React State
What is State in React?
Each component in React has some data in it and is available in the form of an object. state refers to that built-in object in the component and is used to set or modify data within the component. With state, we can keep track of changing information that needs to be displayed by the component, or interacted with by the user. Each component in React can have its own state, and it changes to the state trigger re-renders to update the UI.
Why is State Important in a React Application?
- Dynamic Updates: State allows React components to render dynamic and interactive user interfaces based on the current data in the state.
- Reactivity: When the state changes, React automatically re-renders the component, ensuring that the UI stays in sync with the application logic.
- Isolation: State is scoped to individual components. This makes it easier to manage specific parts of an application.
How to Define State?
In functional components, the useState
hook is used to define state. The useState
hook returns an array with two elements:
- The current state value.
- A function to update the state.
For example, in the following statement, useState()
creates a state with an initial state value of 0
. count
is used to read the state, and setCount
function is used to update the state.
const [count, setCount] = useState(0);
Example: Defining State
In the following React application, we defined a state, and displayed the data in that state to UI using JSX.
App.js
import React, { useState } from 'react';
function Counter() {
// Define state with initial value 0
const [count, setCount] = useState(0);
return (
<div>
<p>Current Count: {count}</p>
</div>
);
}
export default Counter;
Output:
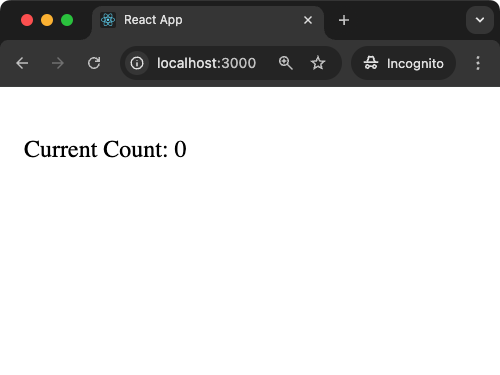
How to Update State?
State updates in React are asynchronous and must be done using the state updater function returned by useState
. React batches these updates to optimise performance.
Example: Updating State
This example adds buttons to increment and decrement a counter:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1); // Increment count by 1
};
const decrement = () => {
setCount(count - 1); // Decrement count by 1
};
return (
<div>
<p>Current Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
You should never directly mutate the state object, meaning, you should not use count to update the state, it should be read only.
Output:
Key Points to Remember
- State is managed locally within a component and updated using the updater function provided by
useState
. - State changes are asynchronous, so avoid directly modifying the state object.
- State updates trigger re-renders to reflect changes in the UI.
Conclusion
State in React is fundamental for building interactive and dynamic user interfaces. By understanding how to define and update state, you can create applications that respond to user input and display changing data in real-time.