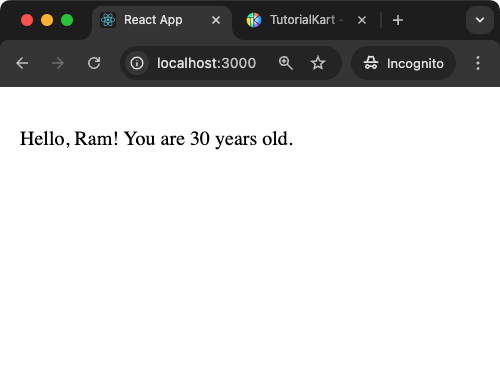
3 Passing Functions as Props
Props can also be used to pass functions from a parent component to a child component. This allows the child component to communicate with the parent.
Example
In this example, a button in the child component triggers a function that is defined in the parent component.
App.js
import React from 'react';
function Child({ onButtonClick }) {
return (
<button onClick={onButtonClick}>Click Me</button>
);
}
function Parent() {
const handleClick = () => {
alert('Button clicked!');
};
return <Child onButtonClick={handleClick} />;
}
export default Parent;
Explanation:
- The parent component defines a function
handleClick
. - This function is passed as a prop to the child component via the
onButtonClick
attribute using<Child onButtonClick={handleClick}
.onButtonClick
is assigned with the passedhandleClick
function. - When the button in the child component is clicked, it triggers the
handleClick
function in the parent component.
Output:
Conclusion
Props are a powerful way to make React components dynamic and reusable. They allow you to pass data and functions between components, enabling better component interaction and separation of concerns. Mastering props is essential for building scalable React applications.