React Quiz App
A React Quiz App is a great project to learn how to manage state, work with dynamic data, and handle user interactions.
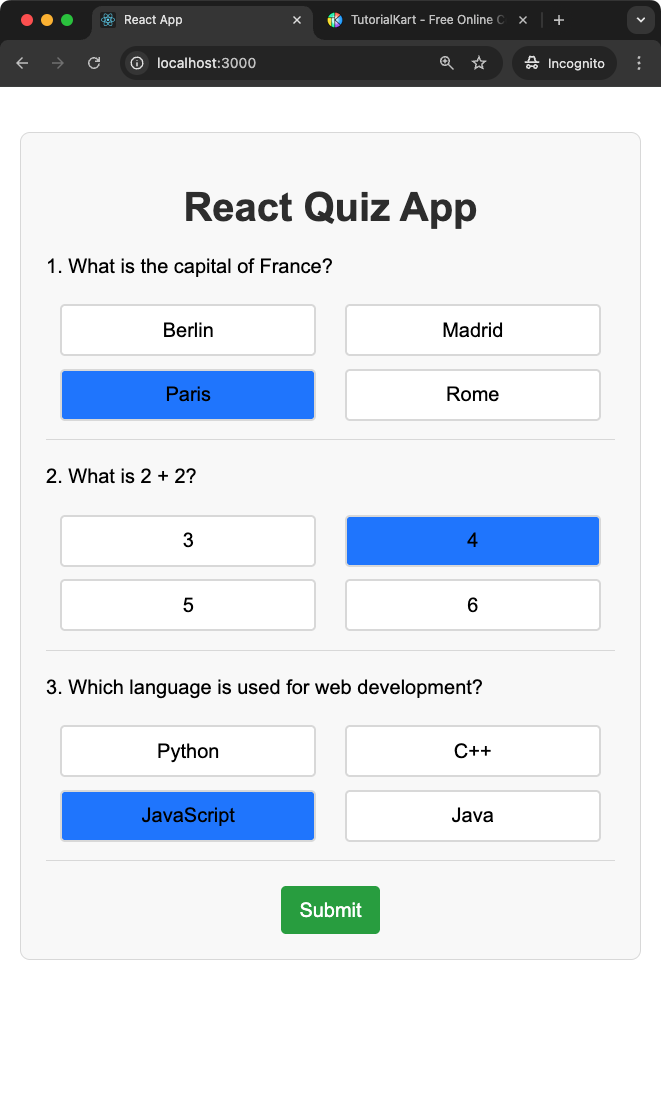
This quiz app features questions with multiple-choice answers, allows users to select their answers, and provides a summary of their score at the end. The app is designed to be interactive, user-friendly, and visually appealing.
Features of the Quiz App
- Displays a list of questions with multiple-choice answers.
- Allows users to select answers for each question.
- Calculates and displays the user’s score at the end of the quiz.
- Includes dynamic styling for correct and incorrect answers.
Steps to Use the App
- Read each question carefully and select the best answer by clicking on one of the options.
- Once all questions are answered, click the “Submit” button to complete the quiz.
- The app will calculate your score and display a summary of correct and incorrect answers.
Complete React Quiz Application
Create an empty React Application, and edit the App.js, and App.css files.
The following is the screenshot of project explorer in Visual Studio Code.
Explorer
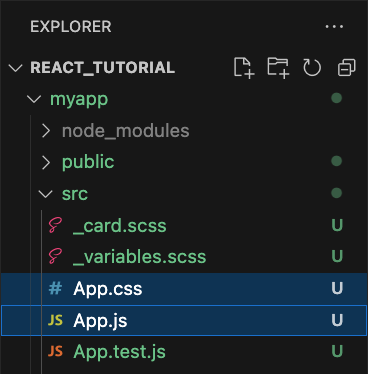
App.js
import React, { useState } from 'react';
import './App.css';
function QuizApp() {
const questions = [
{
question: 'What is the capital of France?',
options: ['Berlin', 'Madrid', 'Paris', 'Rome'],
answer: 'Paris'
},
{
question: 'What is 2 + 2?',
options: ['3', '4', '5', '6'],
answer: '4'
},
{
question: 'Which language is used for web development?',
options: ['Python', 'C++', 'JavaScript', 'Java'],
answer: 'JavaScript'
}
];
const [currentAnswers, setCurrentAnswers] = useState([]);
const [score, setScore] = useState(null);
const [submitted, setSubmitted] = useState(false);
const handleOptionClick = (questionIndex, option) => {
const updatedAnswers = [...currentAnswers];
updatedAnswers[questionIndex] = option;
setCurrentAnswers(updatedAnswers);
};
const handleSubmit = () => {
let calculatedScore = 0;
questions.forEach((q, index) => {
if (currentAnswers[index] === q.answer) {
calculatedScore++;
}
});
setScore(calculatedScore);
setSubmitted(true);
};
const getButtonClass = (option, index, question) => {
let className = "";
if (submitted) {
if (option === question.answer) {
className = 'correct ';
} else if (currentAnswers[index] === option) {
className = 'incorrect ';
}
}
className += (currentAnswers[index] === option) ? 'selected ' : '';
return className;
};
return (
<div className="quiz-app">
<h1>React Quiz App</h1>
{questions.map((q, index) => (
<div key={index} className="question">
<p>{index + 1}. {q.question}</p>
<div className="options">
{q.options.map((option, i) => (
<button
key={i}
className={`option-button ${getButtonClass(option, index, q)}`}
onClick={() => !submitted && handleOptionClick(index, option)}
>
{option}
</button>
))}
</div>
</div>
))}
<button onClick={handleSubmit} className="submit-button">Submit</button>
{score !== null && (
<div className="result">
<p>Your Score: {score} / {questions.length}</p>
</div>
)}
</div>
);
}
export default QuizApp;
App.css
.quiz-app {
font-family: Arial, sans-serif;
margin: 20px auto;
max-width: 600px;
text-align: center;
padding: 20px;
border: 1px solid #ddd;
border-radius: 8px;
background-color: #f9f9f9;
}
h1 {
color: #333;
margin-bottom: 20px;
}
.question {
margin-bottom: 20px;
text-align: left;
padding-bottom: 10px;
border-bottom: 1px solid #ddd;
}
.options {
display: grid;
grid-template-columns: 1fr 1fr;
}
.option-button {
display: block;
margin: 5px auto;
padding: 10px 15px;
border: 2px solid #ddd;
border-radius: 4px;
background-color: #fff;
cursor: pointer;
font-size: 16px;
width: 90%;
}
.option-button:hover {
background-color: #f0f0f0;
}
.option-button.selected {
background-color: #007bff;
}
.option-button.correct {
border-color: #28a745;
}
.option-button.incorrect {
border-color: #dc3545;
}
.submit-button {
padding: 10px 15px;
border: none;
border-radius: 4px;
background-color: #28a745;
color: white;
cursor: pointer;
font-size: 16px;
}
.submit-button:hover {
background-color: #218838;
}
.result {
margin-top: 20px;
font-size: 18px;
color: #333;
}
Conclusion
The React Quiz App is a fun and interactive project to learn about state management and dynamic rendering. You can enhance the app further by adding features like time limits, category filters, or score saving. Try building this app to strengthen your React skills!