React useContext Hook
The useContext
hook allows you to access React’s Context API more easily in functional components. Instead of manually wrapping components with a Consumer
, useContext
lets you consume the context directly, improving code readability and simplicity.
1. Basic Example for useContext Hook: Theme Context
In this example, we create a theme context to manage light and dark modes in an application. The useContext
hook is used to retrieve the current theme.
import React, { createContext, useContext } from 'react';
const ThemeContext = createContext('light');
function ThemeDisplay() {
const theme = useContext(ThemeContext); // Access the current theme
return <p>The current theme is: {theme}</p>;
}
function App() {
return (
<ThemeContext.Provider value="dark">
<ThemeDisplay />
</ThemeContext.Provider>
);
}
export default App;
Explanation:
ThemeContext
: A context created usingcreateContext
with a default value of'light'
.useContext
: Retrieves the current context value (in this case,'dark'
).- The context value is set in the
ThemeContext.Provider
and consumed in theThemeDisplay
component.
Output:
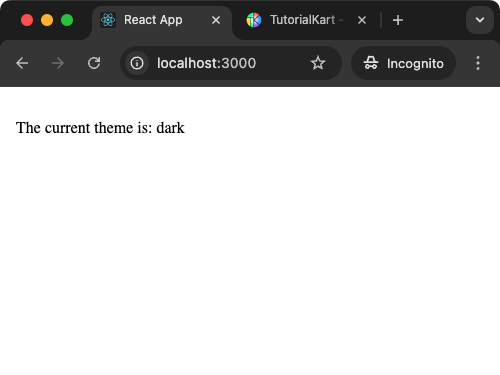
2. Example: User Authentication Context using useContext Hook
This example demonstrates how to use useContext
to manage user authentication. The context provides information about whether a user is logged in.
import React, { createContext, useContext, useState } from 'react';
const AuthContext = createContext();
function AuthDisplay() {
const { isLoggedIn, toggleLogin } = useContext(AuthContext);
return (
<div>
<p>User is {isLoggedIn ? 'logged in' : 'logged out'}.</p>
<button onClick={toggleLogin}>{isLoggedIn ? 'Log Out' : 'Log In'}</button>
</div>
);
}
function App() {
const [isLoggedIn, setIsLoggedIn] = useState(false);
const toggleLogin = () => {
setIsLoggedIn(!isLoggedIn);
};
return (
<AuthContext.Provider value={{ isLoggedIn, toggleLogin }}>
<AuthDisplay />
</AuthContext.Provider>
);
}
export default App;
Explanation:
AuthContext
: Provides authentication state and actions to child components.useContext
: Accesses theisLoggedIn
state andtoggleLogin
function in theAuthDisplay
component.- The
AuthDisplay
component dynamically updates the UI based on the user’s login status.
Output:
3. Example: Language Selector Context using useContext Hook
In this example, a language context is used to manage the application’s current language. The useContext
hook allows components to access and change the language dynamically.
import React, { createContext, useContext, useState } from 'react';
const LanguageContext = createContext();
function LanguageSelector() {
const { language, setLanguage } = useContext(LanguageContext);
return (
<div>
<p>Current Language: {language}</p>
<button onClick={() => setLanguage('English')}>English</button>
<button onClick={() => setLanguage('Spanish')}>Spanish</button>
</div>
);
}
function App() {
const [language, setLanguage] = useState('English');
return (
<LanguageContext.Provider value={{ language, setLanguage }}>
<LanguageSelector />
</LanguageContext.Provider>
);
}
export default App;
Explanation:
LanguageContext
: Provides the current language and a function to update it.useContext
: Accesses thelanguage
value andsetLanguage
function in theLanguageSelector
component.- The UI updates dynamically as the user selects different languages.
Output:
Conclusion
The useContext
hook simplifies the process of consuming context in React applications. It eliminates the need for nested Consumer
components, resulting in cleaner and more readable code. Use useContext
whenever you need to share state or functionality across multiple components without prop drilling.